What is the difference between listOf and mutableListOf in Kotlin?
In Kotlin, listOf and mutableListOf are functions used to create immutable and mutable lists, respectively. The primary difference between them lies in their mutability:
listOf: The listOf function creates an immutable list, meaning once the list is created, its elements cannot be added, removed, or modified. It provides a read-only view of the list’s elements. For example:
kotlin val immutableList = listOf("apple", "banana", "orange")
mutableListOf: The mutableListOf function creates a mutable list, allowing you to add, remove, or modify elements after the list is created. Mutable lists provide flexibility in manipulating data at runtime. For example:
kotlin val mutableList = mutableListOf("apple", "banana", "orange") mutableList.add("grape")
ListOf creates an immutable list, while mutableListOf creates a mutable list. The choice between them depends on whether you need the ability to modify the list’s contents after creation. Immutable lists offer safety and thread-safety guarantees, while mutable lists provide flexibility but require careful handling to avoid unintended modifications.
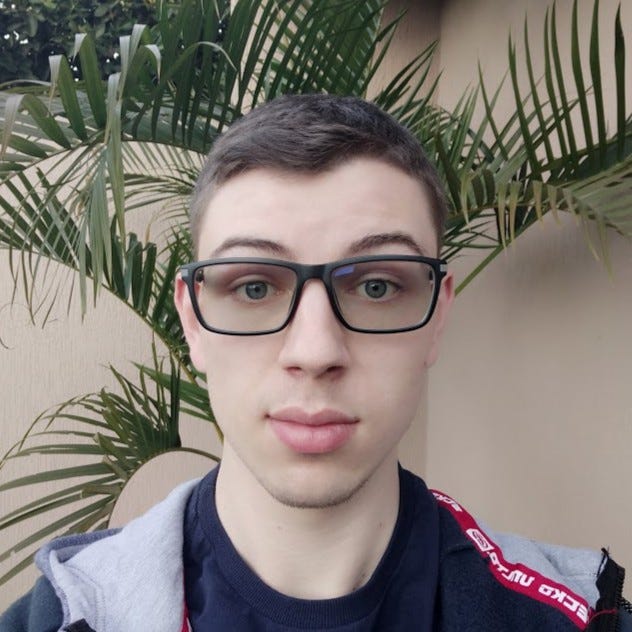
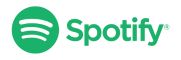