Elevate Your Laravel API with Effective Versioning Strategies
In the dynamic world of web development, APIs serve as the crucial connectors enabling diverse systems to communicate and share data seamlessly. As your application scales, the need to evolve and update your API to accommodate new features becomes imperative. This is where the expertise of seasoned Laravel developers can make a profound difference. However, these updates should not disrupt the existing clients. Hence, API versioning becomes a critical aspect of your API design strategy.
Table of Contents
In this blog post, we’re going to shine a spotlight on Laravel, an incredibly robust PHP framework, and delve into the best practices for efficient API version management. Whether you’re planning to hire Laravel developers for enhancing your project’s real-time communication abilities using Laravel Broadcasting or keen on augmenting your own skills, these insights and practical examples will provide a comprehensive understanding of efficient API versioning.
1. Understanding API Versioning
API versioning is the process of making changes or updates to your API without affecting the current clients using your API. Essentially, API versioning allows you to have multiple versions of your API running simultaneously, which can handle requests based on their versions.
2. Why is API Versioning Necessary?
Changes and improvements are inherent parts of a dynamic web application. However, when you modify your API, you risk disrupting the service for existing clients. These clients might be using older versions of your API and might not have updated their systems to accommodate your changes.
API versioning mitigates this problem. It allows you to continue providing service to older clients while providing a platform for new features and updates. Therefore, API versioning is not just an option; it’s a necessity for maintaining and improving your API over time.
3. API Versioning Strategies
There are several strategies for API versioning, including URI versioning, request header versioning, and media type versioning. The choice of versioning strategy largely depends on your specific use case and preferences.
Laravel does not impose a specific versioning approach, which makes it flexible and adaptable according to your needs. However, the most common method used in Laravel API versioning is URI versioning, where the version number is included in the URL itself.
4. API Versioning in Laravel: Best Practices
4.1. Versioning via URI
Including the version number in the URI is a straightforward approach. The major advantage of this method is its simplicity and ease of use for API consumers.
For example, you could structure your URL as follows:
``` https://api.yourwebsite.com/v1/users https://api.yourwebsite.com/v2/users ```
In this case, `v1` and `v2` represent the version numbers.
In Laravel, you can achieve this by defining the routes in your `api.php` file as follows:
```php Route::prefix('v1')->group(function () { Route::get('users', 'App\Http\Controllers\v1\UserController@index'); }); Route::prefix('v2')->group(function () { Route::get('users', 'App\Http\Controllers\v2\UserController@index'); }); ```
Here, `v1` and `v2` are the directories that contain the versioned controllers.
4.2. Versioning via Request Header
Another popular method for versioning in Laravel is using custom request headers. In this method, the client specifies the required API version in the request’s header.
For instance, a client can make a request with a custom header like this:
``` Accept: application/vnd.yourwebsite.v1+json ```
Here, `v1` is the version number.
You can handle this in Laravel by using a middleware to check the `Accept` header and load the corresponding controller. Create a middleware `ApiVersion.php` as follows:
```php namespace App\Http\Middleware; use Closure; class ApiVersion { public function handle($request, Closure $next) { $version = $request->header('Accept'); if(strpos($version, 'vnd.yourwebsite.v1') !== false){ // Load controllers from v1 } else if(strpos($version, 'vnd.yourwebsite.v2') !== false){ // Load controllers from v2 } return $next($request); } } ```
4.3. Semantic Versioning
While the above methods focus on how to implement versioning, semantic versioning or SemVer is a convention on deciding the version numbers. It follows the format of `MAJOR.MINOR.PATCH`, where:
– `MAJOR` version increment indicates incompatible API changes.
– `MINOR` version increment indicates the addition of functionality in a backward-compatible manner.
– `PATCH` version increment indicates backward-compatible bug fixes.
This helps in understanding the level of changes in the API with the version number itself.
Final Thoughts
API versioning is vital for the longevity and scalability of your API. With Laravel’s flexibility, you can implement a versioning strategy that suits your needs. If you are looking to hire Laravel developers, ensure they understand the importance of maintaining backward compatibility whenever possible and keeping API consumers in mind when making changes. Remember, an API is a contract between you and the clients using it; versioning allows you to change that contract without breaching it.
Hiring Laravel developers with a proper understanding of API versioning and its best practices implementation significantly contributes to the quality and effectiveness of your Laravel API. This knowledge ensures they are able to create robust, real-time communication features using Laravel Broadcasting, which can elevate the user experience your API offers. It might require a little extra effort in the beginning, but it’s worth it for the long-term success and stability of your API.
Table of Contents
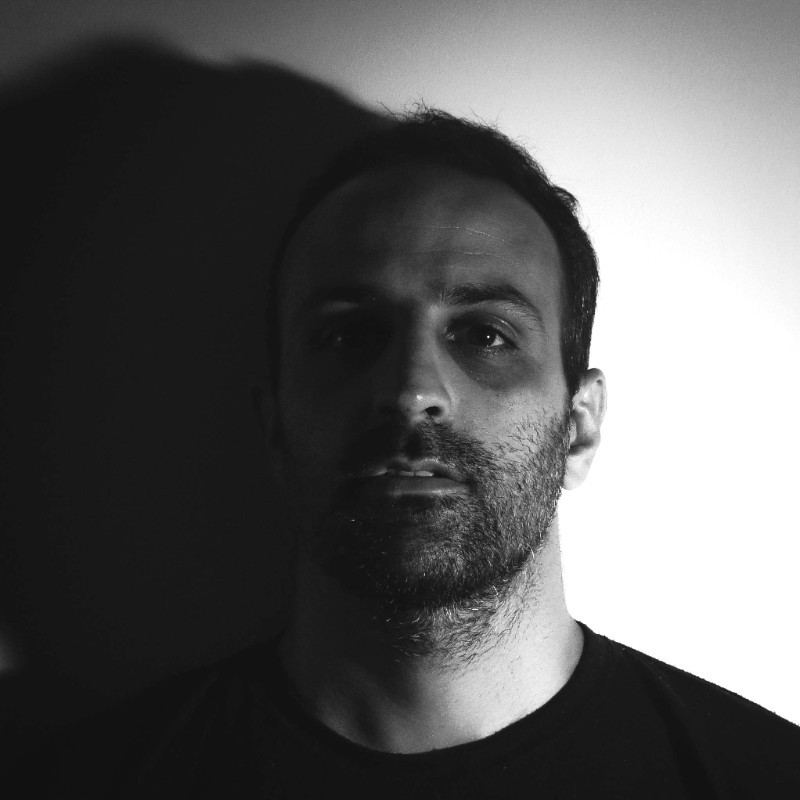
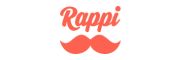