Kotlin and Mobile Analytics: Measuring User Engagement
In the competitive world of mobile app development, understanding and measuring user engagement is key to delivering a successful product. Kotlin, with its modern syntax and powerful features, offers an excellent environment for implementing mobile analytics. This blog explores how Kotlin can be leveraged to track and analyze user engagement, providing practical examples and code snippets to guide your implementation.
Understanding User Engagement
User engagement refers to how users interact with your app and can be measured through various metrics such as session length, screen views, and user retention. Analyzing these metrics helps in identifying user behavior patterns and optimizing the app experience to improve user satisfaction and retention.
Using Kotlin for Mobile Analytics
Kotlin’s concise syntax and robust libraries make it a great choice for implementing mobile analytics. Here are key aspects and code examples demonstrating how Kotlin can be used for tracking and analyzing user engagement.
1. Integrating Analytics SDKs
One of the first steps in measuring user engagement is integrating an analytics SDK into your app. Kotlin works seamlessly with popular analytics services like Google Analytics and Firebase Analytics.
Example: Integrating Firebase Analytics
To integrate Firebase Analytics into your Kotlin app, follow these steps:
– Add Firebase to your project by including the necessary dependencies in your `build.gradle` file:
```kotlin dependencies { implementation 'com.google.firebase:firebase-analytics:21.0.0' } ```
– Initialize Firebase Analytics in your application class:
```kotlin import android.app.Application import com.google.firebase.analytics.FirebaseAnalytics class MyApp : Application() { lateinit var firebaseAnalytics: FirebaseAnalytics override fun onCreate() { super.onCreate() firebaseAnalytics = FirebaseAnalytics.getInstance(this) } } ```
– Log events to track user actions:
```kotlin import com.google.firebase.analytics.FirebaseAnalytics fun logUserEngagementEvent(eventName: String, params: Bundle) { firebaseAnalytics.logEvent(eventName, params) } ```
2. Tracking User Sessions
Tracking user sessions is crucial for understanding how often and how long users interact with your app.
Example: Measuring Session Duration
You can measure session duration by tracking app foreground and background events:
```kotlin import android.os.Bundle import android.app.Activity import com.google.firebase.analytics.FirebaseAnalytics class MainActivity : Activity() { private lateinit var firebaseAnalytics: FirebaseAnalytics private var sessionStartTime: Long = 0 override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) firebaseAnalytics = FirebaseAnalytics.getInstance(this) sessionStartTime = System.currentTimeMillis() } override fun onPause() { super.onPause() val sessionDuration = System.currentTimeMillis() - sessionStartTime val bundle = Bundle().apply { putLong("session_duration", sessionDuration) } firebaseAnalytics.logEvent("session_duration", bundle) } } ```
3. Analyzing User Behavior
Understanding how users navigate through your app can provide insights into their behavior and preferences.
Example: Tracking Screen Views
Use Firebase Analytics to track screen views and user flow:
```kotlin import com.google.firebase.analytics.FirebaseAnalytics fun trackScreenView(screenName: String) { val bundle = Bundle().apply { putString(FirebaseAnalytics.Param.SCREEN_NAME, screenName) } firebaseAnalytics.logEvent(FirebaseAnalytics.Event.SCREEN_VIEW, bundle) } ```
4. Visualizing Analytics Data
Visualizing data helps in making sense of complex metrics and trends. While Firebase Analytics provides a web-based dashboard, you might also want to visualize data directly within your app or on your server.
Example: Creating Custom Reports
For custom reports, you might fetch analytics data and visualize it using chart libraries such as MPAndroidChart.
```kotlin import com.github.mikephil.charting.Chart import com.github.mikephil.charting.charts.LineChart import com.github.mikephil.charting.data.LineData import com.github.mikephil.charting.data.LineDataSet import android.os.Bundle import androidx.appcompat.app.AppCompatActivity class AnalyticsActivity : AppCompatActivity() { private lateinit var lineChart: LineChart override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_analytics) lineChart = findViewById(R.id.lineChart) val data = listOf(10f, 20f, 30f) // Example data val dataSet = LineDataSet(data.mapIndexed { index, value -> Entry(index.toFloat(), value) }, "User Engagement") val lineData = LineData(dataSet) lineChart.data = lineData lineChart.invalidate() // Refresh chart } } ```
Conclusion
Kotlin provides a versatile and modern approach to implementing mobile analytics, allowing developers to effectively measure and analyze user engagement. By integrating analytics SDKs, tracking sessions, analyzing behavior, and visualizing data, you can gain valuable insights into how users interact with your app and make data-driven decisions to enhance their experience.
Further Reading:
Table of Contents
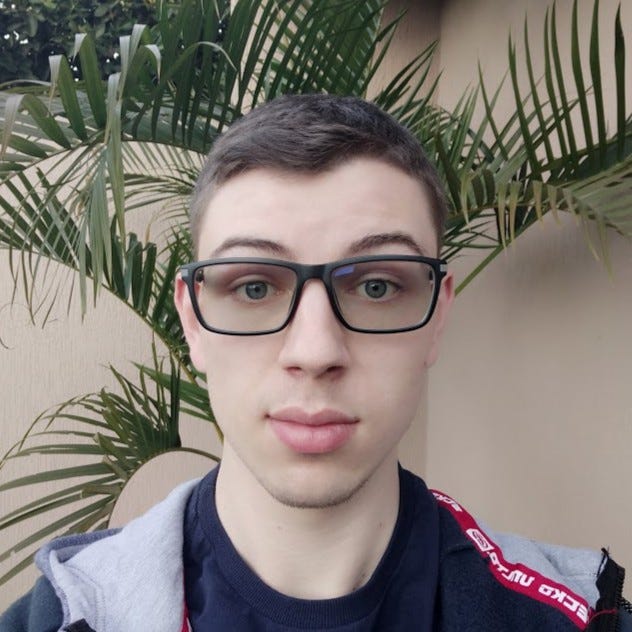
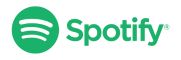