Revolutionize Your Web App with Laravel Broadcasting: A Step-By-Step Guide
In a world that’s relentlessly moving towards real-time data communication, the advent of Laravel Broadcasting has dramatically simplified the way developers implement real-time communication features in web applications. The need for such advanced features has made many businesses and organizations keen to hire Laravel developers with expertise in Laravel Broadcasting.
Table of Contents
Laravel is a renowned open-source PHP framework widely adopted in web application development following the Model-View-Controller (MVC) architectural pattern. Its elegant syntax and the ability to perform tasks involving complex web development processes set it apart from its peers. Laravel Broadcasting, a remarkable feature of Laravel, offers an intuitive interface for developers to seamlessly implement broadcasting events in their applications. Whether you’re a developer seeking to broaden your understanding of Laravel Broadcasting or a business aiming to hire Laravel developers for your project, this article will serve as an invaluable guide. We will explore Laravel Broadcasting in detail, providing practical examples to illustrate its proficiency in facilitating real-time communication channels.
1. What is Laravel Broadcasting?
Broadcasting, in the context of Laravel, enables your application to share specific types of messages with others, helping you achieve real-time data updates. Laravel’s event broadcasting allows you to broadcast your server-side Laravel events to your client-side JavaScript application using a driver-based approach to select your preferred broadcast channel.
Laravel supports several broadcast drivers out of the box — Pusher Channels, Redis, and a log driver for local development. However, you can also write your own driver if none of these meet your application’s needs.
2. Setting Up Laravel Broadcasting
Before we delve into examples, let’s first set up Laravel Broadcasting.
First, ensure you’ve Laravel installed. If not, use Composer’s create-project command:
```bash composer create-project --prefer-dist laravel/laravel blog ```
By default, Laravel’s broadcast option is turned off. To turn it on, we need to change the BROADCAST_DRIVER in the .env file to Pusher, Redis, Log, or your custom driver:
```bash BROADCAST_DRIVER=pusher ```
After that, you need to configure the broadcasting connection settings in your config/broadcasting.php file:
```php 'connections' => [ 'pusher' => [ 'driver' => 'pusher', 'key' => env('PUSHER_APP_KEY'), 'secret' => env('PUSHER_APP_SECRET'), 'app_id' => env('PUSHER_APP_ID'), 'options' => [ 'cluster' => env('PUSHER_APP_CLUSTER'), 'useTLS' => true, ], ], ```
3. Creating and Broadcasting Events
Laravel’s artisan command-line tool can be used to generate an event class.
```bash php artisan make:event UserRegistered ```
This command will create a new event class in the app/Events directory. Here, we will create an event that will be fired when a new user registers.
In the newly created UserRegistered.php file, the `broadcastOn` method returns the channels that the event should broadcast on. We will modify this method to broadcast the event on a public channel:
```php public function broadcastOn() { return new Channel('users'); } ```
4. Receiving Broadcasts
We can listen for the UserRegistered event in our JavaScript application. If you’re using the Pusher Channels broadcast driver, you may use the Echo Javascript package to receive the broadcasts.
First, install Echo via NPM:
```bash npm install --save laravel-echo pusher-js ```
Next, in your bootstrap.js file, initialize Echo:
```javascript import Echo from 'laravel-echo'; window.Pusher = require('pusher-js'); window.Echo = new Echo({ broadcaster: 'pusher', key: process.env.MIX_PUSHER_APP_KEY, cluster: process.env.MIX_PUSHER_APP_CLUSTER, encrypted: true }); ```
Then, in your client-side script, subscribe to the channel and listen for the UserRegistered event:
```javascript Echo.channel('users') .listen('UserRegistered', (e) => { console.log(e.user.name); }); ```
In the example above, ‘users’ is the name of the channel we broadcast to in the UserRegistered event. The listen method receives the name of the event to listen for and a callback that will be executed when the event is received.
5. Securing Channels
While broadcasting events on public channels is useful, sometimes we need to broadcast events on private channels where we can authorize the users.
First, to specify the event should be broadcast on a private channel, we can use the PrivateChannel class:
```php public function broadcastOn() { return new PrivateChannel('user.' . $this->user->id); } ```
In your routes/channels.php file, you can return a boolean value to authorize the user to listen on the channel:
```php Broadcast::channel('user.{id}', function ($user, $id) { return (int) $user->id === (int) $id; }); ```
Then, in your JavaScript application, subscribe to the private channel using the private method:
```javascript Echo.private('user.' + userId) .listen('UserRegistered', (e) => { console.log(e.user.name); }); ```
Conclusion
Laravel Broadcasting provides an elegant and easy-to-use interface for developers to implement real-time communication in web applications. Whether you’re tasked with sending real-time notifications or creating an interactive chat feature, Laravel Broadcasting is a game-changer. Its simplicity, flexibility, and the ability to utilize various drivers make it an ideal choice for developers. This versatility has led many organizations to hire Laravel developers to leverage this potent tool.
Keep in mind, while the examples provided in this article may seem straightforward, real-world applications can be more complex. However, this complexity shouldn’t deter you. The versatility of Laravel Broadcasting allows it to adapt to a broad spectrum of application needs, proving itself to be an invaluable asset in your Laravel toolkit. Therefore, if you’re planning to hire Laravel developers, ensure they are proficient with Laravel Broadcasting to make the most of its features for your web application.
Table of Contents
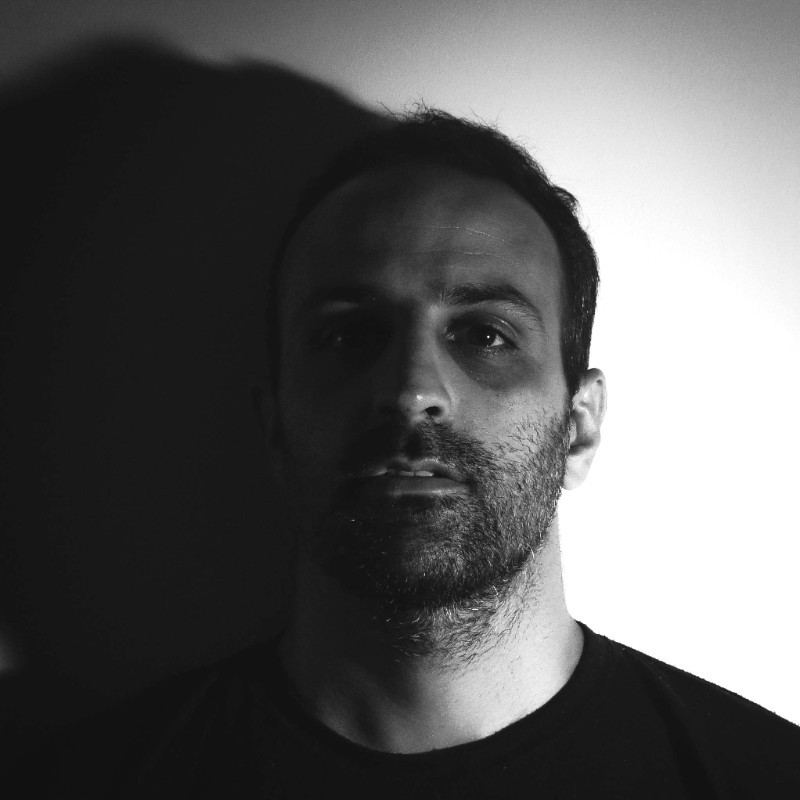
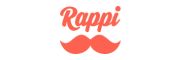