The Modern Developer’s Guide to Laravel API Authentication
With the ever-growing demand for web services, APIs (Application Programming Interfaces) have become quintessential components of modern web development. One of the most crucial aspects of building APIs is ensuring their security. Laravel, a leading PHP framework, offers several ways to authenticate users. However, in this blog post, we will focus on JWT (JSON Web Tokens) and how you can leverage them to secure your APIs in a Laravel application.
1. What is JWT?
JWT stands for JSON Web Token. It’s a compact and self-contained way of transmitting information between parties. The payload information is encoded as a JSON object, then signed either using a secret (HMAC algorithm) or a public/private key pair (RSA or ECDSA).
JWTs are used for authentication and information exchange, and their most significant advantage lies in their stateless nature, which makes them suitable for scalable APIs.
2. Setting Up Laravel and JWT Authentication
To integrate JWT into your Laravel application, we’ll use the “tymon/jwt-auth” package.
2.1. Install the package
Via Composer:
``` composer require tymon/jwt-auth ```
2.2. Publish the configuration
Once the package is installed, you’ll need to publish the JWT configuration file:
``` php artisan vendor:publish --provider="Tymon\JWTAuth\Providers\LaravelServiceProvider" ```
This will place a `jwt.php` configuration file in your `config` directory.
2.3. Generate JWT Secret
Next, generate the secret used to sign the tokens:
``` php artisan jwt:secret ```
This command will update your `.env` file with something like `JWT_SECRET=yourrandomstring`.
2.4. configure the Auth guard
In your `config/auth.php` file, set the API driver to `jwt`.
```php 'guards' => [ 'api' => [ 'driver' => 'jwt', 'provider' => 'users', ], ], ```
2.5. Set Up the User Model
Ensure that your User model implements the `Tymon\JWTAuth\Contracts\JWTSubject` interface and use the `JWTSubject` trait.
```php use Tymon\JWTAuth\Contracts\JWTSubject; class User extends Authenticatable implements JWTSubject { // ... public function getJWTIdentifier() { return $this->getKey(); } public function getJWTCustomClaims() { return []; } } ```
3. Implementing JWT Authentication
Now that our Laravel app is set up to use JWTs, let’s look at some basic operations:
3.1. Logging in and Retrieving a Token
For users to access secured routes, they need to provide a valid JWT. This often starts with the login process.
```php public function login(Request $request) { $credentials = $request->only('email', 'password'); if (! $token = auth('api')->attempt($credentials)) { return response()->json(['error' => 'Unauthorized'], 401); } return $this->respondWithToken($token); } protected function respondWithToken($token) { return response()->json([ 'access_token' => $token, 'token_type' => 'bearer', 'expires_in' => auth('api')->factory()->getTTL() * 60 ]); } ```
3.2. Accessing Secured Routes
To secure a route, use the `auth:api` middleware.
```php Route::group(['middleware' => ['auth:api']], function() { Route::get('user', 'UserController@getAuthenticatedUser'); }); ```
The controller method:
```php public function getAuthenticatedUser() { return response()->json(auth('api')->user()); } ```
3.3. Refreshing a Token
JWTs have an expiration time, and when that time is near or has elapsed, you’ll need to refresh the token.
```php public function refreshToken() { return $this->respondWithToken(auth('api')->refresh()); } ```
3.4. Logging out and Invalidating the Token
To invalidate a token (i.e., logging a user out):
```php public function logout() { auth('api')->logout(); return response()->json(['message' => 'Successfully logged out']); } ```
Conclusion
JWT provides a flexible and stateless solution to authenticate users in an API-centric environment. By integrating the `tymon/jwt-auth` package, Laravel developers can easily secure their applications and ensure that only authenticated users access specific routes. As with all authentication methods, it’s essential to understand the underlying principles, keep your applications updated, and follow best practices to maintain a high level of security.
Table of Contents
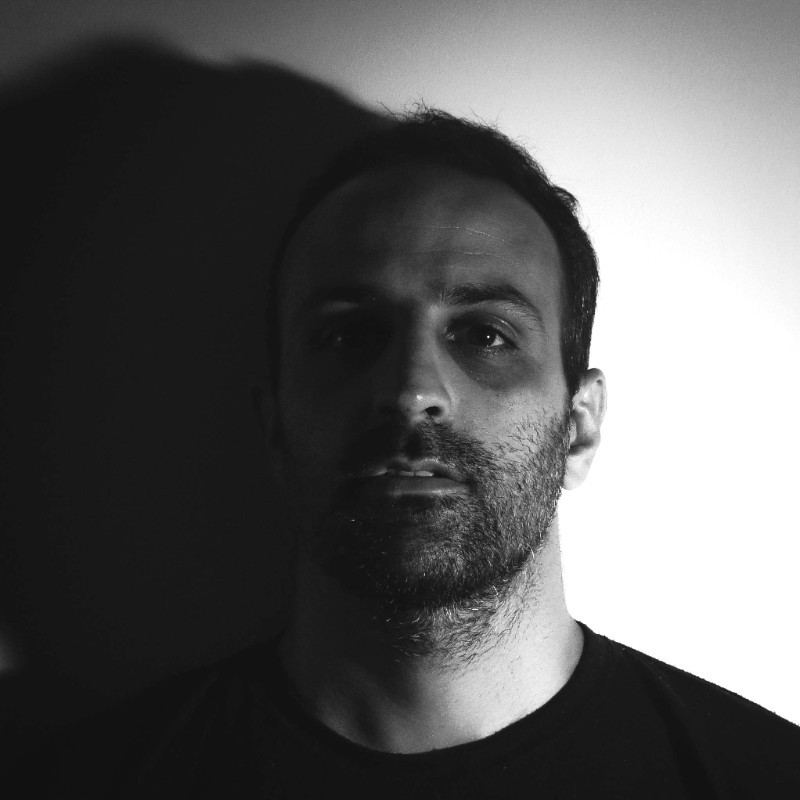
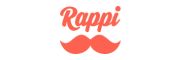