Java vs Kotlin: A Comparative Analysis for Android Development
In the realm of Android app development, the longstanding king, Java, has found a worthy adversary in the form of Kotlin. Introduced by JetBrains in 2011, Kotlin has been gaining significant popularity, leading to an increase in demand to hire Kotlin developers. This language gained official recognition from Google in 2017, allowing Android developers to write their applications in a language other than Java, marking a pivotal moment in Android development history.
The arrival of Kotlin sparked a debate: which language should you choose for Android development, Kotlin or Java? The rising trend to hire Kotlin developers indicates its growing importance in the Android development landscape. However, is it the right choice for you or your project? Let’s delve into the key differences between the two languages, discuss their benefits and drawbacks, and provide some examples to help guide your decision-making process.
History and Popularity
Java has been a widely used language for over two decades. It gained prominence in the Android community as Google selected it as the official language for Android app development. Over the years, developers worldwide have used Java to build numerous feature-rich, reliable Android applications.
Kotlin, though relatively newer, is not a language to overlook. Its growth rate since gaining Google’s endorsement in 2017 has been nothing short of impressive. Kotlin’s enhanced efficiency and developer-friendly features have made it a go-to choice for many Android developers.
Syntax and Learning Curve
Java
Java is a class-based, object-oriented programming language with a syntax similar to C and C++. While this syntax is straightforward for developers with a background in these languages, beginners may find it challenging to grasp. Consider the following example of a simple “Hello, World!” program:
```java public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World!"); } } ```
Java requires a class definition, even for such a simple program. This verbosity might be a hurdle for beginners.
Kotlin
Kotlin, on the other hand, was designed to be an improvement on Java, maintaining interoperability with Java and the JVM. Kotlin’s syntax is simpler and more concise than Java’s. It removes much of the verbosity and redundancy that developers often critique in Java. Here’s how you write the same “Hello, World!” program in Kotlin:
```kotlin fun main(args: Array<String>) { println("Hello, World!") } ```
Kotlin eliminates the need for a class definition for such a simple program, making it easier for beginners to understand.
Null Safety
Java
One of the most common issues in Java is the dreaded NullPointerException. Java allows objects to be assigned null values, which, when accessed, can cause a system crash.
```java String str = null; int len = str.length(); // This will throw a NullPointerException ```
Kotlin
Kotlin tries to eliminate null pointer exceptions by distinguishing nullable and non-nullable types at compile-time.
```kotlin var str: String = "Hello" str = null // Compile error: Null can not be a value of a non-null type String var str2: String? = "Hello" str2 = null // This is OK ```
If you want to call a method or access a property on a nullable variable, you need to use the safe call operator `?.`
```kotlin var len = str2?.length // This will not throw an exception ```
Extensions
Java
Java does not natively support adding new functions to existing classes (also known as extensions). However, developers can simulate this by creating a new class that inherits from the existing one.
```java public class ExtendedString { private String str; public ExtendedString(String str) { this.str = str; } public int wordCount() { return str.split(" ").length; } } ```
Kotlin
In contrast, Kotlin allows for extension functions, which let you add new functions to existing classes without inheriting them.
```kotlin fun String.wordCount(): Int { return this.split(" ").size } val str = "Hello, World!" val count = str.wordCount() // Use the new function ```
This feature makes Kotlin’s code more expressive and easy to read.
Functional Programming
Both Kotlin and Java support functional programming, but the degree varies.
Java gained support for functional programming features like lambda expressions and streams with Java 8. This addition made it possible to write more expressive and compact code, especially when working with collections.
```java List<String> words = Arrays.asList("Hello", "World"); words.stream() .filter(word -> word.startsWith("H")) .forEach(System.out::println); // Prints "Hello" ```
On the other hand, Kotlin offers superior support for functional programming with a variety of powerful built-in functions like `map`, `reduce`, `filter`, and more.
```kotlin val words = listOf("Hello", "World") words.filter { it.startsWith("H") } .forEach { println(it) } // Prints "Hello" ```
In Kotlin, functions are first-class citizens, which allows for higher-order functions, i.e., functions that can accept other functions as parameters and return functions.
Community and Resources
Java, with its long-standing history, has an extensive community and an enormous amount of learning resources, libraries, and frameworks.
Kotlin’s community, while smaller, is rapidly growing. Kotlin has the advantage of full interoperability with Java, which means developers can use all existing Java libraries and frameworks while enjoying Kotlin’s modern features.
Conclusion
Choosing between Kotlin and Java ultimately depends on your specific needs and familiarity with the languages. If you are a beginner or value simplicity and conciseness, Kotlin might be your best bet. Kotlin’s syntax is more straightforward, and it provides several features that simplify Android app development, like null safety and extension functions.
On the other hand, if you have a strong Java background or are working on a large existing Java codebase, it might make more sense to stick with Java. But remember, the software industry is shifting, and the trend to hire Kotlin developers is on the rise, hinting at Kotlin’s growing popularity.
The good news is that choosing one does not rule out the other. Kotlin is fully interoperable with Java, meaning you can gradually introduce Kotlin into a Java codebase, or vice versa. This gradual introduction can be a perfect way to upskill your team or a convincing reason to hire Kotlin developers to diversify your team’s capabilities. This interoperability allows teams to reap the benefits of both languages, creating a flexible and productive development environment.
While Java has served us well for years, Kotlin, with its modern features and Google’s backing, seems set to become the new favorite for Android app development. Whether you’re looking to hire Kotlin developers or still prefer Java, it’s a good idea to become familiar with Kotlin. Its modern features could offer valuable additions to your Android app development toolkit, even if you continue to use Java for now.
Table of Contents
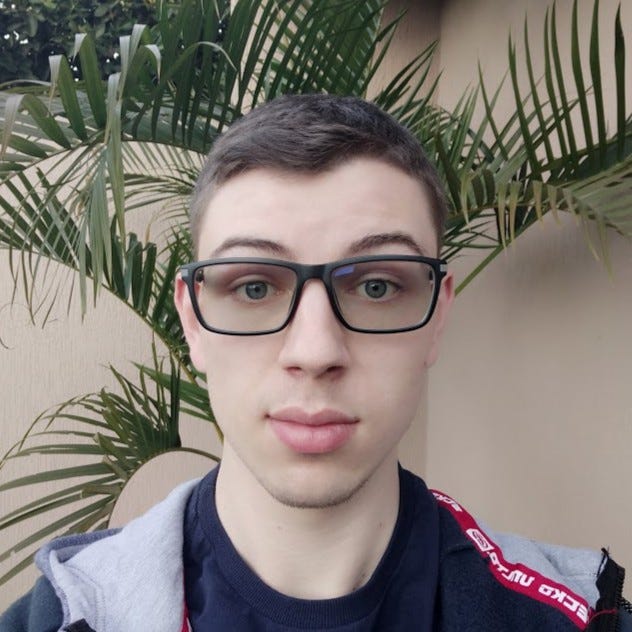
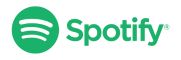