Kotlin’s Sealed Classes: The Ultimate Guide to Boosting Your Code Quality
In the realm of Kotlin, a programming language hailed for its conciseness and safety, sealed classes stand out as one of its unique features. While the traditional enum class is a staple in many languages, including Java, Kotlin takes it a step further by introducing sealed classes. They are used to represent restricted class hierarchies, which means you can have a value that can only be of one of the given types, but with more flexibility and power than enums.
1. What are Sealed Classes?
At its core, a sealed class is an abstract class that cannot be directly instantiated and can have multiple subclasses. However, what makes it different is that these subclasses are known at compile time, which allows us to benefit from exhaustive when statements, ensuring every possibility is handled.
2. Basic Sealed Class Syntax
```kotlin sealed class Weather data class Rainy(val amount: Int) : Weather() data class Sunny(val uvIndex: Int) : Weather() object Cloudy : Weather() ```
Here, `Weather` is a sealed class with three possible types: `Rainy`, `Sunny`, and `Cloudy`.
3. Why Sealed Classes over Enums?
Enums are a great way to represent a fixed set of related constants. However, there are limitations:
- Each enum constant is a single instance, so you can’t have different instances with different properties.
- Enums are less flexible when modeling more complex hierarchies or data structures.
On the other hand, sealed classes:
- Can have multiple instances, each with its properties.
- Can represent hierarchies and complex data structures.
4. Examples Demonstrating Sealed Classes
4.1. State Management:
When developing apps, managing the UI state becomes crucial. Sealed classes make it easy to handle different states.
```kotlin sealed class UiState data class Loading(val message: String) : UiState() data class Success<T>(val data: T) : UiState() data class Error(val error: Throwable) : UiState() fun handleState(state: UiState) { when(state) { is Loading -> print(state.message) is Success<*> -> print("Data: ${state.data}") is Error -> print("Error: ${state.error.message}") } } ```
Here, every UI state is captured by our sealed class, and with an exhaustive when, we ensure we handle every possible state.
4.2. Navigation Events:
In applications, especially mobile ones, navigation plays an integral role. Sealed classes can be used to define navigation events clearly.
```kotlin sealed class NavigationEvent data class GoToProfile(val userId: String) : NavigationEvent() object GoToHome : NavigationEvent() data class OpenSettings(val darkMode: Boolean) : NavigationEvent() fun navigate(event: NavigationEvent) { when(event) { is GoToProfile -> print("Navigating to profile with ID: ${event.userId}") is GoToHome -> print("Navigating to home") is OpenSettings -> print("Opening settings. Dark mode: ${event.darkMode}") } } ```
4.3. Result Wrappers:
In operations that can succeed or fail, a result wrapper using sealed classes can be beneficial.
```kotlin sealed class Result<out T> data class Success<T>(val data: T) : Result<T>() data class Failure(val error: Throwable) : Result<Nothing>() fun fetchUserData(): Result<String> { return try { // Fetching user data logic Success("User Data") } catch (e: Exception) { Failure(e) } } ```
This pattern allows us to gracefully handle success and error scenarios.
5. Benefits of Sealed Classes
- Exhaustive Checks: Kotlin’s when construct checks for exhaustive cases when dealing with sealed classes, ensuring that you’ve handled all possibilities.
- Flexibility: Unlike enums, you can create different instances of a sealed class subtype, each carrying its data.
- Type Safety: With restricted hierarchies, you can be sure that only a specific set of types are permissible, making your code safer.
Conclusion
Kotlin sealed classes offer a superior alternative to traditional enums, especially when modeling complex hierarchies or states. Their flexibility, combined with Kotlin’s type safety and conciseness, makes them an indispensable tool in a Kotlin developer’s toolbox. Whether you’re managing UI states, handling navigation events, or wrapping results, sealed classes pave the way for cleaner and safer code.
Table of Contents
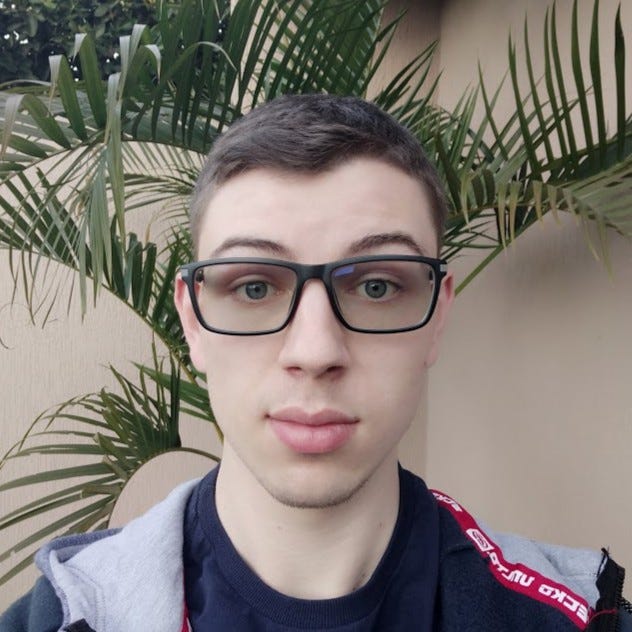
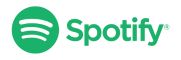