Kotlin for Web Development: Building Modern Backend Applications
Kotlin, a statically typed programming language developed by JetBrains, has gained significant popularity in recent years due to its simplicity, expressiveness, and versatility. Originally designed for Java Virtual Machine (JVM) but also capable of compiling to JavaScript and native code, Kotlin offers a seamless transition for Java developers and provides numerous benefits for web development, particularly in the backend space. In this blog post, we will explore how Kotlin can be used to build modern backend applications for web development, highlighting its features, advantages, and providing code samples along the way.
1. Why Kotlin for Backend Web Development?
Kotlin offers several advantages for backend web development, making it a compelling choice for building modern applications:
- Conciseness: Kotlin’s concise syntax reduces boilerplate code and increases developer productivity.
- Null Safety: Kotlin’s null safety feature helps eliminate the dreaded NullPointerExceptions by providing compile-time checks for nullable types.
- Interoperability with Java: Kotlin seamlessly integrates with existing Java code, allowing developers to leverage existing Java libraries and frameworks.
- Coroutines: Kotlin’s coroutines simplify asynchronous programming by providing a lightweight and efficient way to write asynchronous code.
- Modern Language Features: Kotlin incorporates modern language features like extension functions, data classes, and lambdas, making code more expressive and readable.
- Tooling and Community Support: Kotlin has excellent tooling support, including powerful IDE integration, and a vibrant community that actively contributes to libraries and frameworks.
2. Kotlin Features for Backend Development
2.1 Null Safety
Null safety is one of Kotlin’s most significant features. By distinguishing between nullable and non-nullable types at the language level, Kotlin enforces null safety and eliminates null pointer exceptions at compile-time. This feature leads to more reliable and robust code, reducing the risk of runtime crashes caused by null references.
Example:
kotlin fun getUsernameLength(username: String?): Int { return username?.length ?: 0 }
2.2 Extension Functions
Extension functions allow developers to add new functions to existing classes without modifying their source code. This feature promotes code reuse and improves the readability and maintainability of the codebase. In backend development, extension functions can be particularly useful for adding utility methods or domain-specific functionality to existing classes.
Example:
kotlin fun String.isPalindrome(): Boolean { val reversed = this.reversed() return this == reversed }
2.3 Coroutines
Kotlin’s coroutines provide a powerful way to write asynchronous code in a sequential and more readable manner. With coroutines, developers can easily perform non-blocking operations, such as network requests or database queries, without blocking the main thread. This leads to more scalable and efficient backend applications.
Example:
kotlin suspend fun fetchData(): String { delay(1000) // Simulating a long-running operation return "Data fetched successfully" }
2.4 Interoperability with Java
Kotlin has excellent interoperability with Java, allowing developers to seamlessly use Java libraries and frameworks within their Kotlin codebase. This feature is particularly advantageous when working with existing Java code or integrating with Java-based frameworks, enabling a smooth transition to Kotlin without the need for a complete rewrite.
3. Building a Backend Application with Kotlin
In this section, we will walk through building a simple backend application using Kotlin, leveraging popular libraries like Ktor for handling HTTP requests and Kotlin Exposed for data access.
3.1 Setting Up the Project
To get started, we need to set up a new Kotlin project using a build system like Gradle or Maven. Once the project is set up, we can configure the necessary dependencies for Ktor and Kotlin Exposed.
3.2 Handling HTTP Requests with Ktor
Ktor is a lightweight web framework for Kotlin that provides an expressive DSL for building web applications. We can use Ktor to handle HTTP requests, define routes, and handle different endpoints.
Example:
kotlin fun Application.module() { routing { get("/") { call.respondText("Hello, World!") } } }
3.3 Data Access with Kotlin Exposed
Kotlin Exposed is a database library for Kotlin that offers a DSL for interacting with databases. We can use Kotlin Exposed to define database schemas, query data, and perform CRUD operations.
Example:
kotlin object Users : Table() { val id = integer("id").autoIncrement() val name = varchar("name", length = 50) val email = varchar("email", length = 100) } fun getAllUsers(): List<User> = transaction { Users.selectAll().map { row -> User( id = row[Users.id], name = row[Users.name], email = row[Users.email] ) } }
3.4 Testing the Backend Application
Unit testing is crucial for ensuring the correctness of backend applications. Kotlin provides excellent testing frameworks like JUnit and Spek, which can be used to write tests for different components of the application, including routes, data access, and business logic.
Example:
kotlin class UserRoutesTest { @Test fun `GET request to /users should return a list of users`() { withTestApplication({ module() }) { handleRequest(HttpMethod.Get, "/users").apply { assertEquals(HttpStatusCode.OK, response.status()) assertEquals("[{\"id\":1,\"name\":\"John\",\"email\":\"john@example.com\"}]", response.content) } } } }
Conclusion
Kotlin provides a powerful and expressive language for building modern backend applications in the web development space. With features like null safety, extension functions, coroutines, and seamless interoperability with Java, Kotlin enables developers to create robust and scalable applications. By leveraging libraries like Ktor and Kotlin Exposed, developers can build efficient and maintainable backend systems. Whether you are a Java developer looking to transition to a more modern language or a newcomer to web development, Kotlin is a valuable tool to consider for building your next backend application.
Table of Contents
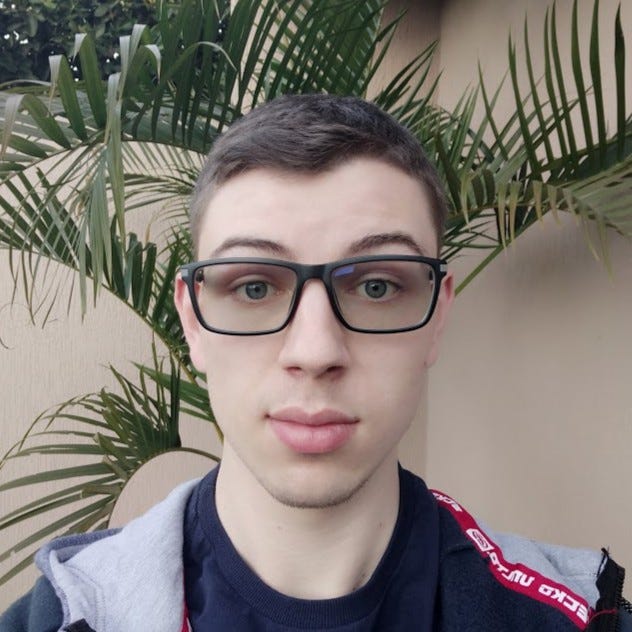
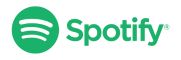