Discover the Power of Instant Feedback: Laravel’s Broadcasting Meets Pusher
The digital age demands real-time responses. From chat applications to notifications and even real-time data analytics, users expect immediate feedback. Laravel, being one of the most popular PHP frameworks, doesn’t disappoint when it comes to this expectation. It offers an elegant solution to the real-time conundrum through its broadcasting system. One of the most popular drivers for Laravel broadcasting is Pusher. In this article, we’ll delve into setting up real-time broadcasting in Laravel with Pusher.
1. What is Broadcasting?
In the context of Laravel, broadcasting allows you to share the same event name between your server-side code and your client-side JavaScript application. When something happens on the server that you want your client to know about without refreshing or polling, you broadcast an event.
2. Getting Started
Before diving in, ensure you have:
– Laravel installation up and running.
– Pusher account (free tier available).
– Basic knowledge of Laravel events.
Step 1: Install and Setup
After setting up a new Laravel project, install the required dependencies:
```bash composer require pusher/pusher-php-server npm install --save laravel-echo pusher-js ```
Next, in your `.env` file, fill in your Pusher credentials:
``` PUSHER_APP_ID=your_pusher_app_id PUSHER_APP_KEY=your_pusher_app_key PUSHER_APP_SECRET=your_pusher_secret PUSHER_APP_CLUSTER=your_pusher_cluster BROADCAST_DRIVER=pusher ```
Step 2: Setting Up the Event
Let’s assume you’re building a chat application and you want to broadcast a message when a user sends a new one. Start by generating a new event:
```bash php artisan make:event MessageSent ```
Edit the generated event (typically found in `app/Events`):
```php use Illuminate\Broadcasting\Channel; use Illuminate\Contracts\Broadcasting\ShouldBroadcast; class MessageSent implements ShouldBroadcast { public $message; public function __construct($message) { $this->message = $message; } public function broadcastOn() { return new Channel('chat'); } } ```
The `ShouldBroadcast` interface tells Laravel this event should be broadcasted.
Step 3: Firing the Event
When a message is sent, you can now fire the event:
```php broadcast(new MessageSent($message))->toOthers(); ```
Step 4: Listening on the Frontend
On the frontend, include the necessary scripts:
```javascript import Echo from 'laravel-echo'; window.Pusher = require('pusher-js'); window.Echo = new Echo({ broadcaster: 'pusher', key: process.env.MIX_PUSHER_APP_KEY, cluster: process.env.MIX_PUSHER_APP_CLUSTER, encrypted: true }); // Now, listen for the event: window.Echo.channel('chat') .listen('MessageSent', (e) => { console.log(e.message); }); ```
3. Advantages of Using Pusher with Laravel
- Simplicity: Pusher’s API and its integration with Laravel make it incredibly straightforward to set up real-time broadcasting.
- Scalability: Pusher is built for scaling. As your application grows, Pusher can handle the increased demand for real-time updates.
- Robustness: With features like reconnecting after a lost connection and encrypted messages, Pusher ensures that your real-time data is reliable and secure.
Conclusion
Real-time functionality has become a staple of modern web applications. Laravel, paired with Pusher, offers an efficient and elegant solution to integrate real-time broadcasting in your applications. The steps provided above are a basic introduction. As you explore further, you’ll uncover a plethora of options, like private channels, presence channels, and more, to enhance the user experience and increase the responsiveness of your applications. So, dive in and make your web apps truly real-time!
Table of Contents
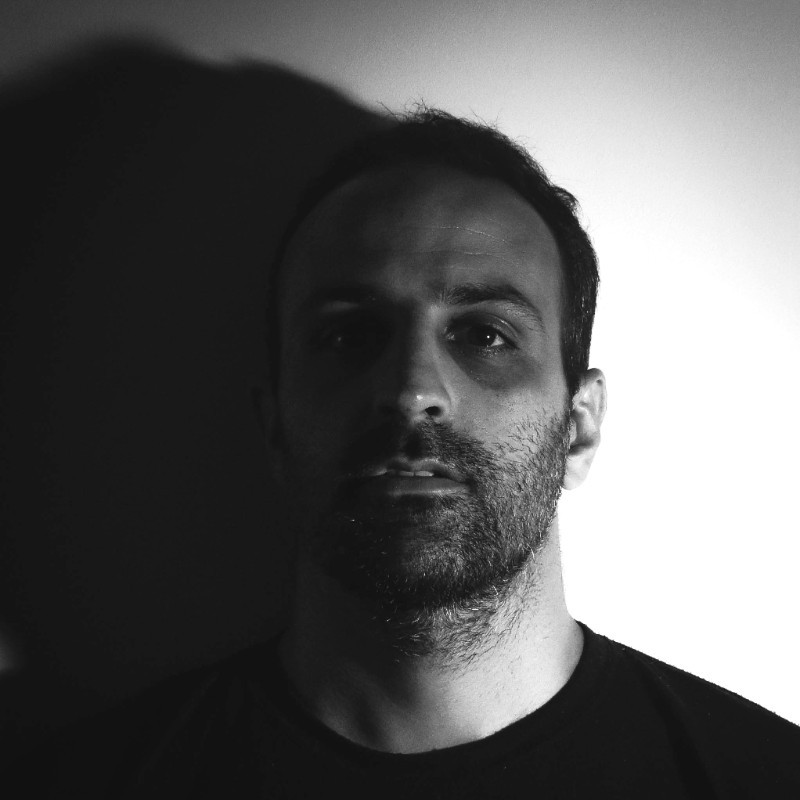
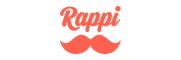