Building APIs with Laravel Passport: Token-Based Authentication
In today’s digital landscape, building robust and secure APIs is crucial for the success of web and mobile applications. One of the essential aspects of API development is authentication, ensuring that only authorized users can access protected resources. Laravel Passport provides a seamless way to implement token-based authentication in your Laravel API, offering a scalable and secure solution. In this blog, we will explore the features of Laravel Passport, step-by-step implementation, and code samples to demonstrate its usage.
What is Laravel Passport?
Laravel Passport is an official Laravel package that enables developers to implement OAuth2 authentication in their applications effortlessly. It leverages the power of the Laravel framework, providing a simple and secure way to manage access tokens, authorizations, and client applications. With Passport, you can issue access tokens for various OAuth2 flows, such as personal access tokens, password grants, and authorization code grants.
Installing and Configuring Laravel Passport
To begin, you need to install Laravel Passport into your Laravel application. Open your terminal and run the following command:
bash composer require laravel/passport
After the installation, run the following command to publish the Passport configuration files:
php artisan passport:install
This command will create the encryption keys needed to generate secure access tokens. Next, you should migrate the Passport database tables using the following command:
php artisan migrate
Finally, you need to add the Passport service provider to the config/app.php file:
php 'providers' => [ // Other providers... Laravel\Passport\PassportServiceProvider::class, ],
Creating API Routes and Controllers
Once Laravel Passport is installed and configured, you can start building your API routes and controllers. Open the routes/api.php file and define your API routes. For example:
php Route::middleware('auth:api')->group(function () { Route::get('/user', function (Request $request) { return $request->user(); }); });
In the above code snippet, we’ve defined an authenticated route /user that returns the authenticated user’s information. You can create additional routes based on your application’s requirements.
User Registration and Login
Before implementing authentication, we need to create a user registration and login functionality. Start by creating a UserController using the following command:
go php artisan make:controller UserController
Inside the UserController, define methods for user registration and login. Here’s an example of the register method:
php public function register(Request $request) { $validatedData = $request->validate([ 'name' => 'required|string|max:255', 'email' => 'required|string|email|max:255|unique:users', 'password' => 'required|string|min:8|confirmed', ]); $user = User::create([ 'name' => $validatedData['name'], 'email' => $validatedData['email'], 'password' => bcrypt($validatedData['password']), ]); $accessToken = $user->createToken('authToken')->accessToken; return response(['user' => $user, 'access_token' => $accessToken]); }
In the register method, we validate the incoming request data, create a new user, and generate an access token using the createToken method provided by Passport. Similarly, you can create a login method to authenticate users and issue access tokens.
Generating Access Tokens
With the user registration and login functionality in place, users can now obtain access tokens. When a user successfully logs in, Laravel Passport generates an access token that can be used to authenticate subsequent requests. This token should be included in the headers of API requests. Here’s an example of using the access token in an API request:
php $response = $client->request('GET', '/api/user', [ 'headers' => [ 'Accept' => 'application/json', 'Authorization' => 'Bearer ' . $accessToken, ], ]);
Protecting API Routes
To protect your API routes and ensure that only authenticated users can access them, you need to add the auth:api middleware to the route or group of routes. This middleware provided by Laravel Passport verifies the access token and authenticates the user. Here’s an example:
php Route::middleware('auth:api')->group(function () { // Protected routes });
By adding the auth:api middleware, any request made to these routes without a valid access token will be rejected.
Revoking Access Tokens
Laravel Passport allows users to revoke their access tokens, improving security and giving users control over their authorized applications. To revoke an access token, you can call the revoke method on the PersonalAccessToken instance. Here’s an example:
php use Laravel\Passport\Token; public function revokeToken(Request $request) { $request->user()->tokens()->where('id', $tokenId)->delete(); return response(['message' => 'Token revoked']); }
In the above code, we use the tokens method on the authenticated user to retrieve their access tokens. Then, we delete the token matching the specified ID.
Refreshing Access Tokens
Access tokens issued by Laravel Passport have an expiration time. To avoid forcing users to repeatedly log in, Passport provides a way to refresh access tokens. By using the refresh token, a new access token can be generated without requiring the user’s credentials. Here’s an example:
php use Laravel\Passport\HasApiTokens; public function refreshToken(Request $request) { $user = User::find($request->user()->id); $newToken = $user->createToken('authToken')->accessToken; return response(['access_token' => $newToken]); }
In the above code, we retrieve the authenticated user and generate a new access token using the createToken method.
Conclusion
Token-based authentication using Laravel Passport provides a secure and scalable solution for building APIs. In this blog, we explored the features of Laravel Passport and walked through the step-by-step implementation process. By following the examples and code samples provided, you can confidently integrate token-based authentication into your Laravel API, ensuring that your resources are protected and accessible only to authorized users.
Table of Contents
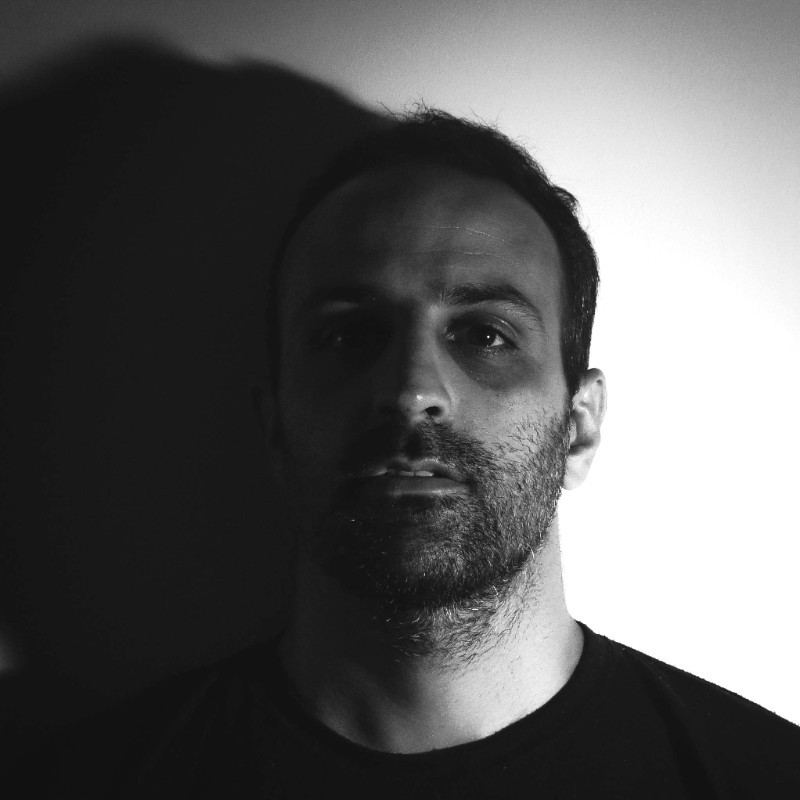
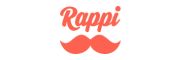