Retrofit Made Easier: Unleash the Power of Kotlin SAM Conversions
In the ever-evolving world of Android app development, one thing remains constant – the need to make network calls to fetch data from APIs. Over the years, several libraries and frameworks have emerged to simplify this process, and one of the most popular choices is Retrofit. In this blog post, we’ll dive into how Kotlin’s Single Abstract Method (SAM) conversions can make your network calls even more concise and elegant when using Retrofit.
Table of Contents
1. What is Kotlin SAM?
Kotlin Single Abstract Method (SAM) conversions are a powerful feature that allows you to treat a lambda expression as an instance of an interface with a single abstract method. This feature was introduced in Kotlin to enhance the readability and conciseness of your code. It’s particularly useful when working with functional interfaces, such as those used in Retrofit’s callback functions.
2. Traditional Retrofit Callbacks
Before we explore Kotlin SAM conversions, let’s take a look at how you would typically make a network call using Retrofit in Java or Kotlin without SAM:
```kotlin val retrofit = Retrofit.Builder() .baseUrl(BASE_URL) .addConverterFactory(GsonConverterFactory.create()) .build() val service = retrofit.create(ApiService::class.java) service.getData().enqueue(object : Callback<DataResponse> { override fun onResponse(call: Call<DataResponse>, response: Response<DataResponse>) { if (response.isSuccessful) { val data = response.body() // Handle data } else { // Handle error } } override fun onFailure(call: Call<DataResponse>, t: Throwable) { // Handle failure } }) ```
While this code works perfectly fine, it can be quite verbose and hard to read, especially when dealing with multiple network calls in a single class. This is where Kotlin SAM conversions come to the rescue.
3. Leveraging Kotlin SAM Conversions
Kotlin’s SAM conversions allow you to simplify the above code significantly. Instead of using the anonymous inner class for the callback, you can use a lambda expression, resulting in cleaner and more concise code:
```kotlin val retrofit = Retrofit.Builder() .baseUrl(BASE_URL) .addConverterFactory(GsonConverterFactory.create()) .build() val service = retrofit.create(ApiService::class.java) service.getData().enqueue { response, throwable -> if (response.isSuccessful) { val data = response.body() // Handle data } else { // Handle error } } ```
With Kotlin SAM conversions, you eliminate the need for verbose callback implementations, making your code more readable and maintainable.
4. Real-world Examples
Let’s explore a couple of real-world scenarios where Kotlin SAM conversions can simplify network calls with Retrofit.
Example 1: Fetching User Profiles
Suppose you have an app that fetches user profiles from a REST API. Traditionally, your code might look like this:
```kotlin userService.getUserProfile(userId).enqueue(object : Callback<UserProfile> { override fun onResponse(call: Call<UserProfile>, response: Response<UserProfile>) { if (response.isSuccessful) { val userProfile = response.body() // Display user profile } else { // Handle error } } override fun onFailure(call: Call<UserProfile>, t: Throwable) { // Handle failure } }) ```
With Kotlin SAM conversions, you can simplify this code as follows:
```kotlin userService.getUserProfile(userId).enqueue { response, throwable -> if (response.isSuccessful) { val userProfile = response.body() // Display user profile } else { // Handle error } } ```
Example 2: Uploading Images
Suppose your app allows users to upload images to a server. Here’s how you might handle the image upload using traditional callbacks:
```kotlin imageService.uploadImage(imageFile).enqueue(object : Callback<UploadResponse> { override fun onResponse(call: Call<UploadResponse>, response: Response<UploadResponse>) { if (response.isSuccessful) { val uploadResponse = response.body() // Handle successful upload } else { // Handle error } } override fun onFailure(call: Call<UploadResponse>, t: Throwable) { // Handle failure } }) ```
With Kotlin SAM conversions, you can make the code more concise:
```kotlin imageService.uploadImage(imageFile).enqueue { response, throwable -> if (response.isSuccessful) { val uploadResponse = response.body() // Handle successful upload } else { // Handle error } } ```
Conclusion
Kotlin’s SAM conversions are a valuable addition to the language, allowing developers to write cleaner and more concise code when working with functional interfaces like Retrofit callbacks. By leveraging this feature, you can simplify your network calls, making your codebase more readable and maintainable.
Incorporating Kotlin SAM conversions into your Android app development workflow can help you save time and reduce boilerplate code, allowing you to focus on building great features and delivering a top-notch user experience.
Links for Further Reading:
- Official Kotlin SAM Conversions Documentation – https://kotlinlang.org/docs/reference/java-interop.html#sam-conversions
- Retrofit Documentation – https://square.github.io/retrofit/
- Kotlin Official Website – https://kotlinlang.org/
Happy coding!
Table of Contents
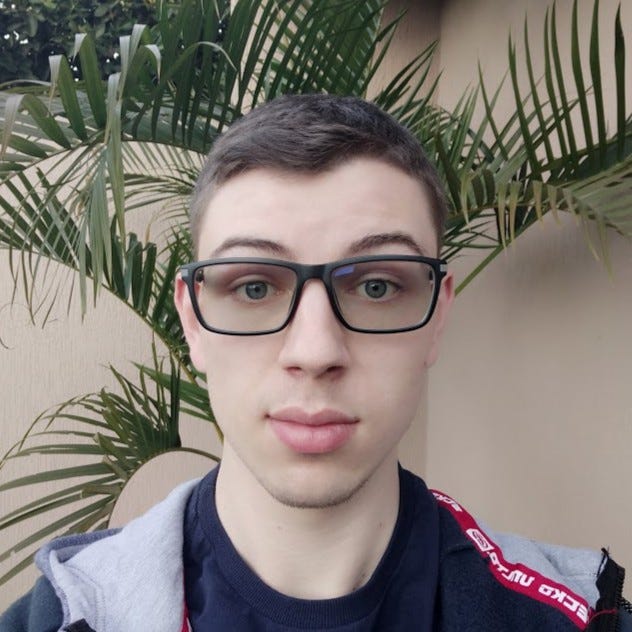
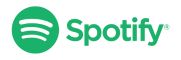