Kotlin and Mobile Payments: Integrating Payment Gateways
Mobile payments have become an integral part of modern commerce, allowing users to make transactions quickly and securely via their smartphones. Kotlin, as a modern and versatile programming language, offers powerful tools for integrating payment gateways into mobile applications. This article explores how Kotlin can be utilized for mobile payment integration, complete with practical examples to help you implement seamless payment solutions.
Understanding Mobile Payments and Payment Gateways
Mobile payments involve transactions made through mobile devices, using various methods like credit cards, digital wallets, and bank transfers. Payment gateways facilitate these transactions by securely processing payment information and ensuring the smooth flow of funds between users and merchants.
Using Kotlin for Payment Gateway Integration
Kotlin, with its concise syntax and interoperability with Java, is an excellent choice for developing Android applications that integrate payment gateways. Below, we explore key aspects of integrating payment gateways using Kotlin, including code examples for practical implementation.
1. Integrating Payment Gateways
The first step in integrating a payment gateway is setting up the necessary SDKs and libraries provided by the payment gateway service. Kotlin can be used to configure and manage these integrations.
Example: Integrating Stripe Payment Gateway
Stripe is a popular payment gateway with a robust SDK for Android. Here’s how you can integrate Stripe using Kotlin:
1. Add Stripe Dependency:
Include the Stripe SDK in your `build.gradle` file:
```gradle implementation 'com.stripe:stripe-android:21.6.1' ```
2. Initialize Stripe in Your App:
```kotlin import com.stripe.android.PaymentConfiguration class MyApplication : Application() { override fun onCreate() { super.onCreate() PaymentConfiguration.init( applicationContext, "your_publishable_key" ) } } ```
3. Create Payment Intent and Handle Payment:
```kotlin import com.stripe.android.model.PaymentIntentParams import com.stripe.android.paymentsheet.PaymentSheet class PaymentActivity : AppCompatActivity() { private lateinit var paymentSheet: PaymentSheet override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_payment) paymentSheet = PaymentSheet(this, ::onPaymentSheetResult) // Call your backend to create a PaymentIntent and fetch the client secret // Then, use the client secret to present the payment sheet } private fun presentPaymentSheet(clientSecret: String) { val paymentIntentParams = PaymentIntentParams.createConfirmPaymentIntentWithPaymentMethodId( "pm_card_visa", // Payment method ID clientSecret ) paymentSheet.presentWithPaymentIntent(paymentIntentParams) } private fun onPaymentSheetResult(paymentResult: PaymentSheetResult) { when (paymentResult) { is PaymentSheetResult.Completed -> { // Handle successful payment } is PaymentSheetResult.Failed -> { // Handle payment failure } } } } ```
2. Handling Payment Responses
Once a payment is processed, handling the response and updating your app’s UI is crucial for providing a smooth user experience.
Example: Handling Payment Response in Kotlin
```kotlin import com.stripe.android.paymentsheet.PaymentSheetResult class PaymentActivity : AppCompatActivity() { private fun onPaymentSheetResult(paymentResult: PaymentSheetResult) { when (paymentResult) { is PaymentSheetResult.Completed -> { // Notify user of successful payment Toast.makeText(this, "Payment Successful!", Toast.LENGTH_SHORT).show() } is PaymentSheetResult.Failed -> { // Notify user of payment failure Toast.makeText(this, "Payment Failed: ${paymentResult.error.message}", Toast.LENGTH_LONG).show() } is PaymentSheetResult.Canceled -> { // Notify user of payment cancellation Toast.makeText(this, "Payment Canceled", Toast.LENGTH_SHORT).show() } } } } ```
3. Testing Payment Integration
Testing is crucial to ensure that your payment integration works seamlessly. Use test cards and sandbox environments provided by payment gateways to simulate transactions and verify functionality.
Example: Using Test Cards for Stripe Integration
Stripe provides test card numbers that you can use for testing:
```kotlin // Example test card number for Stripe val testCardNumber = "4242424242424242" ```
4. Securing Payment Transactions
Security is paramount in handling payment information. Ensure that your app complies with PCI-DSS standards and uses secure connections (HTTPS) for communication.
Example: Ensuring Secure Communication
```kotlin val url = "https://api.yourpaymentgateway.com/endpoint" // Ensure you use HTTPS and validate SSL certificates ```
Conclusion
Kotlin provides a modern and efficient approach to integrating payment gateways into mobile applications. By leveraging Kotlin’s features and the robust SDKs provided by payment gateway services, you can create a seamless and secure payment experience for users. Following best practices for payment integration and security will help ensure that your application offers reliable and trustworthy payment solutions.
Further Reading:
Table of Contents
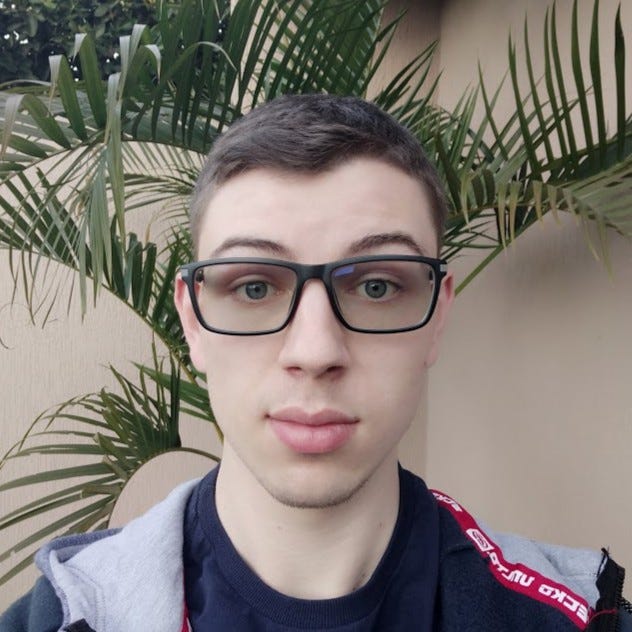
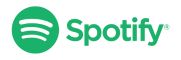