Creating Kotlin Native Applications: Beyond Android Development
In the realm of modern software development, Kotlin has gained immense popularity as a programming language, especially for Android app development. Its concise syntax, null safety, and strong type inference make it a favorite among developers. However, Kotlin is not limited to just Android development; it also offers a powerful feature known as Kotlin Native, enabling developers to build applications that run natively on multiple platforms, including iOS, macOS, Windows, and Linux. In this blog post, we will explore the world beyond Android and dive into the fascinating world of Kotlin Native.
1. Understanding Kotlin Native
Kotlin Native is an exciting technology that allows developers to compile Kotlin code directly into machine code instead of converting it to Java bytecode. This opens up a world of opportunities to create high-performance, native applications on various platforms. Kotlin Native leverages LLVM (Low-Level Virtual Machine), a versatile compiler infrastructure, to generate efficient machine code for different target platforms.
2. Advantages of Kotlin Native
2.1. Cross-platform Development
Kotlin Native enables cross-platform development, allowing you to write code once and run it on multiple platforms. This eliminates the need for separate codebases for different platforms, saving time and effort in the development process. Whether you want to target iOS, macOS, Windows, or Linux, Kotlin Native has got you covered.
2.2. Performance
Since Kotlin Native generates machine code, the performance of the resulting native application is exceptional. The absence of an intermediary virtual machine (such as the JVM for Android) allows Kotlin Native applications to run closer to the metal, optimizing CPU and memory utilization.
2.3. Access to Native APIs
With Kotlin Native, you can directly access platform-specific APIs and libraries, giving you greater control over your application’s behavior. This is particularly useful when you need to implement platform-specific features or leverage platform-specific performance optimizations.
2.4. Code Sharing with Kotlin Multiplatform
Kotlin Native plays well with Kotlin Multiplatform, which enables code sharing between different platforms. This synergy allows you to share common business logic and data models across your Android, iOS, and other applications, reducing redundancy and promoting code consistency.
3. Getting Started with Kotlin Native
To begin your Kotlin Native journey, you need to set up the development environment. Follow the steps below to get started:
Step 1: Installing Kotlin Native
First, make sure you have Kotlin installed on your system. You can download Kotlin from the official website or use a package manager like Homebrew (on macOS) or SDKMAN. To install Kotlin Native, you’ll need the Kotlin command-line tools and the Kotlin/Native plugin for Gradle.
Step 2: Creating a Kotlin Native Project
To create a new Kotlin Native project, use the konanc command-line tool or the Gradle Kotlin Native plugin. For instance, with the Gradle plugin, you can run the following commands:
bash # Create a new Kotlin Native project gradle init --type kotlin-native # Build the project ./gradlew build
Step 3: Project Structure
A typical Kotlin Native project has the following structure:
markdown - src - commonMain - kotlin - # Common Kotlin code shared across platforms - platformNameMain - kotlin - # Platform-specific Kotlin code (e.g., iosMain, macosMain, windowsMain, linuxMain) - platformNameTest - kotlin - # Platform-specific tests (e.g., iosTest, macosTest, windowsTest, linuxTest) - build.gradle.kts - gradle.properties - settings.gradle.kts
4. Kotlin Native Code Samples
Now that we have our Kotlin Native project set up, let’s take a look at some code samples to get a better understanding of how to leverage Kotlin Native for cross-platform development.
Sample 1: Platform-specific API Usage
kotlin import platform.posix.* fun platformSpecificSleep(seconds: Int) { // Use the appropriate sleep function based on the target platform if (PlatformUtils.isMacOS || PlatformUtils.isiOS) { usleep((seconds * 1000).toUInt()) } else { // For other platforms, use standard sleep function sleep(seconds.toUInt()) } } fun main() { platformSpecificSleep(3) println("Wake up after sleeping for 3 seconds!") }
In this example, we’re using platform-specific APIs for sleeping. The usleep function is used on macOS and iOS, while the standard sleep function is used on other platforms.
Sample 2: Shared Code with Kotlin Multiplatform
kotlin expect fun getCurrentPlatform(): String fun main() { val platform = getCurrentPlatform() println("Running on $platform") }
In this code sample, we are using the expect keyword to declare a platform-specific function. The actual implementation of getCurrentPlatform() will be provided separately for each platform (e.g., iOS, macOS, Windows, Linux).
Conclusion
Kotlin Native expands the horizons for Kotlin beyond Android development, offering developers a powerful toolset for building cross-platform applications with native performance. The ability to leverage native APIs, share code with Kotlin Multiplatform, and create high-performance applications on various platforms makes Kotlin Native an excellent choice for modern software development. So, whether you’re targeting iOS, macOS, Windows, or Linux, give Kotlin Native a try and explore the endless possibilities of cross-platform development! Happy coding!
Table of Contents
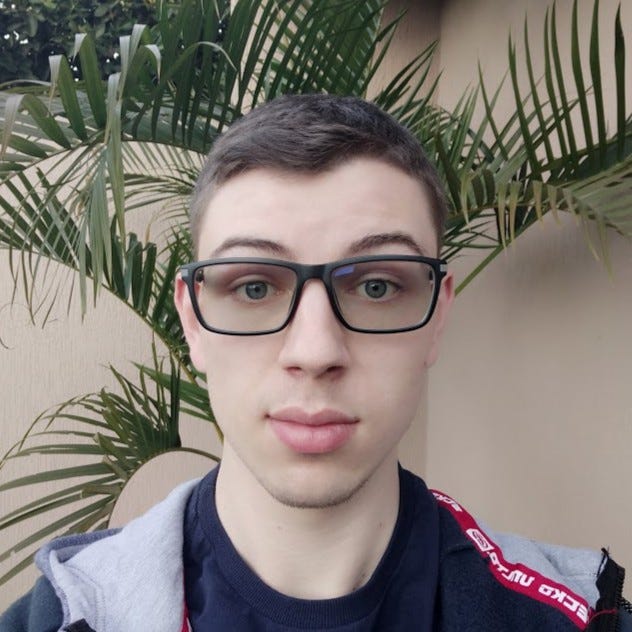
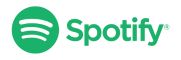