Exploring Kotlin Standard Library: A Treasure Trove of Useful Functions
Kotlin has gained immense popularity among developers due to its concise syntax, null safety, and seamless interoperability with Java. One of the key strengths of Kotlin is its powerful Standard Library. Packed with a wide range of functions and extension methods, the Kotlin Standard Library offers a treasure trove of utilities to simplify common programming tasks. In this blog, we will embark on a journey to explore the Kotlin Standard Library and discover some of its most useful functions. Whether you are a beginner or an experienced Kotlin developer, this guide will provide insights and code samples to enhance your productivity.
1. What is the Kotlin Standard Library?
The Kotlin Standard Library is a comprehensive collection of classes and functions that ship with the Kotlin programming language. It provides a rich set of APIs that complement the language’s features and facilitate common programming tasks. The library is designed to be concise, expressive, and idiomatic, making it a valuable asset for Kotlin developers.
2. Working with Collections
Collections are an essential part of any programming language, and the Kotlin Standard Library provides a wide range of functions to manipulate them efficiently.
2.1. Filtering Collections
Filtering a collection based on specific criteria is a common requirement. The Kotlin Standard Library offers functions like filter(), filterNot(), and filterIndexed() to make this task effortless. Here’s an example:
kotlin val numbers = listOf(1, 2, 3, 4, 5) val evenNumbers = numbers.filter { it % 2 == 0 } println(evenNumbers) // Output: [2, 4]
2.2. Transforming Collections
Transforming elements of a collection is another frequently performed operation. The Standard Library provides functions like map(), flatMap(), and distinct() to achieve this. Consider the following example:
kotlin val names = listOf("Alice", "Bob", "Charlie") val nameLengths = names.map { it.length } println(nameLengths) // Output: [5, 3, 7]
2.3. Grouping and Partitioning Collections
Grouping and partitioning collections based on certain properties can be done easily using the groupBy() and partition() functions. Let’s see an example:
kotlin val words = listOf("apple", "banana", "cherry", "date", "elderberry") val groupedWords = words.groupBy { it.length } println(groupedWords) // Output: {5=[apple, cherry], 6=[banana, elderberry], 4=[date]}
3. String Manipulation
Strings are a fundamental part of any programming language, and Kotlin provides several functions in its Standard Library to work with them effectively.
3.1. String Interpolation
String interpolation allows you to embed expressions and variables directly within a string. Kotlin supports string interpolation using the ${} syntax. Here’s an example:
kotlin val name = "John" val greeting = "Hello, $name!" println(greeting) // Output: Hello, John!
3.2. String Formatting
The Standard Library includes the format() function, which allows you to format strings in a flexible and convenient way. Consider the following example:
kotlin val pi = 3.14159 val formatted = "The value of pi is approximately %.2f".format(pi) println(formatted) // Output: The value of pi is approximately 3.14
3.3. Splitting and Joining Strings
Splitting and joining strings are common operations, and Kotlin provides functions like split() and joinToString() to make them easy. Here’s an example:
kotlin val sentence = "Hello, World!" val words = sentence.split(" ") println(words) // Output: [Hello,, World!] val fruits = listOf("apple", "banana", "cherry") val joinedFruits = fruits.joinToString() println(joinedFruits) // Output: apple, banana, cherry
4. Working with Files
File operations are an integral part of many applications, and the Kotlin Standard Library provides convenient functions for working with files.
4.1. Reading and Writing Files
Reading the contents of a file or writing data to a file can be done using functions like readText() and writeText(). Here’s an example:
kotlin val file = File("example.txt") val content = file.readText() println(content) file.writeText("Hello, World!")
4.2. File Manipulation
The Standard Library also offers various functions for file manipulation, such as copyTo(), moveTo(), and delete(). Let’s look at an example:
kotlin val sourceFile = File("source.txt") val destinationFile = File("destination.txt") sourceFile.copyTo(destinationFile, overwrite = true) sourceFile.delete()
5. Concurrency and Coroutines
Kotlin provides excellent support for concurrency and asynchronous programming through its coroutines feature. The Standard Library offers functions and utilities to work with coroutines effectively.
5.1. Launching Coroutines
The launch() function is a convenient way to start a new coroutine. Here’s an example:
kotlin GlobalScope.launch { // Coroutine code here }
5.2. Synchronization and Mutex
The Standard Library provides synchronization mechanisms like Mutex to protect shared resources accessed by multiple coroutines. Consider the following example:
kotlin val mutex = Mutex() var count = 0 suspend fun increment() { mutex.withLock { count++ } }
6. Mathematical Operations
The Kotlin Standard Library includes functions and classes to perform various mathematical operations efficiently.
6.1. Random Numbers
Generating random numbers can be achieved using the Random class from the Standard Library. Here’s an example:
kotlin val random = Random() val randomNumber = random.nextInt(10) println(randomNumber) // Output: Random number between 0 and 9
6.2. Ranges and Progressions
Ranges and progressions simplify working with sequences of numbers. The Standard Library provides operators and functions like rangeTo() and step() for this purpose. Let’s see an example:
kotlin val range = 1..10 val evenNumbers = range.step(2) println(evenNumbers) // Output: [2, 4, 6, 8, 10]
7. Date and Time
Working with dates and times is a common task in many applications. The Kotlin Standard Library offers classes and functions to handle date and time operations efficiently.
7.1. Date Manipulation
The Date class in the Standard Library allows you to perform various date manipulation operations, such as adding or subtracting days. Here’s an example:
kotlin val now = LocalDate.now() val tomorrow = now.plusDays(1) println(tomorrow) // Output: Tomorrow's date
7.2. Formatting and Parsing Dates
The Standard Library provides the SimpleDateFormat class for formatting and parsing dates. Here’s an example:
kotlin val formatter = SimpleDateFormat("dd-MM-yyyy") val formattedDate = formatter.format(Date()) println(formattedDate) // Output: Current date in dd-MM-yyyy format
Conclusion
The Kotlin Standard Library is a powerful asset for Kotlin developers. Its extensive collection of functions and classes simplifies common programming tasks, enhances productivity, and promotes idiomatic Kotlin code. In this blog, we explored various aspects of the Standard Library, from collections and strings to files and dates. Armed with this knowledge, you can unlock the full potential of Kotlin and streamline your development process. Start leveraging the Kotlin Standard Library today and witness its impact on your code!
Table of Contents
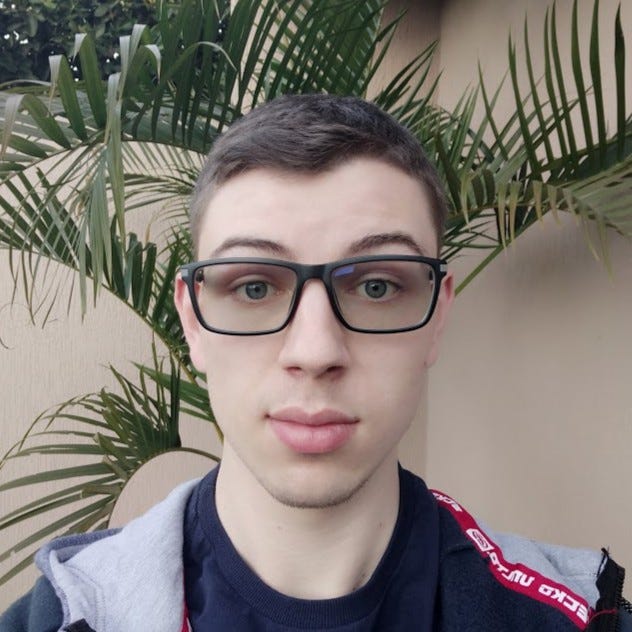
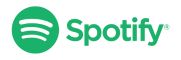