How to create API routes in Laravel?
Creating API routes in Laravel is like mapping out the pathways through which your application communicates with the outside world—it’s a crucial step in building a robust and accessible API. Let’s break down how to create API routes in Laravel in a user-friendly way:
To begin, open your Laravel project in your preferred code editor. Laravel makes it incredibly easy to define API routes using the same expressive syntax as web routes. API routes are typically defined in the routes/api.php file within your Laravel project.
Start by creating a new route in the api.php file. Laravel provides a set of helper methods for different HTTP methods such as get, post, put, patch, delete, and resource. For example, let’s create a simple route that responds to GET requests at the /api/hello endpoint:
php Route::get('/hello', function () { return ['message' => 'Hello, this is your API!']; });
In this example, when a user makes a GET request to /api/hello, Laravel will execute the provided closure function and return a JSON response with a greeting message.
You can also create routes that are handled by controller methods for better organization and maintainability. Suppose you have a UserController with an index method that retrieves a list of users. You can define an API route for this endpoint like so:
Php Route::get('/users', 'UserController@index');
This route points to the index method of the UserController class, and it will be triggered when a GET request is made to /api/users.
Laravel also provides the resource method for easily creating routes that correspond to the typical CRUD operations on a resource. For instance, to create routes for managing articles, you can use:
php Route::resource('articles', 'ArticleController');
This single line generates routes for index, create, store, show, edit, update, and destroy operations related to articles.
Once you’ve defined your API routes, you can start testing them using tools like Postman or curl. Make requests to your defined endpoints to see your API in action.
By following these steps, you’ve successfully created API routes in Laravel. Whether you’re building a simple API or a complex one with various endpoints, Laravel’s intuitive routing system provides a solid foundation for building powerful and accessible APIs.
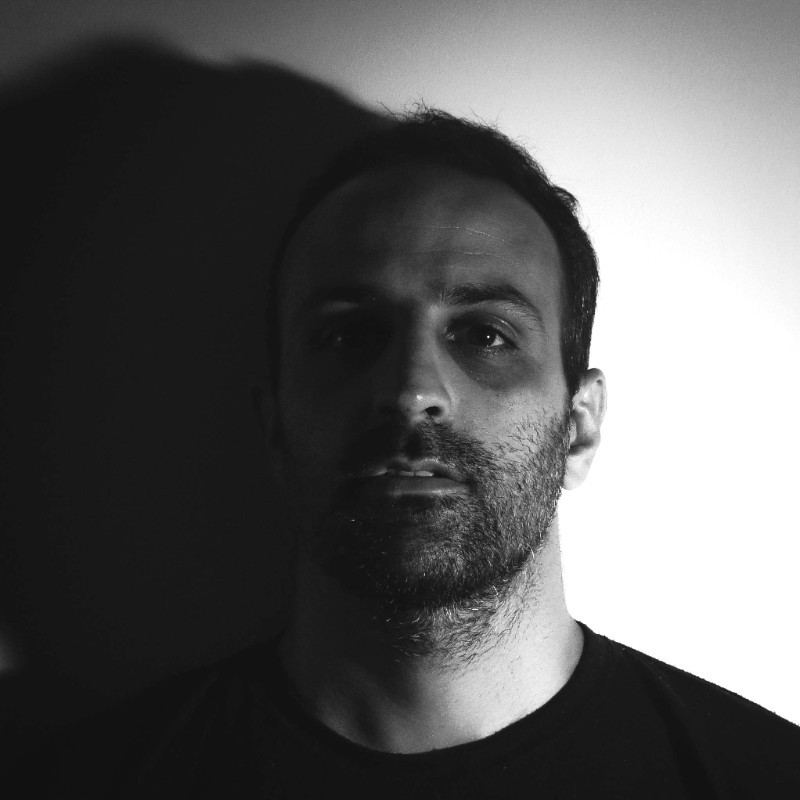
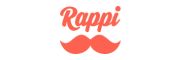