What is the primary constructor in Kotlin?
The primary constructor in Kotlin is a concise and powerful feature that enables the declaration of class properties and initialization logic directly within the class header. Unlike traditional languages where constructors are separate entities, Kotlin integrates constructor functionality directly into the class declaration, promoting clarity and brevity in code.
The primary constructor in Kotlin is declared immediately after the class name, within parentheses. It allows you to define properties and initialize them using constructor parameters, all in one concise declaration. This constructor is invoked implicitly when an instance of the class is created.
One notable aspect of the primary constructor is its ability to declare properties directly within its parameter list. By prefixing constructor parameters with either val or var, you can declare immutable or mutable properties respectively, streamlining the process of defining class properties.
For example:
kotlin class Person(val name: String, var age: Int)
In this example, name is an immutable property initialized using the constructor parameter, while age is a mutable property.
Furthermore, the primary constructor allows for initialization logic to be executed directly within the class header, simplifying the process of setting up object state during instantiation.
kotlin class Person(val name: String, var age: Int) { init { println("Person object created with name $name and age $age") } }
Here, the init block within the class body is part of the primary constructor and executes initialization logic when an instance of the Person class is created.
The primary constructor serves as a foundational aspect of Kotlin’s concise and expressive syntax, allowing for the declaration of properties and initialization logic in a single, streamlined declaration. Its integration into the class header enhances readability and maintainability, enabling developers to focus on defining class properties and behavior in a clear and concise manner.
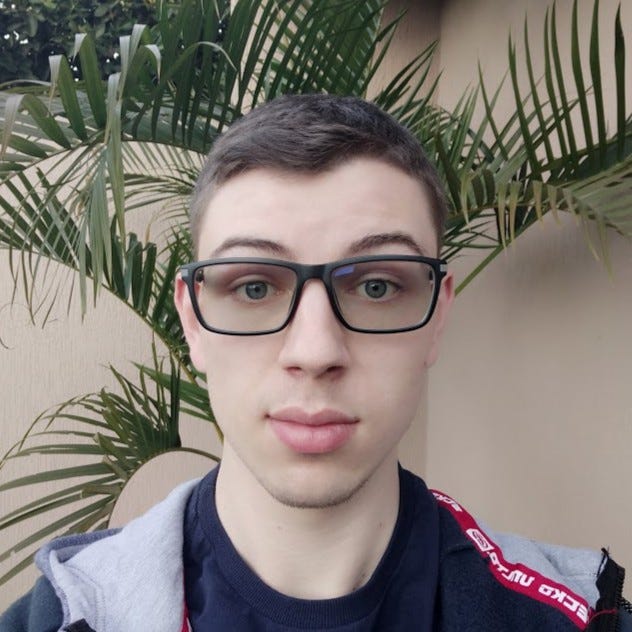
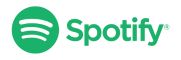