How to Seamlessly Build Single-Page Applications with Laravel & Inertia.js
Single-page applications (SPAs) have become an essential part of modern web development, providing a seamless user experience by dynamically updating the page without refreshing it. While traditional SPA development may require working with complex JavaScript frameworks and APIs, Inertia.js has emerged as an elegant solution to bridge the gap between the server-side Laravel framework and client-side Vue.js (or React, Svelte). This has made it increasingly popular among businesses looking to hire Laravel developers for their projects.
Table of Contents
In this post, we’ll explore how to build a single-page application using Laravel and Inertia.js. We’ll walk through the fundamental concepts and provide examples to help you understand how to integrate these technologies efficiently. This will also be of interest if you’re planning to hire Laravel developers and want to understand the benefits and the process of building an SPA with Laravel and Inertia.js.
1. Introduction to Inertia.js
Inertia.js allows you to create fully client-side rendered, single-page apps, using your favorite server-side routing and controllers. You can write controllers using standard Laravel code and return Inertia responses to client-side JavaScript pages.
2. Installation and Setup
To get started, we’ll need to install Inertia.js and a client-side adapter like Vue.js, React, or Svelte. We’ll use Vue.js in our example.
First, let’s install the Inertia.js server-side package:
```bash composer require inertiajs/inertia-laravel ```
Next, install the client-side packages:
```bash npm install @inertiajs/inertia @inertiajs/inertia-vue3 ```
3. Setting up the JavaScript Files
Configure your `app.js` file with Inertia and Vue:
```javascript import { createApp, h } from 'vue'; import { App, plugin } from '@inertiajs/inertia-vue3'; const el = document.getElementById('app'); createApp({ render: () => h(App, { initialPage: JSON.parse(el.dataset.page), resolveComponent: name => require(`./Pages/${name}`).default, }), }).use(plugin).mount(el); ```
4. Creating Your First Page
Let’s create a simple page to see Inertia in action.
In your Laravel controller, you can return an Inertia response:
```php use Inertia\Inertia; public function show() { return Inertia::render('Welcome', [ 'data' => 'My Inertia and Laravel App!', ]); } ```
Then, create the corresponding Vue component at `resources/js/Pages/Welcome.vue`:
```vue <template> <div> <h1>{{ data }}</h1> </div> </template> <script> export default { props: { data: String, }, } </script> ```
5. Handling Forms with Inertia
Inertia also simplifies handling forms. Here’s an example of how you can manage a form submission:
5.1. Controller
```php public function update(Request $request) { $request->validate([ 'name' => ['required', 'max:50'], ]); // Update logic here return redirect()->route('dashboard')->with('success', 'Updated successfully'); } ```
5.2. Vue Component
```vue <template> <form @submit.prevent="submit"> <input type="text" v-model="form.name" required /> <button type="submit">Update</button> </form> </template> <script> import { Inertia } from '@inertiajs/inertia'; export default { data() { return { form: { name: this.name, }, }; }, methods: { submit() { Inertia.post('/dashboard', this.form); }, }, props: { name: String, }, }; </script> ```
Conclusion
Laravel and Inertia.js form a powerful duo that simplifies the process of building engaging single-page applications. By blending server-side logic with client-side rendering, Inertia empowers developers to create modern, dynamic interfaces without abandoning the tried-and-true practices of traditional web development.
Whether you’re a Laravel veteran looking to embrace SPAs, a front-end developer interested in leveraging the power of Laravel, or looking to hire Laravel developers for your projects, Inertia.js offers a fresh and intuitive approach to building scalable, maintainable, and user-friendly web applications.
Table of Contents
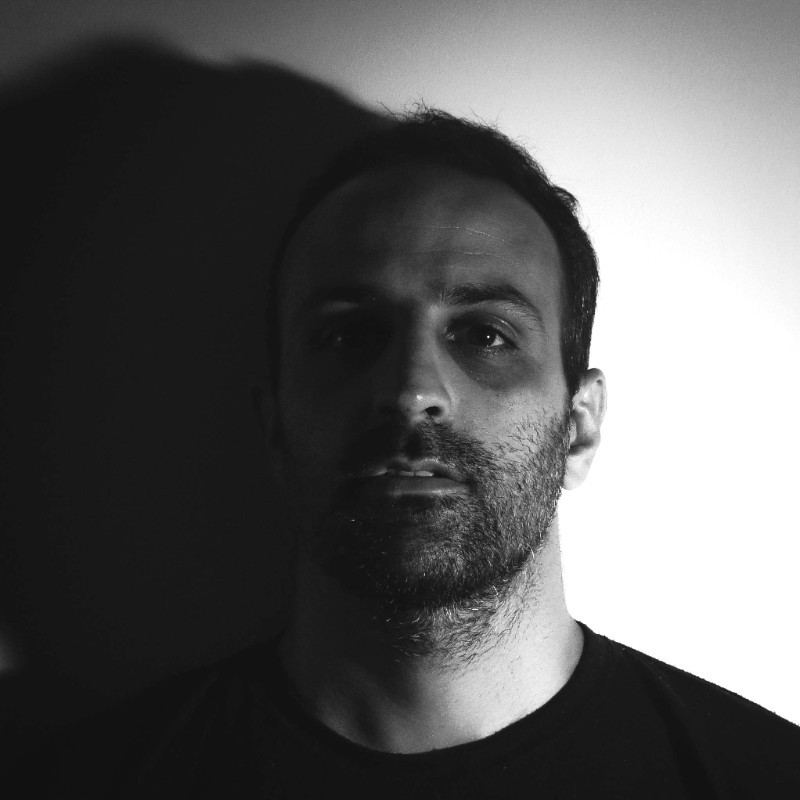
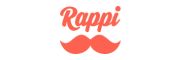