Unlocking AI Potentials: How Kotlin Integrates Machine Learning in Android?
Machine Learning (ML) has taken the tech world by storm, and its application isn’t limited to just data analytics or robotics. Android app development, empowered by the elegance of Kotlin, has started incorporating ML to revolutionize the user experience. Kotlin, the official language for Android app development, brings a clean syntax, concise code, and the power to make ML integration seamless.
In this post, we’ll explore how Kotlin enhances the machine learning experience for Android developers and provide hands-on examples.
1. Why Kotlin for ML in Android?
- Interoperability with Java: Kotlin is fully interoperable with Java, which means you can use all the existing Java-based ML libraries without any hassle.
- Conciseness: With Kotlin’s concise syntax, developers write less code, which leads to fewer bugs. This especially helps when writing intricate ML algorithms or processing data.
- Performance: Kotlin’s efficiency and inline functions allow you to optimize your ML computations without affecting the readability of your code.
2. Implementing Machine Learning in Kotlin-based Android Apps
Let’s dive into some practical examples.
2.1. Image Classification with TensorFlow Lite
Setting up TensorFlow Lite in Android Studio:
– Add the dependency in `build.gradle`:
```kotlin implementation 'org.tensorflow:tensorflow-lite:0.0.0' // Check the latest version ```
Example:
Imagine you’re building an app to classify images of fruits.
– First, train your model using TensorFlow, then convert it to the `.tflite` format.
– Load the model into your Android app.
– Process the image and feed it to the model.
```kotlin val model = TensorImage.load(ModelExecutionOptions()) val tensorImage = TensorImage(DataType.UINT8) tensorImage.load(image) // your input image val outputs = model.process(tensorImage) val probabilityProcessor = TensorProcessor.Builder().add(PostProcessingOp()).build() val processedOutputs = probabilityProcessor.process(outputs) val recognizedFruit = processedOutputs.maxByOrNull { it.value }?.key ```
2.2. Predictive Text Input using DL4J
DeepLearning4J (DL4J) is another library suitable for Android development.
3. Setting up DL4J in Android Studio
– Add the required dependencies in `build.gradle`:
```kotlin implementation 'org.deeplearning4j:deeplearning4j-core:1.x.x' implementation 'org.nd4j:nd4j-native-platform:1.x.x' ```
Example:
Let’s say you want to predict the next word in a sequence.
– After setting up your trained model in the app, you can feed the sequence to get predictions.
```kotlin val sequence = "Kotlin is" val net = MultiLayerNetwork.load(File("path_to_your_model"), false) val input = Nd4j.create(sequence.split(" ").map { wordToIndex(it) }.toIntArray()) val predictions = net.rnnTimeStep(input) val nextWord = indexToWord(predictions.argMax().getInt(0)) ```
4. Personalized App Experience with KMeans Clustering
Say you want to group users based on their app behavior to provide a personalized experience.
Setting up Smile (Statistical Machine Intelligence and Learning Engine):
– Add the following to your `build.gradle`:
```kotlin implementation 'com.github.haifengl:smile-core:2.x.x' ```
Example:
Suppose you collect data points like time spent on app, features used, etc. for personalization.
```kotlin val data = arrayOf( doubleArrayOf(1.0, 2.0), // User A doubleArrayOf(4.0, 5.0), // User B // ... Add more data ) val kmeans = KMeans.fit(data, 3) // 3 clusters val clusters = kmeans.predict(data) when (clusters[0]) { // Check cluster for User A 0 -> showPersonalizedContentA() 1 -> showPersonalizedContentB() 2 -> showPersonalizedContentC() } ```
5. Challenges and Considerations
While Kotlin and Android provide a robust environment to integrate ML, developers should:
– Ensure that the trained ML models are lightweight. Android devices have constraints on memory and compute capacity.
– Regularly update ML models to ensure accuracy and account for evolving data patterns.
– Always respect user privacy. Only collect data with user consent and avoid storing sensitive information.
Conclusion
Integrating Machine Learning into Android apps using Kotlin is both feasible and efficient. With a range of tools and libraries available, developers can now bring intelligent features to mobile apps, enhancing user experience and engagement. Whether it’s predicting user behavior, classifying images, or offering personalized content, Kotlin and ML together hold the promise of transforming Android app development in the coming years.
Table of Contents
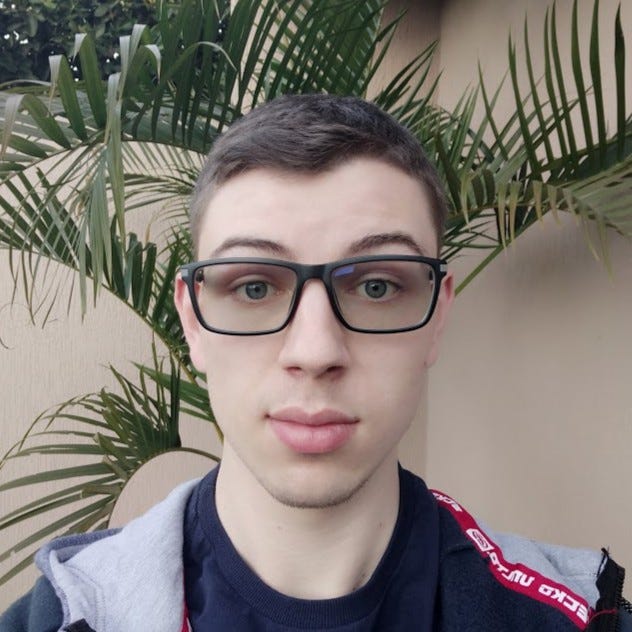
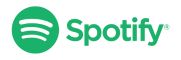