Kotlin and TensorFlow Lite: Machine Learning on Mobile Devices
Machine learning is transforming mobile applications, enabling them to perform sophisticated tasks such as image recognition, language translation, and predictive analytics. TensorFlow Lite (TFLite) is a lightweight version of TensorFlow designed specifically for mobile and edge devices, and when paired with Kotlin, it offers a powerful toolkit for developing advanced machine learning models on Android.
This blog explores how Kotlin and TensorFlow Lite can be used together to implement machine learning capabilities in mobile applications, providing practical examples and code snippets to guide you through the process.
Understanding TensorFlow Lite
TensorFlow Lite is a framework optimized for mobile and embedded devices. It provides tools for converting and deploying TensorFlow models on Android and iOS devices, offering reduced latency and lower power consumption compared to running full TensorFlow models.
Setting Up TensorFlow Lite with Kotlin
Integrating TensorFlow Lite into an Android project using Kotlin involves several steps, including adding dependencies, preparing your model, and writing code to run inference.
1. Adding TensorFlow Lite Dependencies
To get started, you need to add TensorFlow Lite dependencies to your Android project’s `build.gradle` file.
```gradle dependencies { implementation 'org.tensorflow:tensorflow-lite:2.11.0' implementation 'org.tensorflow:tensorflow-lite-support:0.4.0' } ```
2. Converting and Loading a TensorFlow Model
Before you can use a TensorFlow model in your Kotlin app, you need to convert it to the TensorFlow Lite format. Use the TensorFlow Lite Converter to convert your model and include the resulting `.tflite` file in your Android project’s `assets` folder.
3. Running Inference with TensorFlow Lite in Kotlin
Once you have the `.tflite` model file, you can use TensorFlow Lite’s Interpreter API to run inference. Below is an example of how to load and use a TensorFlow Lite model in Kotlin.
Example: Running Inference with a Pretrained Model
```kotlin import android.content.Context import org.tensorflow.lite.Interpreter import org.tensorflow.lite.support.common.FileUtil import org.tensorflow.lite.support.tensorbuffer.TensorBuffer class ModelInference(context: Context) { private val interpreter: Interpreter init { // Load the TensorFlow Lite model val model = FileUtil.loadMappedFile(context, "model.tflite") interpreter = Interpreter(model) } fun predict(input: FloatArray): FloatArray { // Prepare input and output buffers val inputTensor = TensorBuffer.createFixedSize(intArrayOf(1, input.size), DataType.FLOAT32) inputTensor.loadArray(input) val outputTensor = TensorBuffer.createFixedSize(intArrayOf(1, 10), DataType.FLOAT32) // Run inference interpreter.run(inputTensor.buffer, outputTensor.buffer.rewind()) return outputTensor.floatArray } } ```
4. Enhancing Mobile Applications with Machine Learning
With TensorFlow Lite, you can add various machine learning functionalities to your app, such as:
– Image Classification: Analyze images captured by the camera or gallery to recognize objects or scenes.
– Text Classification: Classify user input or analyze sentiment.
– Object Detection: Detect and localize objects within an image.
Example: Image Classification with TensorFlow Lite
To implement image classification, you would typically preprocess the image data, run inference, and interpret the results.
```kotlin import android.graphics.Bitmap import org.tensorflow.lite.support.image.ImageProcessor import org.tensorflow.lite.support.image.TensorImage fun classifyImage(bitmap: Bitmap): FloatArray { // Preprocess the image val imageProcessor = ImageProcessor.Builder() .add(ResizeOp(224, 224, ResizeOp.ResizeMethod.BILINEAR)) .build() val tensorImage = TensorImage.fromBitmap(bitmap) val processedImage = imageProcessor.process(tensorImage) // Run inference return modelInference.predict(processedImage.floatArray) } ```
Conclusion
Integrating TensorFlow Lite with Kotlin enables you to build sophisticated machine learning applications on mobile devices, providing enhanced functionalities and user experiences. By leveraging Kotlin’s concise syntax and TensorFlow Lite’s efficient model deployment, you can develop powerful mobile apps that perform real-time predictions and analyses.
Further Reading:
Table of Contents
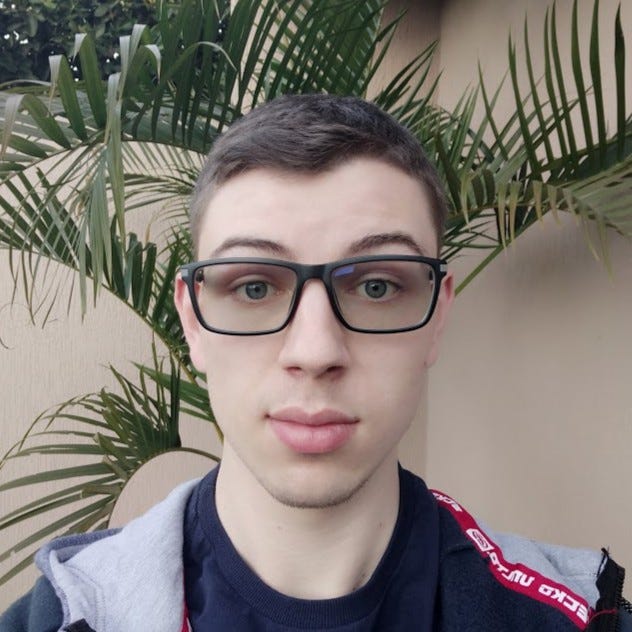
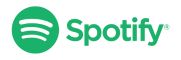