Kotlin Q & A
How do you define a tail-recursive function in Kotlin?
To define a tail-recursive function in Kotlin, you use the tailrec modifier before the function declaration. This modifier informs the Kotlin compiler that the function is tail-recursive and eligible for optimization.
Here’s the general syntax for defining a tail-recursive function in Kotlin:
kotlin tailrec fun functionName(parameters): ReturnType { // Base case if (condition) { return baseCase } // Recursive case return functionName(modifiedParameters) }
In this syntax:
- tailrec is the modifier that indicates the function is tail-recursive.
- functionName is the name of the tail-recursive function.
- parameters are the input parameters of the function.
- ReturnType is the return type of the function.
- The function body contains the base case and recursive case of the tail-recursive algorithm.
It’s important to note that for a function to be tail-recursive, the recursive call must be the last operation performed by the function before returning its result. If the function performs any additional operations after the recursive call, the Kotlin compiler will not optimize it as a tail-recursive function.
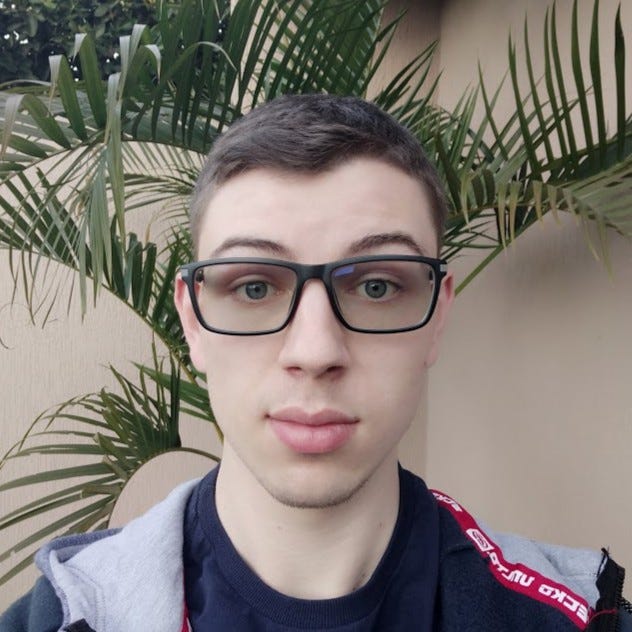
Previously at
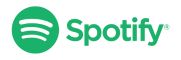
Experienced Android Engineer specializing in Kotlin with over 5 years of hands-on expertise. Proven record of delivering impactful solutions and driving app innovation.