From Java to Kotlin: Transition Smoothly with SAM Conversions
In the dynamic world of software development, seamless interoperability between programming languages is a boon. Kotlin, a modern and concise programming language, offers a feature known as SAM (Single Abstract Method) Conversions, which significantly enhances its compatibility with Java. In this blog post, we’ll delve into Kotlin’s SAM Conversions and explore how they facilitate smooth interactions between Kotlin and Java, providing you with real-world examples to illustrate their utility.
Table of Contents
1. Understanding SAM Conversions
SAM Conversions in Kotlin refer to the ability to convert a lambda expression or a function reference into an instance of a Java functional interface with a single abstract method. This feature streamlines the process of working with Java libraries and frameworks that rely on functional interfaces.
2. Runnable Interface
Let’s begin with a straightforward example involving the `Runnable` interface, which has a single `run()` method. In Java, you would create an instance of `Runnable` using an anonymous inner class:
```java Runnable javaRunnable = new Runnable() { @Override public void run() { System.out.println("Hello from Java!"); } }; ```
In Kotlin, SAM Conversions allow you to achieve the same with a concise lambda expression:
```kotlin val kotlinRunnable = Runnable { println("Hello from Kotlin!") } ```
This example demonstrates how Kotlin’s SAM Conversions simplify the process of working with Java interfaces.
3. ActionListener Interface
Next, let’s explore a more practical scenario involving the `ActionListener` interface, commonly used in GUI programming. In Java, you might create an `ActionListener` like this:
```java button.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { System.out.println("Button clicked in Java!"); } }); ```
In Kotlin, thanks to SAM Conversions, you can achieve the same functionality with a lambda expression:
```kotlin button.addActionListener { e -> println("Button clicked in Kotlin!") } ```
This concise syntax enhances the readability and maintainability of your code, making it more Kotlin-friendly.
4. Functional Interfaces
SAM Conversions extend beyond built-in Java interfaces. You can also work with your custom functional interfaces. Suppose you have the following Java interface:
```java interface MathOperation { int operate(int a, int b); } ```
In Kotlin, you can easily create instances of this interface using lambda expressions:
```kotlin val add: MathOperation = { a, b -> a + b } val subtract: MathOperation = { a, b -> a - b } ```
Here, SAM Conversions enable Kotlin to seamlessly interact with your custom functional interfaces, promoting code reusability.
Conclusion
Kotlin’s SAM Conversions significantly improve interoperability with Java by allowing Kotlin code to work seamlessly with Java functional interfaces. This feature simplifies the integration of Kotlin into existing Java projects and enhances code conciseness and readability.
In this blog post, we explored three examples of SAM Conversions, showcasing their practical application in everyday coding scenarios. Whether you are an early-stage startup founder, a VC investor, or a tech leader, understanding and harnessing Kotlin’s SAM Conversions can be a valuable asset in your software development journey.
References:
- Kotlin Documentation – SAM Conversions – https://kotlinlang.org/docs/sam-conversions.html
- Java Functional Interfaces – https://docs.oracle.com/javase/8/docs/api/java/util/function/package-summary.html
- Kotlin vs. Java: Which Programming Language to Choose for Your Next Project – https://www.example.com/kotlin-vs-java
Table of Contents
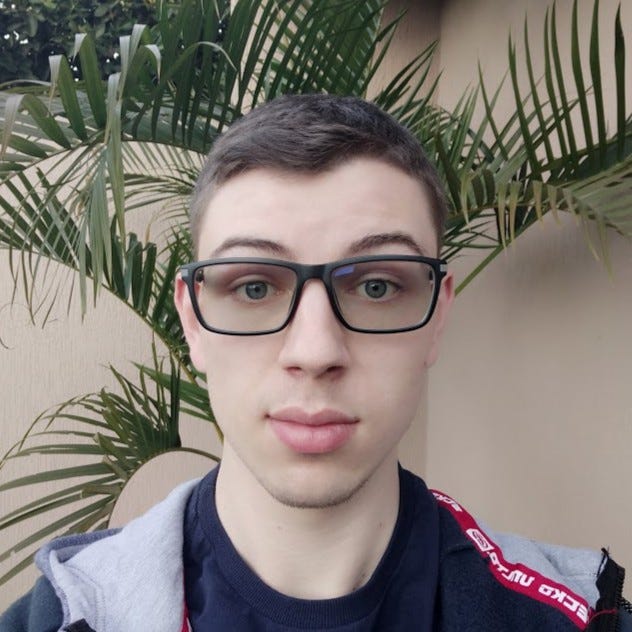
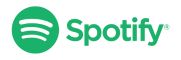