Laravel API Resources: Transforming Data for APIs
In the realm of modern web development, creating robust and efficient APIs has become a pivotal aspect of building successful applications. When dealing with APIs, data transformation plays a crucial role in shaping the output that clients receive. Laravel, a popular PHP framework, offers a powerful tool called API Resources that simplifies and optimizes the process of transforming data for APIs. In this blog post, we will delve into the world of Laravel API Resources, exploring its features, benefits, and how to use them effectively to transform your data and provide a seamless experience for your API consumers.
Table of Contents
1. Understanding the Role of Data Transformation in APIs
APIs act as bridges between different software applications, enabling them to communicate and exchange data seamlessly. However, the data that a server sends to clients might not always be in the format that the clients need. This is where data transformation comes into play. Data transformation involves converting the raw data fetched from a database or other sources into a format that is suitable for the API response. This could involve formatting, filtering, restructuring, and even merging data from multiple sources.
Without proper data transformation, APIs might send excessive or redundant data, leading to slow response times and unnecessary bandwidth consumption. Moreover, APIs could expose sensitive or unnecessary information to clients if not transformed correctly. To tackle these issues and provide an efficient and tailored API response, Laravel introduced API Resources.
2. Introducing Laravel API Resources
Laravel API Resources are a powerful feature designed to help developers transform data seamlessly for API responses. They allow you to control the structure and format of the data sent to clients, ensuring that the API output aligns with the client’s needs. API Resources provide a structured way to define the transformation logic, making your code more organized and maintainable.
3. Benefits of Using Laravel API Resources
Data Transformation Control: With API Resources, you have full control over how your data is presented in the API response. You can easily format, rename, and restructure attributes as needed.
- Performance Optimization: By specifying exactly which data should be included in the response, API Resources help optimize performance by reducing the payload size. This results in faster response times and a better user experience.
- Versioning: Laravel API Resources facilitate versioning your API responses. This means that as your API evolves and changes, you can ensure that clients receive the correct version of the data structure, maintaining backward compatibility when needed.
- Eloquent Integration: API Resources seamlessly integrate with Eloquent, Laravel’s ORM (Object-Relational Mapping). This allows you to transform Eloquent models into custom data structures for your API responses effortlessly.
- Consistent Output: API Resources enable you to maintain a consistent data structure across different API endpoints. This helps API consumers understand and work with the data more effectively.
4. Using Laravel API Resources
Let’s dive into using Laravel API Resources in your project. To get started, you’ll need a Laravel application up and running, and you should have a basic understanding of how Eloquent models and controllers work.
Step 1: Creating an API Resource Class
To begin, you need to create an API Resource class. This class will define how your data is transformed for the API response. You can use the php artisan command-line tool to generate a new API Resource class:
bash php artisan make:resource ArticleResource
This will create a new file named ArticleResource.php in the app/Http/Resources directory.
Step 2: Defining the Transformation
Open the ArticleResource.php file and define the transformation logic within the toArray method. This method is where you specify the attributes that should be included in the API response and how they should be transformed. Here’s an example of how the ArticleResource class might look:
php namespace App\Http\Resources; use Illuminate\Http\Resources\Json\JsonResource; class ArticleResource extends JsonResource { public function toArray($request) { return [ 'id' => $this->id, 'title' => $this->title, 'content' => $this->content, 'published_at' => $this->created_at->format('Y-m-d'), // ... additional attributes and transformations ]; } }
In this example, we’re transforming an Article model, including its id, title, content, and the formatted published_at attribute in the API response.
Step 3: Using the Resource in a Controller
Once you’ve defined your API Resource, it’s time to use it in your controller. Open the relevant controller file and import the ArticleResource class. Then, use the resource in your API response method, like so:
php use App\Http\Resources\ArticleResource; use App\Models\Article; class ArticleController extends Controller { public function show($id) { $article = Article::findOrFail($id); return new ArticleResource($article); } }
By returning an instance of the ArticleResource class, you’re instructing Laravel to apply the transformation logic defined in the resource when sending the API response.
5. Advanced Transformations and Additional Features
Laravel API Resources provide various features for advanced data transformation:
- Relationships: API Resources allow you to include related models and resources in your API response. This enables you to provide comprehensive data to clients without requiring additional requests.
- Collections: When dealing with collections of resources, API Resources can transform each item in the collection using the same logic defined in the resource class.
- Conditional Transformation: You can conditionally include or exclude attributes based on certain criteria, enhancing the flexibility of your API responses.
- Custom Methods: API Resources let you define custom methods to include additional data or perform specific transformations.
Conclusion
Laravel API Resources empower developers to transform and format data for APIs efficiently and effectively. By providing control over the data transformation process, these resources enable you to optimize performance, maintain a consistent output, and enhance the user experience of your APIs. With features like versioning, Eloquent integration, and advanced transformation capabilities, Laravel API Resources are a must-have tool for any Laravel-based API project. By following the steps outlined in this blog post, you can seamlessly implement API Resources in your Laravel application and elevate the quality of your API responses. So, embrace the power of Laravel API Resources and transform your APIs into a well-structured and performant communication channel between your applications.
In conclusion, Laravel API Resources offer a streamlined approach to data transformation for APIs. By allowing developers to define precisely how data is presented in API responses, these resources enhance performance, flexibility, and maintainability of API projects. By understanding their benefits and learning how to use them effectively, you can take your Laravel API development to the next level. So why wait? Dive into the world of Laravel API Resources and unlock the potential of transforming your API data seamlessly.
Table of Contents
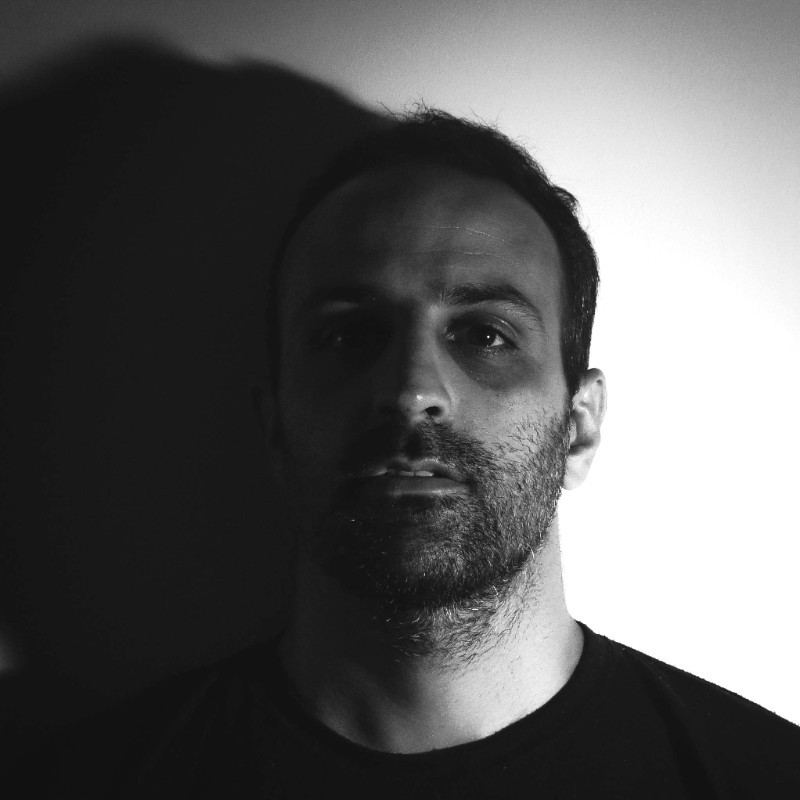
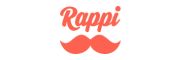