Kotlin for Server-Side Development: Building APIs with Ktor
In the world of server-side development, Kotlin has rapidly gained popularity due to its expressive syntax, safety features, and seamless integration with Java libraries. One of the most compelling frameworks for building APIs with Kotlin is Ktor. Whether you are a seasoned backend developer or just starting your journey into server-side programming, Ktor provides an excellent platform to create efficient and scalable APIs. In this blog post, we’ll explore the fundamentals of Kotlin for server-side development and dive deep into the process of building APIs using Ktor.
1. Why Choose Kotlin for Server-Side Development?
1.1. Concise and Expressive Syntax
Kotlin’s concise syntax allows developers to express complex ideas in a clean and readable manner. Features like extension functions, data classes, and lambda expressions enable concise code, reducing boilerplate and enhancing productivity.
kotlin // Kotlin data class example data class User(val id: Int, val name: String, val email: String) // Extension function fun String.isEmailValid(): Boolean { return this.contains("@") }
1.2. Interoperability with Java
Being fully interoperable with Java, Kotlin allows developers to leverage existing Java libraries effortlessly. This feature is crucial for server-side development as it enables the reuse of well-established Java components and tools.
1.3. Null Safety
Kotlin’s null safety system helps to eliminate NullPointerExceptions (NPE) at compile-time. By distinguishing nullable and non-nullable types, Kotlin ensures safer code execution and reduces the number of runtime crashes.
kotlin fun findUserById(id: Int): User? { // Some logic to find the user return user // Nullable User } // Safe call with ?. operator val user = findUserById(42) val userEmail = user?.email // userEmail is nullable
1.4. Coroutines for Asynchronous Programming
Kotlin’s built-in support for coroutines simplifies asynchronous programming on the server-side. It allows for efficient handling of concurrent operations without the complexity of nested callbacks.
kotlin suspend fun fetchUserData(userId: Int): User { return withContext(Dispatchers.IO) { // Perform asynchronous operations // and return the User object } }
2. An Introduction to Ktor
Ktor is an asynchronous web framework built using Kotlin. It is lightweight, easy to set up, and has excellent performance. Ktor embraces Kotlin’s coroutines, making it a natural fit for building modern, asynchronous APIs.
2.1. Setting Up a Ktor Project
Before diving into building APIs with Ktor, let’s set up a new project.
Step 1: Install IntelliJ IDEA or any other preferred IDE for Kotlin development.
Step 2: Create a new Kotlin project and select the “Ktor” template.
Step 3: Follow the project setup wizard to configure the project.
2.2. Building Your First Ktor API
Now that we have our Ktor project set up, let’s create a simple API with a few endpoints:
2.2.1. Hello World API
kotlin import io.ktor.application.* import io.ktor.response.* import io.ktor.routing.* import io.ktor.server.engine.* import io.ktor.server.netty.* fun Application.module() { routing { get("/") { call.respondText("Hello, World!") } } } fun main() { embeddedServer(Netty, port = 8080, module = Application::module).start(wait = true) }
In this example, we import the necessary Ktor components and define a simple API with one GET endpoint (“/”) that responds with “Hello, World!”.
2.2.2. Parameterized API
kotlin fun Application.module() { routing { get("/greet/{name}") { val name = call.parameters["name"] call.respondText("Hello, $name!") } } }
This API takes a parameter from the URL and returns a personalized greeting. For example, accessing “/greet/John” will result in “Hello, John!”.
3. Routing and Handling Requests
In Ktor, routing is the process of defining which code block should be executed for specific HTTP requests. The routing function defines a route table, where we can specify different routes and their corresponding handlers.
kotlin fun Application.module() { routing { // Define your routes here } }
To handle requests, we use functions like get, post, put, etc., corresponding to the respective HTTP methods. Within these functions, we define the response to be sent back to the client.
4. Handling POST Requests
kotlin fun Application.module() { routing { post("/createUser") { val user = call.receive<User>() // Process and store the user data call.respond(HttpStatusCode.Created, "User created successfully") } } }
In this example, we define a POST endpoint to create a new user. The call.receive<User>() function extracts the incoming JSON data and converts it into a User object, allowing us to process and store the user data.
5. Database Interaction
To build real-world APIs, interaction with databases is essential. Ktor provides flexibility in choosing the database library you prefer. For instance, you can use Exposed for SQL-based databases or MongoDB for NoSQL databases.
6. Using Exposed for SQL-based Databases
kotlin // Add Exposed dependency to your build.gradle dependencies { implementation "org.jetbrains.exposed:exposed-core:$exposed_version" implementation "org.jetbrains.exposed:exposed-dao:$exposed_version" implementation "org.jetbrains.exposed:exposed-jdbc:$exposed_version" implementation "org.jetbrains.exposed:exposed-java-time:$exposed_version" } // Define a users table object Users : IntIdTable() { val name = varchar("name", 255) val email = varchar("email", 255).uniqueIndex() } // Query data from the table fun getAllUsers(): List<User> { return transaction { Users.selectAll().map { row -> User( id = row[Users.id].value, name = row[Users.name], email = row[Users.email] ) } } }
In this example, we set up a simple users table and query all users from the database using Exposed.
7. Error Handling
Effective error handling is crucial for any API to provide meaningful responses to clients when things go wrong. Ktor provides a mechanism to handle errors gracefully.
kotlin fun Application.module() { install(StatusPages) { exception<NotFoundException> { cause -> call.respond(HttpStatusCode.NotFound, "Resource not found") } exception<ValidationException> { cause -> call.respond(HttpStatusCode.BadRequest, cause.message ?: "Invalid request") } exception<Throwable> { cause -> call.respond(HttpStatusCode.InternalServerError, "Something went wrong") } } }
In this example, we use Ktor’s StatusPages feature to handle specific exceptions and return appropriate HTTP status codes and error messages.
Conclusion
Kotlin, with its expressive syntax and powerful features, is an excellent choice for server-side development. When combined with the lightweight and efficient Ktor framework, building APIs becomes a delightful experience. In this blog post, we’ve only scratched the surface of what Kotlin and Ktor can offer for server-side development. There’s a vast ecosystem of libraries and tools waiting to be explored. So, if you’re ready to embark on your journey into server-side development, don’t hesitate to embrace the elegance of Kotlin and the productivity of Ktor.
Happy coding!
Table of Contents
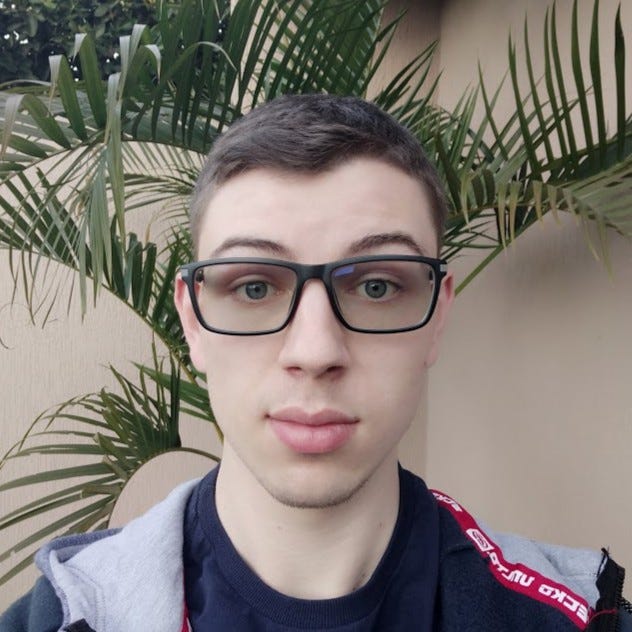
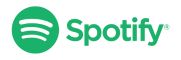