Kotlin Uncovered: Simplifying Domain-Specific Language Design with Practical Examples
Kotlin has emerged as a favored language for many developers, as well as businesses looking to hire Kotlin developers, due to its simplicity, conciseness, and interoperability with Java. Among its numerous powerful features, one less-discussed yet significantly impactful feature is Kotlin’s ability to define Domain-Specific Languages (DSLs). Kotlin DSLs are a method for creating APIs that offer ease in reading and writing, whilst encapsulating intricate logic. If you’re a business seeking to hire Kotlin developers or an individual interested in expanding your knowledge, this post is for you. We’ll delve into the nitty-gritty of DSLs, explore how to design them in Kotlin, and examine a few tangible examples.
What is a DSL?
A Domain-Specific Language (DSL) is a computer language specialized to a particular application domain. This is in contrast to a General-Purpose Language (GPL), which is designed for a broad range of application domains. DSLs offer a way of writing software that is focused on a specific problem domain, making the code easier to understand and manage.
Why Kotlin for DSL?
Kotlin provides several features that make it a good choice for designing DSLs:
- Type-safe Builders: This is a Kotlin feature used to create complex hierarchical data structures in a semi-declarative way.
- Extension Functions: Kotlin allows you to extend a class with new functionality without inheriting from the class.
- Lambdas with Receiver: Lambdas with receiver are essentially functions that can be invoked on an object, giving the ability to access this object inside the function.
Kotlin DSL Example 1: A Simple HTML Builder
Let’s start with a simple DSL to create HTML documents. This DSL uses type-safe builders and extension functions to create a clean, easy-to-use API.
```kotlin fun html(block: HTML.() -> Unit): HTML = HTML().apply(block) class HTML { fun body(block: Body.() -> Unit) { tag("body", block) } private fun <T : Tag> tag(name: String, block: T.() -> Unit): T { return T(name).apply(block) } } open class Tag(val name: String) { fun String.attr(value: String) { println("$this=$value") } fun text(value: String) { println(value) } } class Body : Tag("body") ```
This DSL can be used to create HTML documents in the following way:
```kotlin html { body { "id".attr("main") text("Hello, Kotlin DSL!") } } ```
This DSL is readable, and the usage is clear and concise.
Kotlin DSL Example 2: Building a Configuration DSL
Another common use case for DSLs is for configuration. A configuration DSL can make complex configurations simple and readable. Let’s create a simple configuration DSL for a server:
```kotlin data class Server(val name: String, var port: Int = 8080, var isHttps: Boolean = false) fun server(name: String, block: Server.() -> Unit) = Server(name).apply(block) fun Server.port(block: () -> Int) { port = block() } fun Server.isHttps(block: () -> Boolean) { isHttps = block() } ```
This DSL can be used to configure a server as follows:
```kotlin val myServer = server("myServer") { port { 8000 } isHttps { true } } ```
This DSL is type-safe, meaning that if you try to set the port to a non-integer or isHttps to a non-boolean, the Kotlin compiler will throw an error.
Kotlin DSL Example 3: A DSL for SQL Query
DSLs can also be used to encapsulate complex logic
and make it easier to read and write. Let’s take a look at a DSL for constructing SQL queries:
```kotlin data class Query(val table: String) { var where: String = "" infix fun WHERE(condition: String) { where = condition } override fun toString(): String { return "SELECT * FROM $table WHERE $where" } } fun query(table: String, block: Query.() -> Unit) = Query(table).apply(block) ```
This DSL can be used to construct SQL queries in the following way:
```kotlin val q = query("users") { WHERE ("id = 1") } println(q) // prints: SELECT * FROM users WHERE id = 1 ```
This DSL is a simple example but could be expanded to support more SQL syntax, like JOINs and ORDER BYs.
Conclusion
Kotlin DSLs are a powerful tool that can make code easier to read and write, encapsulate complex logic, and provide type-safe APIs. This level of sophistication makes hiring Kotlin developers a valuable decision for businesses aiming for robust, maintainable code. The examples provided, though simple, effectively illustrate the power and flexibility of Kotlin DSLs. If you haven’t yet explored DSLs in Kotlin, there’s no better time than now. With the right guidance, perhaps from expert Kotlin developers, you’ll soon be creating powerful, easy-to-use APIs that streamline your development process.
Table of Contents
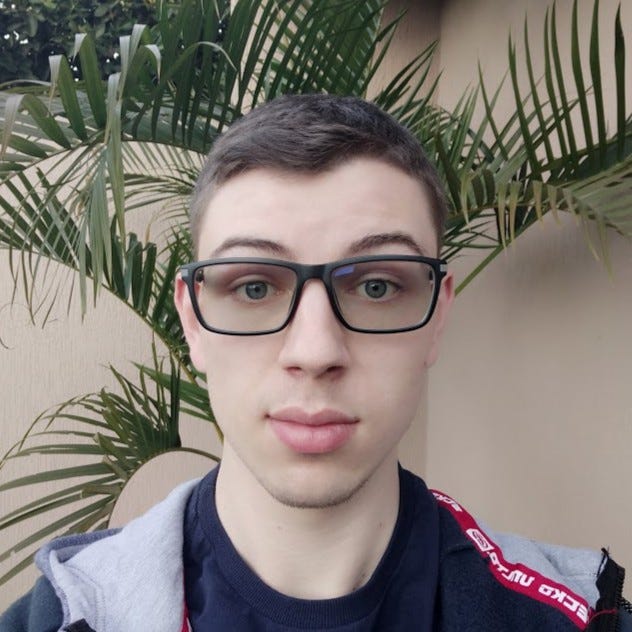
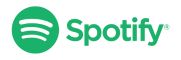