Exploring Kotlin Sequence API: Laziness and Efficiency
In Kotlin, the Sequence API provides a powerful way to handle large collections of data with efficiency and minimal memory usage. Sequences enable lazy evaluation, allowing operations to be performed only when necessary, which can significantly enhance the performance of your applications. This article explores the Kotlin Sequence API, demonstrating its benefits and providing practical examples of how to utilize it for efficient data processing.
Understanding Kotlin Sequences
A sequence in Kotlin is a container for a potentially large or even infinite collection of data that is processed lazily. Unlike collections, sequences perform operations such as filtering, mapping, and more in a way that avoids intermediate results, leading to more efficient code execution.
When you use a sequence, elements are processed one by one as needed, rather than all at once. This lazy processing helps in reducing memory consumption and improving performance, especially when dealing with large datasets.
Using Kotlin Sequences for Efficient Data Processing
Kotlin’s Sequence API is designed for scenarios where you want to perform multiple transformations on a collection of data efficiently. Below are some key aspects and examples of how Kotlin sequences can be leveraged for laziness and efficiency.
1. Creating a Sequence
To begin using sequences, you can easily convert a collection into a sequence using the `asSequence()` function. You can also create sequences from scratch using the `sequence` builder.
Example: Converting a List to a Sequence
```kotlin fun main() { val numbers = listOf(1, 2, 3, 4, 5) val sequence = numbers.asSequence() // Lazily process the sequence val result = sequence.map { it * 2 } .filter { it > 5 } .toList() println(result) // Output: [6, 8, 10] } ```
In this example, the sequence performs the mapping and filtering operations lazily, which means that each element is processed only as needed, and no intermediate collections are created.
2. Generating Sequences
Kotlin allows you to generate sequences using functions like `generateSequence` or through the `sequence` builder. These methods are particularly useful when you need to create sequences from non-collection sources or even infinite sequences.
Example: Generating an Infinite Sequence
```kotlin fun main() { val infiniteSequence = generateSequence(0) { it + 2 } // Take only the first 5 elements val result = infiniteSequence.take(5).toList() println(result) // Output: [0, 2, 4, 6, 8] } ```
This example demonstrates how you can generate an infinite sequence and then use lazy processing to take only a finite number of elements.
3. Combining Multiple Operations
One of the key benefits of sequences is the ability to combine multiple operations without incurring the overhead of intermediate collections.
Example: Combining Mapping and Filtering Operations
```kotlin fun main() { val numbers = listOf(1, 2, 3, 4, 5, 6) val sequence = numbers.asSequence() val result = sequence.filter { it % 2 == 0 } .map { it * it } .toList() println(result) // Output: [4, 16, 36] } ```
Here, the filtering and mapping operations are combined and processed lazily, resulting in an efficient and concise code that processes only the necessary elements.
4. Exploring Sequence Operations and Performance
Sequences can significantly improve performance, especially when dealing with large datasets or chains of operations. The main advantage comes from the fact that operations are performed lazily, meaning that elements are processed as they are needed.
Example: Comparing Performance of Sequences and Collections
```kotlin fun main() { val largeList = (1..1_000_000).toList() val startTimeList = System.currentTimeMillis() val listResult = largeList.filter { it % 2 == 0 } .map { it * 2 } .take(100) .toList() val endTimeList = System.currentTimeMillis() println("List processing time: ${endTimeList - startTimeList} ms") val startTimeSeq = System.currentTimeMillis() val sequenceResult = largeList.asSequence() .filter { it % 2 == 0 } .map { it * 2 } .take(100) .toList() val endTimeSeq = System.currentTimeMillis() println("Sequence processing time: ${endTimeSeq - startTimeSeq} ms") } ```
This example highlights how sequences can offer performance improvements by avoiding the creation of intermediate collections, especially in cases where the data set is large, and only a subset of the data is required.
Conclusion
Kotlin’s Sequence API is a powerful tool for writing efficient, concise, and memory-friendly code. By leveraging lazy evaluation, sequences help in reducing unnecessary computations and memory overhead, making them an excellent choice for processing large or complex datasets. Understanding how to effectively use sequences will enable you to write more optimized Kotlin code.
Further Reading
Table of Contents
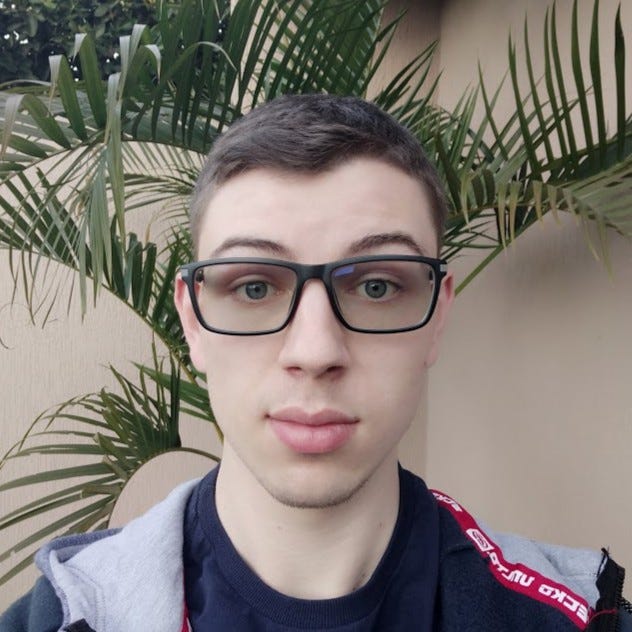
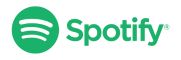