What are properties and methods in Kotlin classes?
In Kotlin, classes serve as blueprints for creating objects, encapsulating both data and behavior. Properties and methods are essential components of Kotlin classes that allow for the representation of state and actions associated with objects.
Properties in Kotlin classes represent the state or characteristics of objects. They are essentially variables declared within a class. Properties can be declared as mutable (var) or immutable (val). Immutable properties cannot be changed once initialized, while mutable properties can be reassigned new values.
For example:
kotlin class Person { val name: String = "John" // Immutable property var age: Int = 30 // Mutable property }
In this example, name is an immutable property initialized with a constant value, while age is a mutable property that can be modified.
Methods in Kotlin classes represent actions or behaviors that objects can perform. They are essentially functions defined within a class. Methods can manipulate the state of the object (properties) and perform various operations.
For example:
kotlin class Person { var name: String = "" fun greet() { println("Hello, my name is $name") } }
In this example, greet() is a method defined within the Person class, which prints a greeting message using the name property.
Properties and methods work together to define the behavior and characteristics of objects in Kotlin classes. They encapsulate the internal state and behavior of objects, providing a clean and modular way to organize code and represent real-world entities.
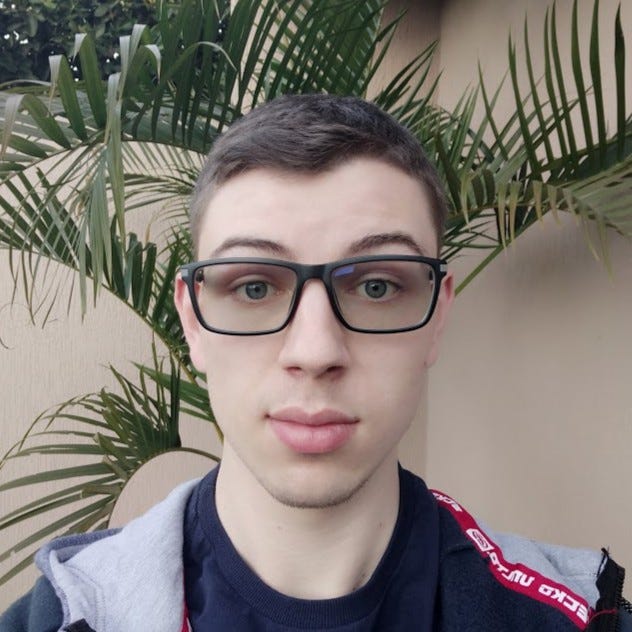
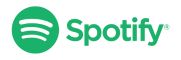