Effortless Android Database Operations: Kotlin and Realm Unleashed
Are you tired of dealing with complex and verbose database operations in your Android app development? Look no further! In this blog post, we’ll explore how Kotlin and Realm can simplify your database operations, making your life as an Android developer much easier.
Table of Contents
1. Why Kotlin and Realm?
Before we dive into the details, let’s briefly discuss why Kotlin and Realm are an excellent combination for Android app development.
Kotlin, a statically typed programming language, has gained immense popularity in the Android development community due to its concise syntax and enhanced null safety features. It seamlessly integrates with Java and offers robust support for modern Android development.
Realm, on the other hand, is a powerful and efficient mobile database that’s designed to work seamlessly with both Java and Kotlin. It provides real-time data synchronization, automatic schema migrations, and high performance, making it an ideal choice for mobile app developers.
2. Setting Up Your Project
To get started with Kotlin and Realm, ensure you have the necessary dependencies in your Android project. You can add Realm to your app’s build.gradle file like this:
```gradle implementation 'io.realm:realm-android:10.4.0' ```
Now, let’s dive into some practical examples of how Kotlin and Realm simplify database operations.
3. Initializing Realm
Before you can perform any database operations, you need to initialize Realm in your application. In your `Application` class, add the following code to initialize Realm:
```kotlin import android.app.Application import io.realm.Realm class MyApplication : Application() { override fun onCreate() { super.onCreate() Realm.init(this) } } ```
This code initializes Realm in your application, allowing you to access the database throughout your app.
4. Creating a Realm Object
Realm works with Kotlin data classes seamlessly. Let’s say you’re building a task management app, and you want to create a `Task` class to represent tasks. Here’s how you can define it as a Realm object:
```kotlin import io.realm.RealmObject import io.realm.annotations.PrimaryKey open class Task( @PrimaryKey var id: String = "", var title: String = "", var description: String = "" ) : RealmObject() ```
With just a few annotations, you’ve defined a `Task` class that can be stored in the Realm database.
5. Saving Data to Realm
Now, let’s add a new task to the database. With Kotlin and Realm, it’s as simple as this:
```kotlin fun addTask(task: Task) { val realm = Realm.getDefaultInstance() realm.executeTransaction { realmInstance -> realmInstance.copyToRealmOrUpdate(task) } realm.close() } ```
This code creates an instance of Realm, opens a transaction, and adds the task to the database. It’s concise and easy to understand.
6. Querying Data from Realm
Retrieving data from Realm is just as straightforward. Suppose you want to fetch all tasks with a specific status:
```kotlin fun getTasksWithStatus(status: String): RealmResults<Task> { val realm = Realm.getDefaultInstance() val tasks = realm.where(Task::class.java) .equalTo("status", status) .findAll() realm.close() return tasks } ```
This code queries the database for tasks with a specific status, and the result is returned as a `RealmResults` object.
7. Updating and Deleting Data
Updating and deleting data in Realm is equally convenient. For instance, to update a task’s title:
```kotlin fun updateTaskTitle(taskId: String, newTitle: String) { val realm = Realm.getDefaultInstance() realm.executeTransaction { realmInstance -> val task = realmInstance.where(Task::class.java) .equalTo("id", taskId) .findFirst() task?.title = newTitle } realm.close() } ```
Deleting a task is just as easy:
```kotlin fun deleteTask(taskId: String) { val realm = Realm.getDefaultInstance() realm.executeTransaction { realmInstance -> val task = realmInstance.where(Task::class.java) .equalTo("id", taskId) .findFirst() task?.deleteFromRealm() } realm.close() } ```
These examples demonstrate how Kotlin and Realm simplify database operations in Android app development. You can focus on your app’s logic and features without getting bogged down by intricate database code.
Conclusion
In this blog post, we’ve explored how Kotlin and Realm can streamline your Android app’s database operations. By choosing Kotlin for its concise syntax and Realm for its real-time data synchronization and performance, you can create robust and efficient Android applications.
Kotlin and Realm work seamlessly together, providing you with a powerful toolset to simplify CRUD operations, reduce boilerplate code, and enhance your overall development experience. So, why not give them a try in your next Android project?
Happy coding!
Links for Further Reading:
- Kotlin Official Documentation – https://kotlinlang.org/docs/home.html
- Realm Documentation – https://docs.mongodb.com/realm/sdk/android/
Table of Contents
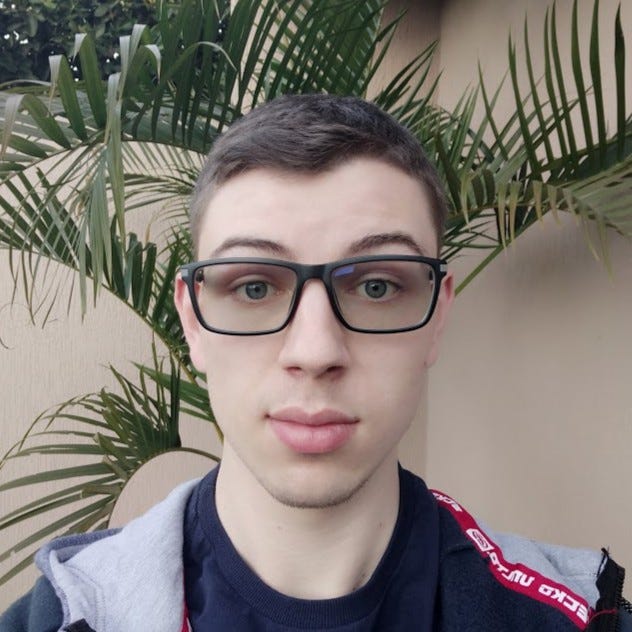
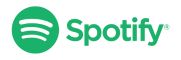