Kotlin and Secure Coding: Best Practices for App Security
In an era where digital threats are constantly evolving, secure coding has become an essential part of application development. Kotlin, with its modern syntax and robust features, provides developers with the tools to build secure and reliable applications. This blog explores the best practices for secure coding in Kotlin, offering practical examples to help developers enhance their app security.
Understanding Secure Coding
Secure coding involves writing software that is free from vulnerabilities, ensuring that the application is protected from potential threats. This practice is crucial in preventing security breaches, data leaks, and other cyber threats that can compromise the integrity and confidentiality of an application.
Best Practices for Secure Coding in Kotlin
Kotlin’s features, like null safety and type inference, contribute to writing more secure code. However, developers must also follow secure coding practices to further enhance the security of their applications. Below are some key practices and code examples demonstrating how to implement them in Kotlin.
1. Input Validation and Sanitization
Validating and sanitizing user input is crucial to prevent attacks like SQL injection and cross-site scripting (XSS). Kotlin provides various ways to handle input validation effectively.
Example: Validating User Input
```kotlin fun isValidEmail(email: String): Boolean { val emailRegex = "^[A-Za-z](.*)([@]{1})(.{1,})(\\.)(.{1,})" return email.matches(emailRegex.toRegex()) } fun main() { val email = "user@example.com" if (isValidEmail(email)) { println("Valid Email") } else { println("Invalid Email") } } ```
2. Secure Data Storage
Sensitive data, such as passwords and API keys, should be stored securely. Kotlin can be used in conjunction with Android’s `EncryptedSharedPreferences` for secure storage.
Example: Storing Data Securely with EncryptedSharedPreferences
```kotlin import android.content.Context import androidx.security.crypto.EncryptedSharedPreferences import androidx.security.crypto.MasterKeys fun getEncryptedSharedPreferences(context: Context): SharedPreferences { val masterKeyAlias = MasterKeys.getOrCreate(MasterKeys.AES256_GCM_SPEC) return EncryptedSharedPreferences.create( "secure_prefs", masterKeyAlias, context, EncryptedSharedPreferences.PrefKeyEncryptionScheme.AES256_SIV, EncryptedSharedPreferences.PrefValueEncryptionScheme.AES256_GCM ) } fun saveSecureData(context: Context, key: String, value: String) { val sharedPreferences = getEncryptedSharedPreferences(context) sharedPreferences.edit().putString(key, value).apply() } ```
3. Handling Authentication and Authorization
Proper handling of authentication and authorization is key to preventing unauthorized access. Kotlin’s integration with Android’s security APIs helps implement robust authentication mechanisms.
Example: Implementing Biometric Authentication
```kotlin import androidx.biometric.BiometricManager import androidx.biometric.BiometricPrompt import androidx.fragment.app.FragmentActivity fun authenticateUser(activity: FragmentActivity) { val biometricManager = BiometricManager.from(activity) if (biometricManager.canAuthenticate() == BiometricManager.BIOMETRIC_SUCCESS) { val promptInfo = BiometricPrompt.PromptInfo.Builder() .setTitle("Biometric Authentication") .setSubtitle("Authenticate using your biometric credential") .setNegativeButtonText("Cancel") .build() val biometricPrompt = BiometricPrompt(activity, activity.mainExecutor, object : BiometricPrompt.AuthenticationCallback() { override fun onAuthenticationSucceeded(result: BiometricPrompt.AuthenticationResult) { println("Authentication succeeded") } override fun onAuthenticationFailed() { println("Authentication failed") } }) biometricPrompt.authenticate(promptInfo) } } ```
4. Avoiding Hardcoding Sensitive Information
Hardcoding sensitive information, such as API keys or passwords, is a common security flaw. Kotlin developers should avoid this practice by using secure storage solutions or environment variables.
Example: Using BuildConfig for API Keys
```kotlin // build.gradle android { defaultConfig { buildConfigField("String", "API_KEY", "\"your_api_key_here\"") } } // Accessing the API key in Kotlin code val apiKey = BuildConfig.API_KEY ```
5. Secure Network Communication
Using secure communication protocols, such as HTTPS, and validating SSL certificates are essential for protecting data in transit. Kotlin’s networking libraries, like OkHttp, make it easy to implement secure network communication.
Example: Enforcing HTTPS with OkHttp
```kotlin import okhttp3.OkHttpClient import okhttp3.Request import java.security.cert.CertificateException import javax.net.ssl.SSLContext import javax.net.ssl.TrustManagerFactory import javax.net.ssl.X509TrustManager fun getSecureOkHttpClient(): OkHttpClient { val trustManagerFactory = TrustManagerFactory.getInstance(TrustManagerFactory.getDefaultAlgorithm()) trustManagerFactory.init(null as KeyStore?) val trustManagers = trustManagerFactory.trustManagers val x509TrustManager = trustManagers[0] as X509TrustManager val sslContext = SSLContext.getInstance("TLS") sslContext.init(null, arrayOf(x509TrustManager), null) return OkHttpClient.Builder() .sslSocketFactory(sslContext.socketFactory, x509TrustManager) .build() } fun main() { val client = getSecureOkHttpClient() val request = Request.Builder() .url("https://secureapi.example.com/data") .build() val response = client.newCall(request).execute() println(response.body?.string()) } ```
Conclusion
Kotlin provides a range of features that, when combined with secure coding practices, can significantly enhance the security of your applications. By following best practices like input validation, secure data storage, proper authentication, and secure network communication, you can protect your apps from potential vulnerabilities. Implementing these practices will lead to more secure and resilient applications in the ever-evolving landscape of cybersecurity.
Further Reading:
Table of Contents
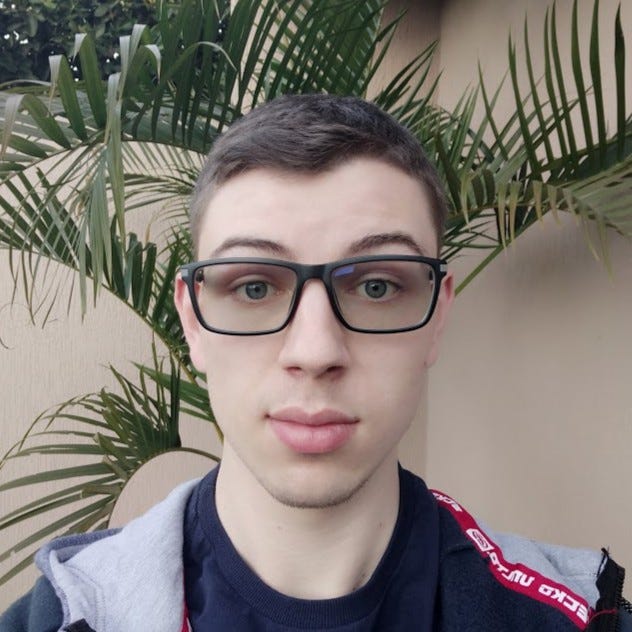
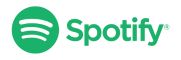