Exploring Kotlin Serialization with JSON: Handling Complex Data
Kotlin is a modern language known for its concise syntax and powerful features. One of its standout capabilities is Kotlin Serialization, which simplifies JSON data handling. This blog explores how Kotlin Serialization can be used to manage and manipulate complex JSON data effectively, offering practical examples to enhance your data handling skills.
Understanding Kotlin Serialization
Kotlin Serialization is a library that provides a way to serialize and deserialize Kotlin objects to and from various formats, including JSON. It offers a type-safe approach to working with data, ensuring that your data transformations are robust and less error-prone.
Using Kotlin Serialization for JSON Data Handling
Kotlin Serialization makes it easy to convert complex JSON data into Kotlin objects and vice versa. Below, we’ll look at key aspects and examples to help you master JSON data handling with Kotlin.
1. Setting Up Kotlin Serialization
First, you need to include the Kotlin Serialization library in your project. You can do this by adding the necessary dependencies to your `build.gradle` file.
```groovy plugins { id 'org.jetbrains.kotlin.plugin.serialization' version '1.8.10' } dependencies { implementation "org.jetbrains.kotlinx:kotlinx-serialization-json:1.5.0" } ```
2. Serializing and Deserializing Simple JSON Data
Here’s a basic example of how to serialize and deserialize simple JSON data using Kotlin Serialization.
Example: Serializing a Kotlin Data Class
```kotlin import kotlinx.serialization.* import kotlinx.serialization.json.* @Serializable data class User(val name: String, val age: Int) fun main() { val user = User("Alice", 30) val jsonString = Json.encodeToString(user) println(jsonString) // Output: {"name":"Alice","age":30} val deserializedUser = Json.decodeFromString<User>(jsonString) println(deserializedUser) // Output: User(name=Alice, age=30) } ```
3. Handling Complex JSON Structures
Complex JSON structures, such as nested objects and lists, can also be handled efficiently with Kotlin Serialization.
Example: Serializing and Deserializing Nested JSON Data
```kotlin import kotlinx.serialization.* import kotlinx.serialization.json.* @Serializable data class Address(val city: String, val zipCode: String) @Serializable data class Person(val name: String, val address: Address) fun main() { val person = Person("Bob", Address("New York", "10001")) val jsonString = Json.encodeToString(person) println(jsonString) // Output: {"name":"Bob","address":{"city":"New York","zipCode":"10001"}} val deserializedPerson = Json.decodeFromString<Person>(jsonString) println(deserializedPerson) // Output: Person(name=Bob, address=Address(city=New York, zipCode=10001)) } ```
4. Custom Serialization and Deserialization
Sometimes, you may need custom serialization logic to handle specific cases. Kotlin Serialization allows you to define custom serializers.
Example: Custom Serializer for a Date
```kotlin import kotlinx.serialization.* import kotlinx.serialization.json.* import java.time.LocalDate import java.time.format.DateTimeFormatter object LocalDateSerializer : KSerializer<LocalDate> { private val formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd") override val descriptor: SerialDescriptor = PrimitiveSerialDescriptor("LocalDate", PrimitiveKind.STRING) override fun serialize(encoder: Encoder, value: LocalDate) { encoder.encodeString(formatter.format(value)) } override fun deserialize(decoder: Decoder): LocalDate { return LocalDate.parse(decoder.decodeString(), formatter) } } @Serializable data class Event(val name: String, @Serializable(with = LocalDateSerializer::class) val date: LocalDate) fun main() { val event = Event("Conference", LocalDate.of(2024, 8, 8)) val jsonString = Json.encodeToString(event) println(jsonString) // Output: {"name":"Conference","date":"2024-08-08"} val deserializedEvent = Json.decodeFromString<Event>(jsonString) println(deserializedEvent) // Output: Event(name=Conference, date=2024-08-08) } ```
5. Integrating with APIs
Kotlin Serialization is also useful when working with JSON data from APIs. You can easily fetch and process JSON responses using Kotlin.
Example: Fetching Data from a REST API
```kotlin import kotlinx.serialization.* import kotlinx.serialization.json.* import kotlinx.coroutines.* import java.net.URL @Serializable data class ApiResponse(val data: List<String>) suspend fun fetchApiData(): ApiResponse { val json = withContext(Dispatchers.IO) { URL("https://api.example.com/data").readText() } return Json.decodeFromString(json) } fun main() = runBlocking { val apiResponse = fetchApiData() println(apiResponse) } ```
Conclusion
Kotlin Serialization offers a powerful and flexible approach to handling JSON data. From basic serialization and deserialization to managing complex data structures and custom serializers, Kotlin Serialization equips you with the tools needed for effective JSON data manipulation. Mastering these techniques will enhance your ability to work with data in a variety of applications.
Further Reading:
Table of Contents
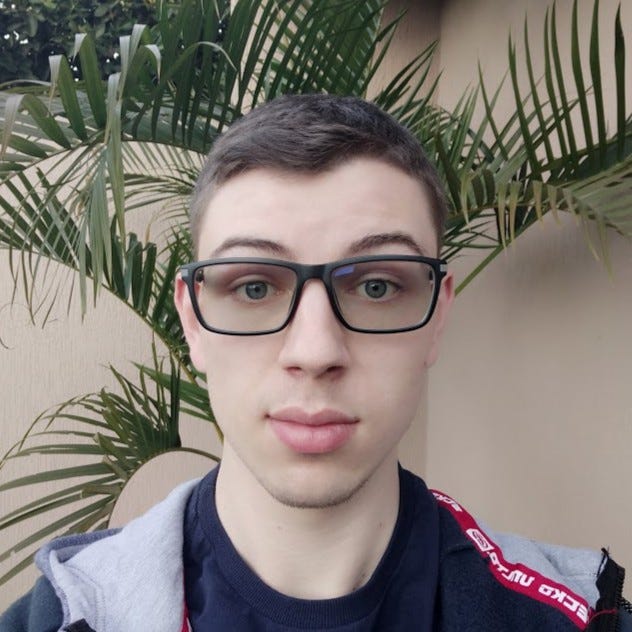
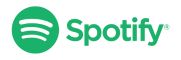