Kotlin and Retrofit: Simplifying Network Calls in Android
In the world of modern Android app development, networking is an essential aspect. Whether it’s fetching data from an API or posting user information to a server, network calls are ubiquitous. However, handling networking tasks can often be complex and error-prone. To streamline this process and make it more efficient, developers often turn to libraries like Retrofit, which, when combined with Kotlin, can simplify the process of making network calls. In this blog, we’ll explore the power of Kotlin and Retrofit in simplifying network calls in Android apps.
1. Why Kotlin?
Before diving into Retrofit and its integration with Android, let’s take a moment to understand why Kotlin has become the preferred language for Android app development.
1.1. Conciseness and Readability
Kotlin offers a concise syntax that reduces boilerplate code, making it easier to read and maintain. Its enhanced functional features, such as extension functions, smart casts, and null safety, help developers write cleaner and more robust code.
1.2. Interoperability
Kotlin is fully interoperable with Java, which means developers can use existing Java libraries, frameworks, and tools in their Kotlin projects. This compatibility makes the transition from Java to Kotlin relatively smooth.
1.3. Enhanced Safety
Kotlin’s type system and null safety features help catch potential runtime errors during compilation, reducing the chances of crashes and NullPointerExceptions.
1.4. Officially Supported by Google
Google has officially endorsed Kotlin as a first-class language for Android development, which means it receives continuous updates and support from both the Kotlin and Android communities.
2. Introducing Retrofit
Retrofit is a popular networking library for Android that simplifies the process of making HTTP requests and handling responses. It is developed and maintained by Square, and its clean and intuitive API design makes it a favorite among Android developers.
2.1. Easy Integration
To use Retrofit in your Android project, add the following dependency to your build.gradle file:
gradle dependencies { implementation 'com.squareup.retrofit2:retrofit:2.9.0' // Other dependencies }
2.2. Retrofit’s Key Components
Retrofit revolves around three key components:
2.2.1. Retrofit Instance
The Retrofit class is the heart of the library, and it handles creating the actual network requests. It can be customized using the Retrofit.Builder class to specify the base URL, converter factories, and more.
2.2.2. API Interface
The API interface is an essential part of Retrofit, as it defines the endpoints and the structure of the network requests. By annotating the methods in the interface with annotations like @GET, @POST, @PUT, etc., developers can specify the HTTP method and the endpoint’s path.
2.2.3. Call Adapter
Retrofit provides call adapters that allow developers to customize how network responses are handled. For instance, the RxJava2CallAdapter allows using RxJava for asynchronous programming with Retrofit.
3. Making Simple GET Request with Retrofit
Let’s go through a step-by-step process of making a simple GET request using Retrofit.
Step 1: Define the Data Model
First, we need to define the data model that represents the response we expect from the API. For example, let’s assume we are making a request to get information about a user:
kotlin data class User(val id: Long, val name: String, val email: String)
Step 2: Create the API Interface
Next, create an interface that defines the API endpoints. For this example, we’ll define a method to get a user by ID:
kotlin interface ApiService { @GET("/users/{id}") suspend fun getUserById(@Path("id") userId: Long): User }
In this example, we use the @GET annotation to specify that this method will make a GET request. The @Path annotation is used to substitute the userId parameter into the endpoint URL.
Step 3: Create the Retrofit Instance
To create a Retrofit instance, build it using the Retrofit.Builder and define the base URL:
kotlin val retrofit = Retrofit.Builder() .baseUrl("https://api.example.com/") .addConverterFactory(GsonConverterFactory.create()) .build()
Here, we use Gson as the converter factory, which automatically converts JSON responses to Kotlin objects.
Step 4: Create an API Service
Create an instance of the API service using the Retrofit instance:
kotlin val apiService = retrofit.create(ApiService::class.java)
Step 5: Make the Network Call
Finally, make the network call from a coroutine (since the method is marked with suspend):
kotlin viewModelScope.launch { try { val userId = 1L val user = apiService.getUserById(userId) // Do something with the user data } catch (e: Exception) { // Handle error } }
That’s it! Retrofit takes care of the rest, and you get the user data in a clean and straightforward way.
4. Handling Errors and Network Failures
While making network calls, error handling and network failure scenarios are crucial aspects to consider. Retrofit simplifies this by providing options to handle errors effectively.
4.1. Error Converter
Retrofit allows you to add a custom error converter to map error responses from the server to your own error model. This allows you to handle different error scenarios in a unified way.
To create a custom error model and error converter, follow these steps:
4.1.1. Define the Error Model
kotlin data class ApiError(val code: Int, val message: String)
4.2.1. Create the Error Converter
kotlin class ApiErrorConverter : Converter<ResponseBody, ApiError> { override fun convert(responseBody: ResponseBody): ApiError? { // Implement logic to parse the error response body } }
4.2.2. Add the Converter to Retrofit
kotlin val retrofit = Retrofit.Builder() .baseUrl("https://api.example.com/") .addConverterFactory(GsonConverterFactory.create()) .addConverterFactory(ApiErrorConverter()) .build()
Now, when an error occurs, Retrofit will use the ApiErrorConverter to parse the response body and give you an ApiError object.
4.2. Network Failures
To handle network failures, you can use Retrofit’s Call class along with the enqueue() method, which performs the network call asynchronously and provides callbacks for success and failure.
kotlin apiService.getUserById(userId).enqueue(object : Callback<User> { override fun onResponse(call: Call<User>, response: Response<User>) { if (response.isSuccessful) { val user = response.body() // Handle successful response } else { // Handle error response } } override fun onFailure(call: Call<User>, t: Throwable) { // Handle network failure } })
Conclusion
In this blog, we explored the powerful combination of Kotlin and Retrofit in simplifying network calls in Android apps. Kotlin’s expressive syntax and robust features make it an excellent language choice for Android development, while Retrofit’s easy integration and clean API design streamline the process of making network requests.
By utilizing Retrofit’s key components like the Retrofit instance, API interface, and call adapters, developers can create a robust and efficient networking layer in their Android apps. Additionally, handling errors and network failures becomes more manageable with Retrofit’s custom error converter and the enqueue() method.
With Kotlin and Retrofit, developers can focus on building innovative features for their Android apps without getting bogged down by the complexities of networking tasks. Happy coding!
Table of Contents
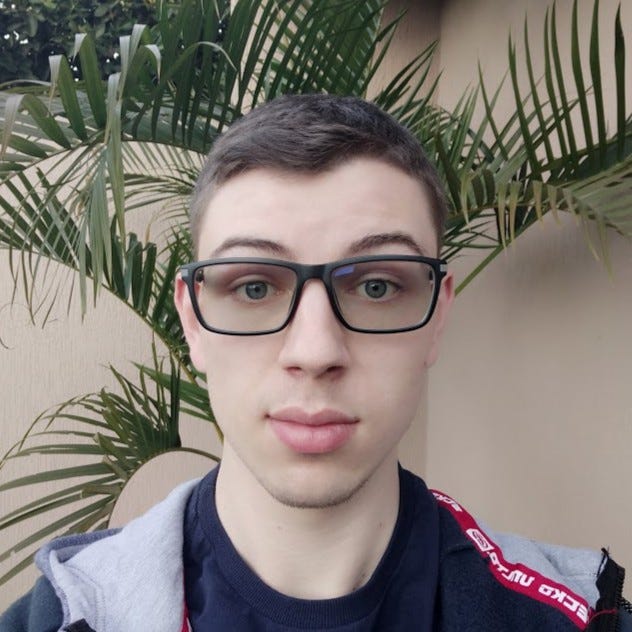
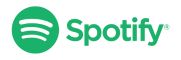