What is smart casting in Kotlin?
Smart casting is a convenient feature in Kotlin that allows the compiler to automatically cast variables within certain scopes based on type checks performed in the code. It eliminates the need for explicit type casting, making code cleaner and more concise.
In Kotlin, smart casting applies primarily to immutable local variables and properties that are checked for type compatibility using is or !is operators. When the compiler detects that a variable has been checked for a specific type, it automatically casts the variable to that type within the scope where the check is performed.
For example:
kotlin fun processValue(value: Any) { if (value is String) { println(value.length) // value is automatically cast to String } }
In this example, value is smart cast to String within the scope of the if statement after the type check.
Smart casting simplifies code and reduces verbosity by eliminating the need for explicit type checks and casts. It enhances code readability and maintainability by reducing boilerplate code associated with type conversions.
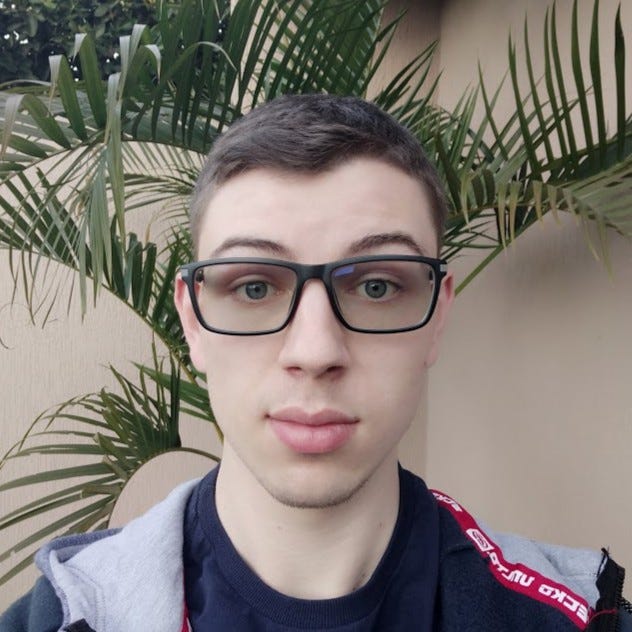
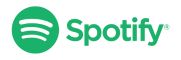