Get Started with Real-Time Notifications & Chats using Laravel Broadcasting
Laravel, one of the most popular PHP frameworks, offers developers a rich set of tools and utilities. One of its powerful features is broadcasting, which facilitates real-time communication in applications. With Laravel Echo, a JavaScript library, it becomes even easier to subscribe to channels and listen for events broadcasted by Laravel.
In this blog post, we’ll delve into how Laravel Broadcasting paired with Laravel Echo allows for real-time communication in web applications. We’ll walk through the setup process, demonstrate some practical use cases, and share some tips for optimizing performance.
1. Setting Up Broadcasting in Laravel
1.1. Configuration
First, you should configure your broadcasting settings. In the `.env` file, set the `BROADCAST_DRIVER` to the appropriate driver (`pusher`, `redis`, `log`, or `null`). For this example, we’ll use Pusher.
```bash BROADCAST_DRIVER=pusher ```
Then, install the necessary dependencies:
```bash composer require pusher/pusher-php-server "~4.0" ```
1.2. Service Provider
Laravel’s `BroadcastServiceProvider` located in `app/Providers` should be uncommented in the `config/app.php` providers array.
1.3. Event
Generate a new event using `artisan`.
```bash php artisan make:event UserEvent ```
This event will broadcast data when triggered.
2. Setting Up Laravel Echo
2.1. Installation
To get started with Echo, first install the Echo and its dependencies via NPM:
```bash npm install --save laravel-echo pusher-js ```
2.2. Configuration
Once installed, open the `resources/js/bootstrap.js` file and initialize Echo:
```javascript import Echo from 'laravel-echo'; window.Pusher = require('pusher-js'); window.Echo = new Echo({ broadcaster: 'pusher', key: process.env.MIX_PUSHER_APP_KEY, cluster: process.env.MIX_PUSHER_APP_CLUSTER, encrypted: true }); ```
Remember to replace the placeholders with your Pusher app credentials.
3. Practical Use Cases
3.1. Real-time Notifications
Imagine you want to notify a user when a new comment is added to their post. In your `CommentAdded` event, use the `ShouldBroadcast` interface and define the data you wish to broadcast.
```php public function broadcastWith() { return ['comment' => $this->comment->content]; } ```
On the client side, use Echo to listen for this event:
```javascript Echo.private(`post.${postId}`) .listen('CommentAdded', (e) => { console.log(e.comment); }); ```
3.2. Live Chat
For a chat system, when a message is sent, broadcast it:
```php public function broadcastWith() { return ['message' => $this->message->text]; } ```
On the client side, listen for new messages in a chat room:
```javascript Echo.private(`chat.${roomId}`) .listen('MessageSent', (e) => { console.log(e.message); }); ```
4. Optimization Tips
- Use Presence Channels: Presence channels give you real-time awareness of who’s subscribed to a channel. This is especially useful for features like showing who’s online in a chat room.
- Throttle and Debounce: If you expect high frequency of events (like a live typing indicator), debounce the events on the client side before broadcasting them. This will reduce server load.
- Secure Your Channels: Always use authentication and authorization practices when dealing with channels. Laravel offers built-in functionalities for this purpose.
Conclusion
Laravel Broadcasting combined with Laravel Echo offers a robust solution for adding real-time features to your web application. From live notifications to chat systems, the possibilities are endless. It’s easy to get started, and with the right practices, you can build highly interactive and dynamic applications.
Dive in, experiment, and experience the magic of real-time communication in Laravel!
Table of Contents
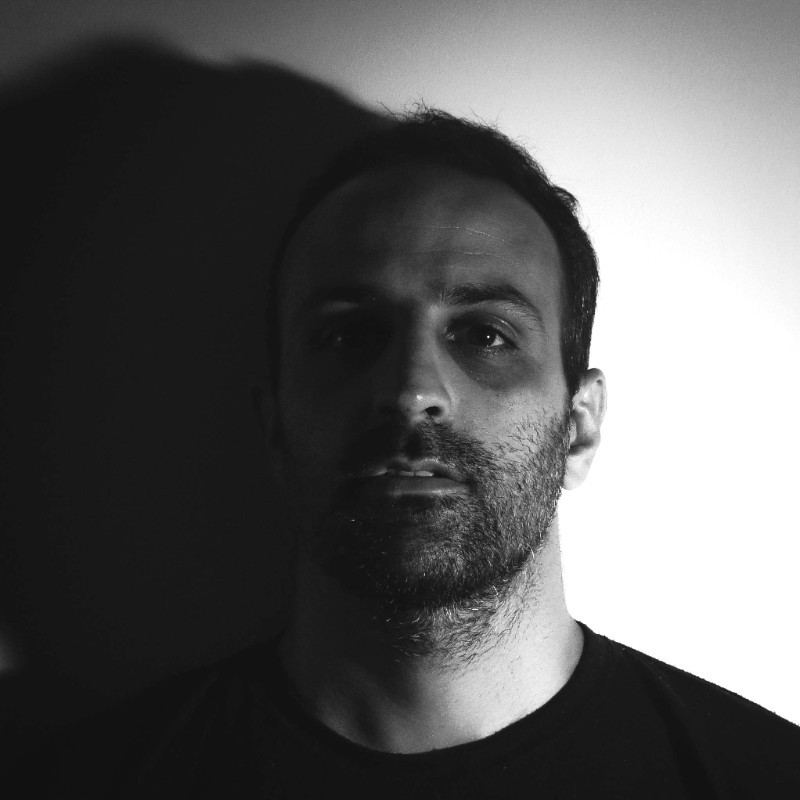
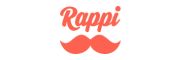