Kotlin and OpenCV: The Ultimate Pair for Android Vision Projects
The combination of OpenCV, a powerful open-source computer vision library, and Kotlin, a modern programming language for Android app development, provides an impressive platform for creating robust computer vision applications on Android. In this article, we’ll delve into how to integrate OpenCV in a Kotlin Android application and explore a few examples that demonstrate the power of computer vision.
1. Setting Up OpenCV with Kotlin in Android
Before diving into the examples, it’s essential to set up OpenCV correctly in your Kotlin project.
Prerequisites:
– Android Studio
– Kotlin plugin for Android Studio
– OpenCV Android SDK
Steps:
- Download and Import the OpenCV SDK for Android: Download the SDK from the official OpenCV website and extract it. In Android Studio, choose ‘File’ -> ‘Import Module’ and select the ‘sdk/java’ folder from the extracted files.
- Add Native Libraries: Copy the native libraries from the OpenCV Android SDK (`OpenCV-android-sdk/sdk/native/libs/`) to your Android project’s `app/src/main/jniLibs` directory.
- Update the build.gradle: Add the OpenCV library dependency in the `app/build.gradle`:
```kotlin implementation project(path: ':openCVLibraryXXX') // where XXX is the version ```
- Load OpenCV: Before using OpenCV functions, you need to load the library. It’s often done in the main activity or application class:
```kotlin if (!OpenCVLoader.initDebug()) { Log.e(TAG, "OpenCV initialization failed.") } else { Log.d(TAG, "OpenCV initialization succeeded.") } ```
Now, let’s dive into some practical examples!
2. Examples
2.1. Image Grayscale:
Converting an image to grayscale is a fundamental operation in image processing. Here’s how you can achieve it using OpenCV and Kotlin:
```kotlin fun convertToGrayScale(input: Mat): Mat { val gray = Mat() Imgproc.cvtColor(input, gray, Imgproc.COLOR_BGR2GRAY) return gray } ```
2.2. Face Detection:
Using OpenCV’s pre-trained classifiers, detecting faces in an image becomes straightforward:
```kotlin fun detectFaces(input: Mat): Mat { val faceCascade = CascadeClassifier() faceCascade.load("path_to_haar_xml") // Load the XML file of the classifier val faces = MatOfRect() faceCascade.detectMultiScale(input, faces) for (rect in faces.toArray()) { Imgproc.rectangle(input, rect.tl(), rect.br(), Scalar(0.0, 255.0, 0.0), 2) } return input } ```
2.3. Edge Detection:
Edge detection highlights significant edges within an image, making it crucial for many computer vision task
```kotlin fun detectEdges(input: Mat): Mat { val edges = Mat() Imgproc.Canny(input, edges, 50.0, 150.0) return edges } ```
2.4. Feature Matching:
Matching features between two images can be helpful for tasks like image stitching or object recognition:
```kotlin fun matchFeatures(img1: Mat, img2: Mat): Mat { val keypoints1 = MatOfKeyPoint() val keypoints2 = MatOfKeyPoint() val descriptors1 = Mat() val descriptors2 = Mat() val detector = ORB.create() detector.detectAndCompute(img1, Mat(), keypoints1, descriptors1) detector.detectAndCompute(img2, Mat(), keypoints2, descriptors2) val matcher = DescriptorMatcher.create(DescriptorMatcher.BRUTEFORCE_HAMMING) val matches = MatOfDMatch() matcher.match(descriptors1, descriptors2, matches) val output = Mat() Features2d.drawMatches(img1, keypoints1, img2, keypoints2, matches, output) return output } ```
Conclusion
By marrying the capabilities of OpenCV with the expressiveness of Kotlin, developers can efficiently build powerful computer vision applications on Android. Whether you’re developing a facial recognition tool, a photo editing application, or any other vision-centric app, the combined power of Kotlin and OpenCV offers you a versatile platform to bring your ideas to life.
Remember to always check the OpenCV documentation and Kotlin references for any updates or advanced functionalities that might be introduced in the future. As the tech world evolves, so do the possibilities of what you can achieve. Happy coding!
Table of Contents
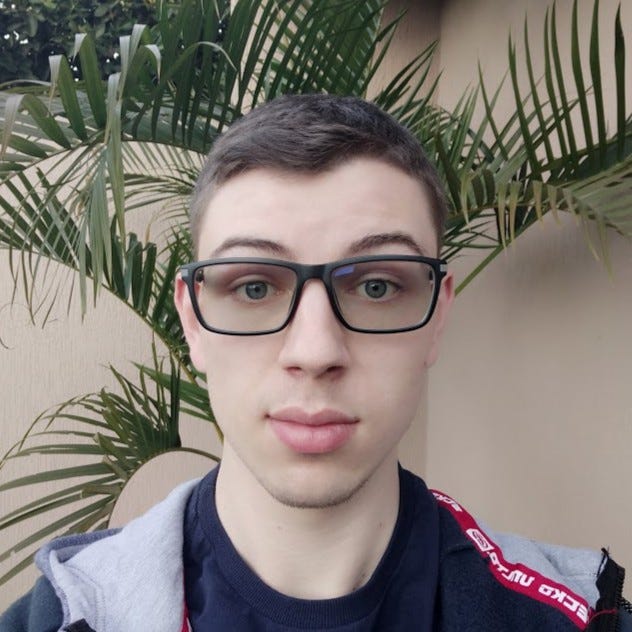
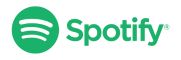