Kotlin and ReactiveUI: A Match Made for Dynamic UI Excellence
The modern app development landscape demands more fluid, interactive, and responsive user interfaces than ever before. Gone are the days when a simple request-response cycle would suffice. Users now want instantaneous feedback, smooth transitions, and efficient data handling. Enter reactive programming.
Kotlin, as a modern language for Android and other platforms, provides excellent support for building reactive UIs, especially when combined with ReactiveUI. In this article, we’ll delve into how Kotlin and ReactiveUI can be leveraged to create effective reactive interfaces, supported by concrete examples.
1. Basics of Reactive Programming
Reactive programming is an approach where data streams and the propagation of changes are core to the architecture. Essentially, when a data source changes, this change propagates to components that depend on the data, updating the UI or performing other necessary tasks.
2. Introduction to ReactiveUI
ReactiveUI is a functional reactive programming library for UI development. It provides a consistent way to represent mutable data as observable streams, allowing developers to effortlessly bind UI components to data sources, manage side-effects, and craft complex UI interactions.
3. Kotlin and ReactiveUI: The Perfect Pair
Kotlin, with its expressive syntax, concise codebase, and first-class support for functional programming paradigms, integrates seamlessly with ReactiveUI.
Example: Basic UI Binding
Consider a simple scenario where a TextView needs to display a user’s name, which might change over time.
```kotlin // Model class data class User(var name: String) // UI Binding val user = User("John Doe") val nameObservable = Observable.just(user.name) nameObservable.subscribe { newName -> textView.text = newName } ```
Any changes to the `user.name` will update the `textView` automatically.
Example: Handling UI Events
Let’s say you want to perform a network call when a button is clicked but only if an input field isn’t empty:
```kotlin val inputObservable = RxTextView.textChanges(inputField) val buttonClickObservable = RxView.clicks(submitButton) val canClickObservable = inputObservable.map { it.isNotEmpty() } buttonClickObservable .filter { canClickObservable.blockingFirst() } .subscribe { // Make the network call } ```
This way, the network call only occurs if the input field isn’t empty when the button is clicked.
Example: Combining Multiple Streams
Suppose we want to validate two fields – an email and a password – and only enable the login
button when both are valid:
```kotlin val emailObservable = RxTextView.textChanges(emailField) .map { it.isValidEmail() } val passwordObservable = RxTextView.textChanges(passwordField) .map { it.isValidPassword() } Observable.combineLatest(emailObservable, passwordObservable, BiFunction<Boolean, Boolean, Boolean> { emailValid, passwordValid -> emailValid && passwordValid }).subscribe { isValid -> loginButton.isEnabled = isValid } ```
In this example, the `isValidEmail` and `isValidPassword` are hypothetical extension functions that return a boolean indicating the validity of the content.
4. Side Effects and Threading
ReactiveUI and Kotlin provide powerful tools for managing side effects and handling multi-threading. With Kotlin’s coroutines and ReactiveUI’s scheduling, it’s easy to shift tasks off the UI thread and ensure smooth user experiences.
Example: Making an Asynchronous API Call
Imagine fetching a user profile from an API when the app starts:
```kotlin val apiCallObservable = Observable.fromCallable { // Call the API and fetch the user profile }.subscribeOn(Schedulers.io()) .observeOn(AndroidSchedulers.mainThread()) apiCallObservable.subscribe { userProfile -> // Update UI with the user profile } ```
In the above code, `subscribeOn` determines the thread where the API call runs, while `observeOn` switches back to the UI thread to update the user interface.
5. Advanced Scenarios with Kotlin and ReactiveUI
Example: Debouncing User Input
Consider a search bar where you want to make an API call based on user input but not with every single character change:
```kotlin val searchObservable = RxTextView.textChanges(searchField) .debounce(300, TimeUnit.MILLISECONDS) .distinctUntilChanged() .subscribeOn(Schedulers.io()) .observeOn(AndroidSchedulers.mainThread()) searchObservable.subscribe { searchTerm -> // Execute the search based on searchTerm } ```
By using `debounce`, the API call is deferred until the user stops typing for 300 milliseconds. `distinctUntilChanged` ensures that consecutive duplicate entries are ignored.
Conclusion
ReactiveUI and Kotlin together offer a potent combination for building reactive user interfaces. As we’ve seen through the examples, this pairing allows developers to handle a variety of common (and advanced) UI scenarios with elegance, simplicity, and robustness. The reactive paradigm can initially feel challenging, but once grasped, it opens the door to a new world of fluid and intuitive user interfaces. Happy coding!
Table of Contents
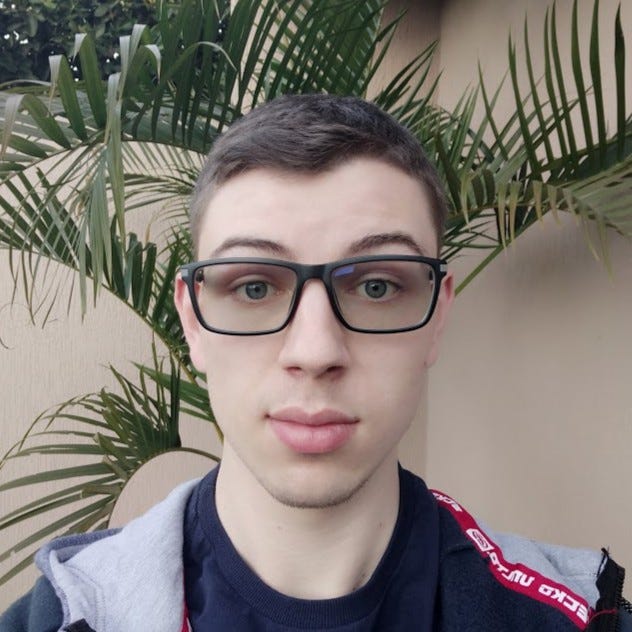
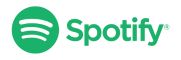