Mastering Metaprogramming: A Deep Dive into Kotlin Reflection
Kotlin, the statically typed programming language from JetBrains, offers an array of advanced features, and among the most powerful of these is Kotlin Reflection. With Kotlin Reflection, you can inspect and manipulate your code during runtime. This opens the door to metaprogramming: the act of writing code that can read, generate, analyze, or transform other code.
This article delves into Kotlin Reflection, showcasing its utility and providing hands-on examples to illustrate its metaprogramming capabilities.
1. Basics of Kotlin Reflection
Reflection in Kotlin is facilitated by the `kotlin-reflect` library. You’ll need to include this in your build file to access reflection features:
```kotlin implementation "org.jetbrains.kotlin:kotlin-reflect:$kotlin_version" ```
Once set up, you can access the class metadata of any object using the `::class` syntax:
```kotlin val stringType = "Hello, World!"::class println(stringType) // Prints: class kotlin.String ```
2. Inspecting Classes and Properties
With Kotlin Reflection, you can delve deep into classes, their properties, and functions.
Example: Fetching Class Properties
```kotlin data class Person(val name: String, val age: Int) val personClass = Person::class for (property in personClass.memberProperties) { println(property.name) } // Outputs: // name // age ```
3. Invoking Functions Dynamically
Kotlin Reflection allows you to call functions in a dynamic fashion, offering flexibility during runtime.
```kotlin fun greet(message: String) { println("Greeting: $message") } val greetFunction = ::greet greetFunction.call("Hello Kotlin Reflection!") // Outputs: Greeting: Hello Kotlin Reflection! ```
4. Accessing Annotations
Annotations are crucial for meta-information, and Kotlin Reflection seamlessly integrates with them.
```kotlin annotation class Info(val description: String) @Info(description = "Sample class for demonstration.") class Sample val annotations = Sample::class.annotations for (annotation in annotations) { if (annotation is Info) { println(annotation.description) } } // Outputs: Sample class for demonstration. ```
5. Modifying Mutable Properties
Reflection also enables property modification for mutable properties:
```kotlin data class MutablePerson(var name: String, var age: Int) val person = MutablePerson("Alice", 25) val propName = MutablePerson::name propName.setter.call(person, "Bob") println(person.name) // Outputs: Bob ```
6. Working with Constructor Parameters
Instantiating objects dynamically is a breeze with Kotlin Reflection:
```kotlin data class Animal(val species: String, val habitat: String) val constructor = Animal::class.primaryConstructor!! val lion = constructor.call("Lion", "Savannah") println(lion) // Outputs: Animal(species=Lion, habitat=Savannah) ```
7. A Practical Use Case: Simple Object Mapper
Imagine you want to map data from one object to another without using third-party libraries. Kotlin Reflection is here to help!
```kotlin data class Source(val name: String, val age: Int) data class Destination(var name: String = "", var age: Int = 0) fun mapData(source: Source, destination: Destination) { val sourceProperties = Source::class.memberProperties for (property in sourceProperties) { val value = property.get(source) val destProperty = Destination::class.memberProperties.find { it.name == property.name } destProperty?.let { (it as KMutableProperty<*>).setter.call(destination, value) } } }
val source = Source("Emma", 30) val destination = Destination() mapData(source, destination) println(destination) // Outputs: Destination(name=Emma, age=30) ```
Conclusion
Kotlin Reflection is a potent tool for metaprogramming. It grants developers the ability to inspect and manipulate code dynamically during runtime, ushering in vast possibilities for code generation, analysis, and transformation.
From inspecting classes to working with annotations, functions, properties, and even creating simple object mappers, Kotlin Reflection has you covered. Dive deep into its extensive documentation, explore its vast capabilities, and leverage its power to craft advanced, dynamic, and flexible Kotlin applications.
Table of Contents
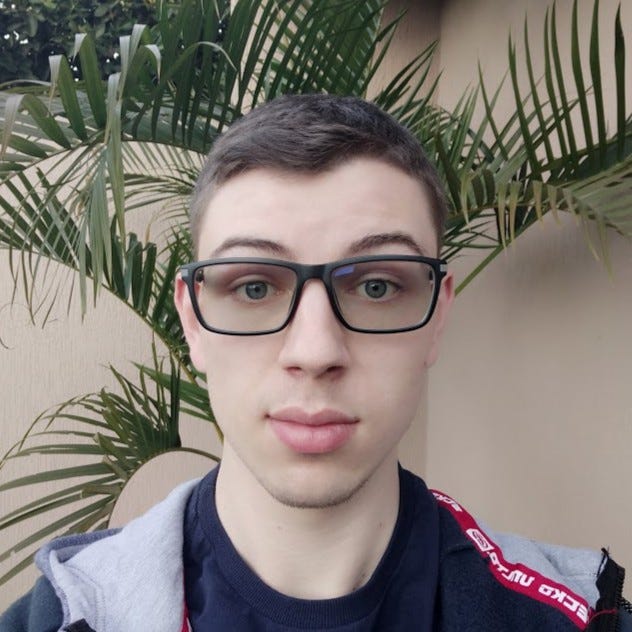
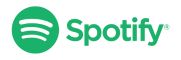