Building RESTful APIs with Laravel: A Complete Tutorial
When it comes to web development, creating a robust, user-friendly, and efficient backend is just as crucial as designing a sleek and intuitive frontend. One of the most effective ways to achieve this is by hiring Laravel developers, who are adept at building such backends using Representational State Transfer (REST) APIs. These APIs allow different software applications to communicate with each other seamlessly.
In this tutorial, we’ll delve into how to build RESTful APIs using Laravel, a popular PHP framework known for its simplicity and elegance, often chosen by skilled Laravel developers.
Introduction to REST APIs
REST APIs work on a request-response model where a client sends a request to a server, which processes the request and sends back an appropriate response. These requests and responses are usually made in JSON format. REST APIs use HTTP methods like GET, POST, PUT, PATCH, and DELETE to perform CRUD (Create, Retrieve, Update, Delete) operations on resources.
Setting Up Laravel
Before you can start building your RESTful API, you’ll need to have Laravel and Composer installed on your machine. If you haven’t done so already, you can follow the installation instructions from Laravel’s official documentation [here](https://laravel.com/docs/8.x/installation).
Step 1: Creating a New Laravel Project
To create a new Laravel project, navigate to your preferred directory in the terminal and run the following command:
composer create-project --prefer-dist laravel/laravel blog
This will create a new Laravel application in a directory named “blog”.
Step 2: Configuring the Database
After setting up the Laravel project, you’ll need to configure your database. Laravel supports a variety of databases like MySQL, SQLite, PostgreSQL, and SQL Server. You can set up your database configuration in the `.env` file located in your project’s root directory.
For example, if you’re using MySQL, your configuration might look like this:
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=laravel DB_USERNAME=root DB_PASSWORD=
Make sure to replace the placeholders with your actual database name, username, and password.
Step 3: Creating a Model and Migration
In Laravel, models are used to interact with the database. For our blog API, we’ll need a Post model. You can create a model and a corresponding migration file using the command:
php artisan make:model Post -m
This will create two files: `app/Models/Post.php` and `database/migrations/xxxx_xx_xx_xxxxxx_create_posts_table.php`.
Next, open the migration file and define the structure of your ‘posts’ table:
```php public function up() { Schema::create('posts', function (Blueprint $table) { $table->id(); $table->string('title'); $table->text('content'); $table->timestamps(); }); }
Then, migrate the table to the database using the command:
php artisan migrate
Step 4: Building the API Routes
Open the `routes/api.php` file and define the routes for your API. Laravel provides resource routing to easily map the CRUD operations:
```php use Illuminate\Support\Facades\Route; use App\Http\Controllers\PostController; Route::resource('posts', PostController::class);
This will create routes for index, create, store, show, edit, update, and delete methods.
Step 5: Creating the Controller
Controllers handle the logic of your application. Use the command below to generate a resource controller:
php artisan make:controller PostController --resource
This will create a controller at `app/Http/Controllers/PostController.php` with methods for each action.
Step 6: Implementing CRUD Operations
Now you can implement the CRUD operations in your `PostController`. Here’s a basic example of how you might implement these operations:
```php use App\Models\Post; public function index() { return Post::all(); } public function store(Request $request) { $post = Post::create($request->all()); return response()->json($post, 201); } public function show(Post $post) { return $post; } public function update(Request $request, Post $post) { $post->update($request->all()); return response()->json($post, 200); } public function destroy(Post $post) { $post->delete(); return response()->json(null, 204); }
Step 7: Testing the API
To test your API, you can use tools like Postman or Insomnia. Send requests to your API’s endpoints (`/api/posts`) and check the responses to ensure that your API is functioning as expected.
Conclusion
“Congratulations! You’ve now built a RESTful API using Laravel. This tutorial provided a basic introduction to the process, paving the way for you to explore more features Laravel offers, such as middleware, validation, and authentication. Of course, if you’re aiming for a more complex project, you might want to hire Laravel developers for their expertise. Keep learning, keep experimenting, and whether you choose to develop further skills or hire Laravel developers, you’re on your way to creating great projects in no time!”
Table of Contents
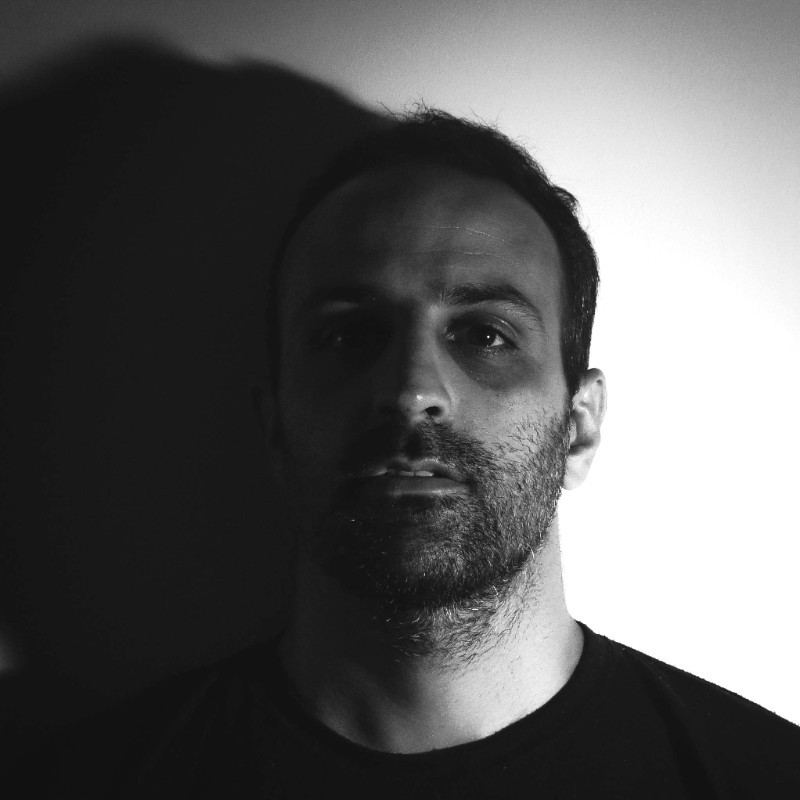
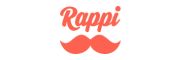