Kotlin and TensorFlow on Android: The New Frontier in Mobile ML
In recent years, mobile devices have become powerful enough to execute complex tasks that were traditionally reserved for high-end servers or desktop computers. Machine Learning (ML), a field that involves training models to make predictions based on data, is no exception. With TensorFlow, a popular ML framework by Google, it is now possible to run ML models directly on Android devices.
Kotlin, the modern statically-typed language officially supported for Android development, is well-suited for integrating TensorFlow functionalities, thanks to its concise syntax and interoperability with Java libraries.
In this post, we’ll explore how Kotlin and TensorFlow come together for ML on Android. We’ll walk through examples, and by the end, you’ll have a clear idea of how to get started with your own ML-infused Android app.
1. Setting Up the Environment
Before you start, ensure you have Android Studio installed and the TensorFlow Lite Android library added to your project. You can add the library by inserting the following dependency into your app’s `build.gradle` file:
```kotlin implementation 'org.tensorflow:tensorflow-lite:0.0.0' // Replace '0.0.0' with the latest version number. ```
2. Converting TensorFlow Models to TensorFlow Lite
TensorFlow models (usually with a `.pb` extension) need to be converted to TensorFlow Lite format (`.tflite`) for use on Android. TensorFlow Lite models are optimized for mobile and other edge devices.
To do this, use TensorFlow’s TFLiteConverter:
```python import tensorflow as tf model = tf.keras.models.load_model('path_to_your_model.pb') converter = tf.lite.TFLiteConverter.from_keras_model(model) tflite_model = converter.convert() with open('model.tflite', 'wb') as f: f.write(tflite_model) ```
After running this script, you should see a `model.tflite` file, ready for use in your Android project.
3. Loading the Model on Android
Once you have your `.tflite` model, add it to the `assets` folder of your Android project. Then, you can load it using TensorFlow Lite’s Interpreter class:
```kotlin val assetManager = context.assets val modelFileName = "model.tflite" val fileDescriptor = assetManager.openFd(modelFileName) val inputStream = FileInputStream(fileDescriptor.fileDescriptor) val fileChannel = inputStream.channel val startOffset = fileDescriptor.startOffset val declaredLength = fileDescriptor.declaredLength val modelByteBuffer = fileChannel.map(FileChannel.MapMode.READ_ONLY, startOffset, declaredLength) val interpreter = Interpreter(modelByteBuffer) ```
4. Making Predictions
Now that the model is loaded, you can use it to make predictions. Let’s say you want to classify an image:
```kotlin val bitmap = ... // Load your image as a Bitmap val byteBuffer = convertBitmapToByteBuffer(bitmap) val result = Array(1) { FloatArray(numOfLabels) } // `numOfLabels` is the number of classes your model can predict. interpreter.run(byteBuffer, result) val predictedLabel = result[0].indexOf(result[0].maxOrNull()!!) ```
The function `convertBitmapToByteBuffer` is a utility function that you’d write to convert your Bitmap image into a ByteBuffer, which is the input format expected by the interpreter.
5. Optimizing Performance
For better performance, especially on devices with multi-core CPUs, you can set the number of threads used by the interpreter:
```kotlin interpreter.options.setNumThreads(4) // Adjust the number based on the device's capabilities ```
6. Examples
– Image Classification: Use a pre-trained model, like MobileNet, to classify objects in pictures. Users could snap a photo or select one from their gallery, and the app will identify the object.
– Text Generation: Train a model on text data, and allow users to generate new, similar text. For instance, train a model on Shakespeare’s works, and users can generate “Shakespearean-style” sentences.
– Voice Commands: Use a model trained on audio data to recognize voice commands. Implement features where users can control the app using their voice.
Conclusion
Machine learning on Android, powered by Kotlin and TensorFlow, unlocks a plethora of opportunities for developers. With the ability to run complex models directly on the device, apps can provide smarter, more personalized experiences to users. From image recognition to natural language processing, the possibilities are vast and exciting.
As with any development endeavor, remember to continuously test and optimize your ML models for the best performance and user experience on Android devices. Happy coding!
Table of Contents
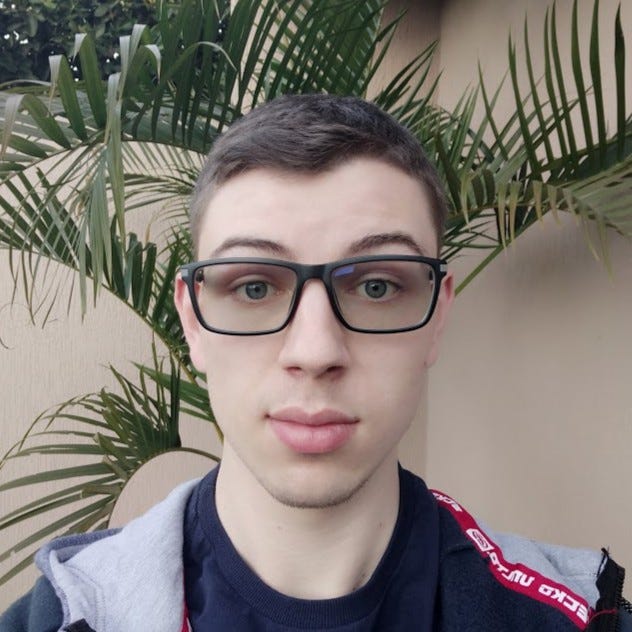
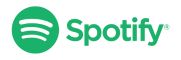