What is the let function in Kotlin?
The let function in Kotlin is a powerful tool used to execute a given block of code on a non-null object. It provides a concise and expressive way to perform operations on objects while ensuring they are not null, thus preventing NullPointerExceptions.
The let function is an extension function defined on all types, including nullable types. When called on a nullable object, let executes the specified lambda function only if the object is not null. Inside the lambda function, the object becomes accessible through the it keyword.
Here’s a simple example demonstrating the usage of the let function:
kotlin val nullableName: String? = "John" nullableName?.let { name -> println("Name is $name") }
In this example, the let function is invoked on nullableName, a nullable String? type. If nullableName is not null, the lambda function inside let is executed, and the non-null value is accessed through the name parameter.
The let function is particularly useful for performing operations such as data processing, transformation, or validation on non-null objects in a safe and concise manner. It helps improve code readability and maintainability by eliminating the need for explicit null checks and reducing boilerplate code.
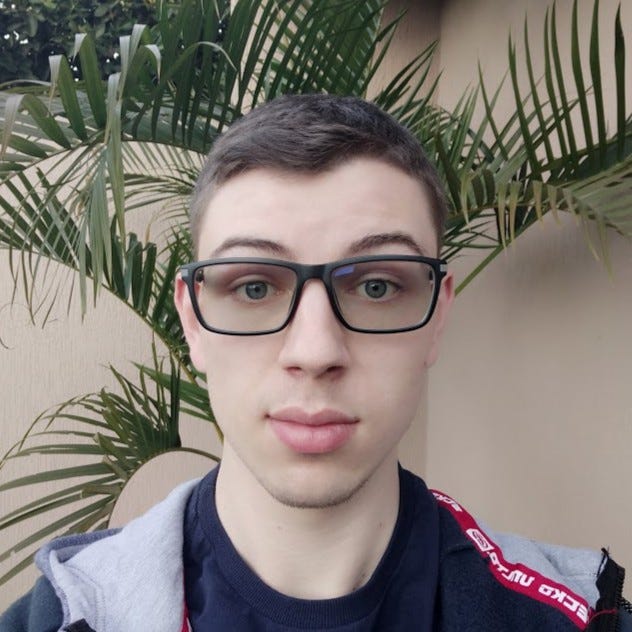
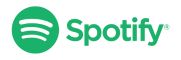