Step into the Future of API Development with Laravel and GraphQL
With the ever-increasing demand for more flexible and efficient APIs, many developers, including those who are looking to hire Laravel developers, are turning to GraphQL. GraphQL is a query language for APIs developed by Facebook. It enables front-end developers to request only the data they need, reducing the amount of data transferred and subsequently speeding up app loading times. This specificity simplifies client-side coding and promotes efficiency.
Table of Contents
When you combine GraphQL with Laravel, a highly popular PHP framework, you unlock a suite of incredibly powerful API development tools. As a result, many companies aiming to hire Laravel developers are now prioritizing experience with GraphQL in their search criteria. This powerful combination of GraphQL and Laravel is redefining the landscape of API development, making it more efficient, flexible, and powerful than ever before.
1. Understanding GraphQL and Laravel
Before we dive into the how-to, let’s briefly discuss what GraphQL and Laravel are.
GraphQL is a data query and manipulation language for APIs and a runtime for executing those queries with your existing data. Unlike REST where you need to make multiple round trips to fetch data or you might over-fetch data, with GraphQL, you specify exactly what you want and get precisely that.
Laravel, on the other hand, is a free, open-source PHP framework created by Taylor Otwell, designed for the development of web applications following the MVC (Model-View-Controller) architectural pattern. Laravel provides a clean and elegant syntax that is easy to grasp and use, leading to a streamlined development process.
2. Setting Up
To kickstart this Laravel GraphQL journey, you need to have a Laravel project setup. If you don’t already have one, use composer to create a new Laravel project:
```bash composer create-project --prefer-dist laravel/laravel graphql-demo ```
Next, you need to set up the GraphQL package for Laravel. We will be using the `rebing/graphql-laravel` package, which provides powerful functionality for developing a GraphQL API.
```bash composer require rebing/graphql-laravel ```
After installing the GraphQL package, publish the configuration file:
```bash php artisan vendor:publish --provider="Rebing\GraphQL\GraphQLServiceProvider" ```
3. Creating the GraphQL Schema
Now that we have our project set up, it’s time to create our first GraphQL schema. For this demonstration, we will be creating a simple blog system, with `Post` and `User` types.
3.1. Define the User Type
First, we will define the `User` type. Create a new file `UserType.php` in the `app/GraphQL/Types` directory and use the following code:
```php namespace App\GraphQL\Types; use GraphQL\Type\Definition\Type; use Rebing\GraphQL\Support\Type as GraphQLType; use App\Models\User; class UserType extends GraphQLType { protected $attributes = [ 'name' => 'User', 'description' => 'A user', 'model' => User::class, // define the model associated ]; public function fields(): array { return [ 'id' => [ 'type' => Type::nonNull(Type::int()), 'description' => 'The id of the user', ], 'name' => [ 'type' => Type::string(), 'description' => 'The name of user', ], 'email' => [ 'type' => Type::string(), 'description' => 'The email of user', ], ]; } } ```
In this file, we have defined the `User` type with `id`, `name`, and `email` fields.
3.2. Define the Post Type
Similarly, we will define the `Post` type. Create a new file `PostType.php` in the `app/GraphQL/Types` directory and use the following code:
```php namespace App\GraphQL\Types; use GraphQL\Type\Definition\Type; use Rebing\GraphQL\Support\Type as GraphQLType; use App\Models\Post; class PostType extends GraphQLType { protected $attributes = [ 'name' => 'Post', 'description' => 'A post', 'model' => Post::class, ]; public function fields(): array { return [ 'id' => [ 'type' => Type::nonNull(Type::int()), 'description' => 'The id of the post', ], 'title' => [ 'type' => Type::string(), 'description' => 'The title of the post', ], 'content' => [ 'type' => Type::string(), 'description' => 'The content of the post', ], 'user' => [ 'type' => Type::listOf(GraphQL::type('User')), 'description' => 'The author of the post', ], ]; } } ```
Notice that for the `user` field, we use the `User` type we have just defined. This defines the relationship between `User` and `Post`.
4. Defining Queries
Next, we will define the queries to fetch data from our server. First, we will define a query to fetch all users. Create a new file `UsersQuery.php` in the `app/GraphQL/Queries` directory:
```php namespace App\GraphQL\Queries; use Rebing\GraphQL\Support\Query; use GraphQL\Type\Definition\Type; use Rebing\GraphQL\Support\Facades\GraphQL; use App\Models\User; class UsersQuery extends Query { protected $attributes = [ 'name' => 'users', ]; public function type(): Type { return Type::listOf(GraphQL::type('User')); } public function resolve($root, $args) { return User::all(); // get all users } } ```
We can also define a query to fetch a single user by their ID. Let’s create a new file `UserQuery.php`:
```php namespace App\GraphQL\Queries; use Rebing\GraphQL\Support\Query; use GraphQL\Type\Definition\Type; use Rebing\GraphQL\Support\Facades\GraphQL; use App\Models\User; class UserQuery extends Query { protected $attributes = [ 'name' => 'user', ]; public function type(): Type { return GraphQL::type('User'); } public function args(): array { return [ 'id' => ['name' => 'id', 'type' => Type::nonNull(Type::int())], ]; } public function resolve($root, $args) { return User::findOrFail($args['id']); } } ```
Similarly, we can define queries for our `Post` type.
5. Mutations
Mutations in GraphQL allow us to create, update, and delete data. Let’s create a mutation to create a user. In the `app/GraphQL/Mutations` directory, create a new file `CreateUserMutation.php`:
```php namespace App\GraphQL\Mutations; use Rebing\GraphQL\Support\Mutation; use GraphQL\Type\Definition\Type; use App\Models\User; class CreateUserMutation extends Mutation { protected $attributes = [ 'name' => 'createUser', ]; public function type(): Type { return GraphQL::type('User'); } public function args(): array { return [ 'name' => ['name' => 'name', 'type' => Type::nonNull(Type::string())], 'email' => ['name' => 'email', 'type' => Type::nonNull(Type::string())], ]; } public function resolve($root, $args) { return User::create([ 'name' => $args['name'], 'email' => $args['email'], ]); } } ```
This mutation will allow us to create a new user by providing a `name` and `email`.
6. Testing the GraphQL API
Once we have defined our types, queries, and mutations, we can now test our API. To fetch all users, we can use the following query:
```graphql query { users { id name email } } ```
To create a new user, we can use the following mutation:
```graphql mutation { createUser(name: "Test User", email: "test@email.com") { id name email } } ```
Conclusion
Crafting an API has never been easier thanks to the dynamic duo of GraphQL and Laravel. By harnessing GraphQL’s data-driven approach, you can streamline your process, reducing unnecessary data fetching, and optimizing the number of requests. As a result, performance significantly improves due to less data being transferred over the network.
On the other side of the coin, Laravel simplifies backend development with its expressive syntax and powerful features, making it the go-to for many professional Laravel developers for hire.
By fusing these two technologies, Laravel and GraphQL not only stand strong independently but also synergize to provide a robust solution for building efficient, scalable APIs. If you’re looking to hire Laravel developers, this proficiency in GraphQL can be a crucial factor to consider in your selection process, as it opens up new dimensions in API development.
Table of Contents
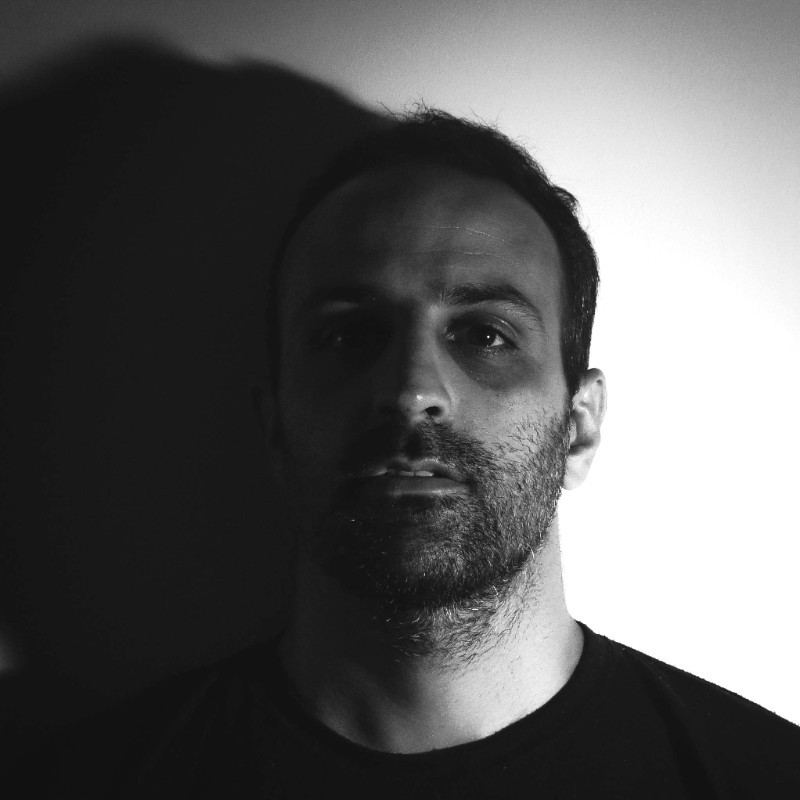
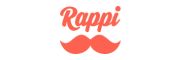