Crafting Elite Kotlin Microservices: A Guide to Spring Boot and Ktor
Kotlin, the statically-typed language from JetBrains, has become a favored choice for many developers, especially in the realm of Android development. But its features don’t end there; Kotlin is also making waves in backend development, particularly with microservices. In this article, we’ll dive deep into building microservices using Kotlin with two prominent frameworks: Spring Boot and Ktor.
1. Spring Boot with Kotlin
Spring Boot, a project from the larger Spring ecosystem, is a widely used tool to build standalone, production-ready applications. With its auto-configuration and embedded server, it’s never been easier to run Java-based applications, and this includes applications written in Kotlin!
Example: A simple Spring Boot service
Here’s a simple microservice in Kotlin using Spring Boot:
```kotlin import org.springframework.boot.autoconfigure.SpringBootApplication import org.springframework.boot.runApplication import org.springframework.web.bind.annotation.GetMapping import org.springframework.web.bind.annotation.RestController @SpringBootApplication @RestController class Application { @GetMapping("/") fun greet(): String = "Hello from Spring Boot!" } fun main(args: Array<String>) { runApplication<Application>(*args) } ```
Here’s what we did:
- Annotated the main class with `@SpringBootApplication`.
- Added a REST endpoint by annotating a method with `@GetMapping` and the class with `@RestController`.
- Ran the application using `runApplication`.
2. Ktor with Kotlin
Ktor, also from JetBrains, is an asynchronous framework specifically designed for creating microservices in Kotlin. Its reactive nature makes it suitable for high-performance applications.
Example: A simple Ktor service
Let’s build a microservice using Ktor:
```kotlin import io.ktor.application.* import io.ktor.response.* import io.ktor.routing.* import io.ktor.server.engine.embeddedServer import io.ktor.server.netty.Netty fun main() { embeddedServer(Netty, port = 8080) { routing { get("/") { call.respondText("Hello from Ktor!") } } }.start(wait = true) } ```
Steps:
- Used the `embeddedServer` from Ktor with Netty as the engine.
- Defined the routes using the `routing` DSL.
- Responded to a GET request on the root path.
3. Comparing Spring Boot and Ktor
- Maturity & Ecosystem: Spring Boot has been around for a while and has a vast ecosystem, including Spring Cloud for microservices. Ktor, while mature, is newer and has a smaller ecosystem.
- Learning Curve: Ktor offers a straightforward approach to building microservices, ideal for those who want a lightweight, “Kotlin-ish” way. Spring Boot, while more feature-rich, has a steeper learning curve.
- Performance: Ktor, being asynchronous, can offer better performance for I/O-bound tasks. However, Spring Boot’s reactive stack, Spring WebFlux, can also achieve similar results.
- Flexibility: Ktor is highly modular, allowing you to pick and choose features. Spring Boot, with its auto-configuration, makes assumptions but can be fine-tuned.
- Community Support: Both frameworks have strong community support, but Spring Boot, being part of the larger Spring ecosystem, might have a wider reach.
Conclusion
Whether you choose Spring Boot or Ktor depends on your specific needs:
– For projects that need a comprehensive solution with a vast ecosystem, especially if integrating with other Spring projects, Spring Boot is the way to go.
– If you’re after a lightweight, reactive, and Kotlin-centric solution for building microservices, Ktor might be your pick.
Both frameworks allow you to leverage Kotlin’s features, making backend development concise and expressive. With Kotlin at the helm, it’s a great time to dive into microservice development, regardless of the framework you choose.
To hire pre- vetted Kotlin developers from CloudDevs, get in touch with our consultants today.
Table of Contents
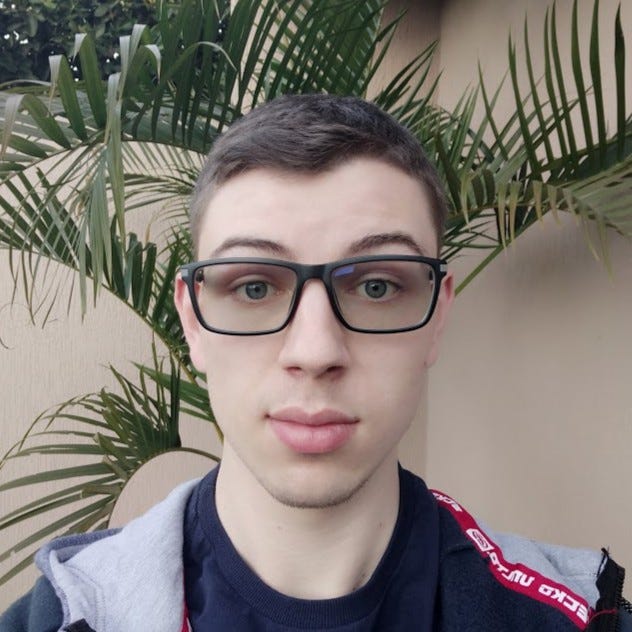
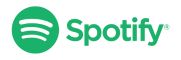