Laravel’s Secret to Effortless Subscription Billing: Introducing Cashier with Stripe
The convenience of subscription-based services has become prevalent in our digital era. When it comes to subscription billing, Stripe is among the front-runners due to its simplicity and scalability. Laravel, a leading PHP framework, offers an expressive and fluent interface to Stripe’s subscription billing services through Laravel Cashier. In this post, we’ll delve deep into how you can easily handle subscription billing with Laravel Cashier for Stripe.
1. What is Laravel Cashier?
Laravel Cashier provides a fluent interface to Stripe’s subscription billing services, allowing developers to handle subscription billing smoothly without getting into the intricacies of Stripe’s API. It handles almost everything for you, from creating subscriptions to managing subscription plans and even generating invoices.
2. Getting Started with Laravel Cashier and Stripe
2.1. Installation
Before diving into the code, ensure you’ve installed Laravel Cashier. You can do this using composer:
```bash composer require laravel/cashier ```
2.2. Configuration
After installation, publish the Cashier configuration and migration files:
```bash php artisan vendor:publish --tag="cashier-migrations" php artisan migrate ```
Next, set your Stripe API keys within the `.env` file:
```bash STRIPE_KEY=your-stripe-key STRIPE_SECRET=your-stripe-secret ```
3. Dive into Subscription Billing
3.1. Creating a Subscription
Creating a subscription is a breeze with Laravel Cashier. First, ensure your user model uses the `Billable` trait:
```php use Laravel\Cashier\Billable; class User extends Authenticatable { use Billable; } ```
Now, you can easily create a subscription:
```php $user = User::find(1); $user->newSubscription('default', 'plan_id_from_stripe')->create($creditCardToken); ```
In the above snippet, ‘default’ is the name of the subscription, and `plan_id_from_stripe` should be replaced with the actual plan ID from your Stripe dashboard.
3.2. Checking Subscription Status
You can check if a user is subscribed to a particular plan:
```php if ($user->subscribed('default')) { // User is subscribed to the default subscription } ```
To determine if a user is still within their trial period:
```php if ($user->onTrial('default')) { // User is on their trial period } ```
3.3. Updating and Cancelling Subscriptions
Changing the subscription plan:
```php $user->subscription('default')->swap('new_plan_id_from_stripe'); ```
Cancelling a subscription:
```php $user->subscription('default')->cancel(); ```
3.4. Handling Invoices
Laravel Cashier simplifies the invoice generation process too. To retrieve a collection of the user’s invoices:
```php $invoices = $user->invoices(); ```
To render a particular invoice as a PDF (you’ll need the dompdf PHP library for this):
```php return $user->downloadInvoice($invoiceId, [ 'vendor' => 'Your Company', 'product' => 'Your Product', ]); ```
4. Handling Webhooks
Stripe uses webhooks to notify your application when an event occurs on your account, like a successful charge or a failed subscription payment. Laravel Cashier comes with a controller that handles these for you.
To make use of this, add the route to `routes/web.php`:
```php Route::cashierWebhooks('stripe/webhook'); ```
Ensure you point Stripe to this webhook URL via the dashboard.
Conclusion
Laravel Cashier provides an intuitive interface to Stripe’s subscription billing services, making it a breeze to implement subscription-based features in your Laravel application. From creating and managing subscriptions to handling invoices and webhooks, Laravel Cashier has got you covered.
While we’ve only scratched the surface in this post, the potential of what you can achieve using Laravel Cashier and Stripe is vast. We recommend diving deeper into the official Laravel Cashier documentation to explore all the functionalities and features it offers.
By seamlessly blending the power of Laravel with the efficiency of Stripe, Laravel Cashier sets the stage for you to create robust, scalable, and efficient subscription-based services for your users. So, what are you waiting for? Dive in, and start building!
Table of Contents
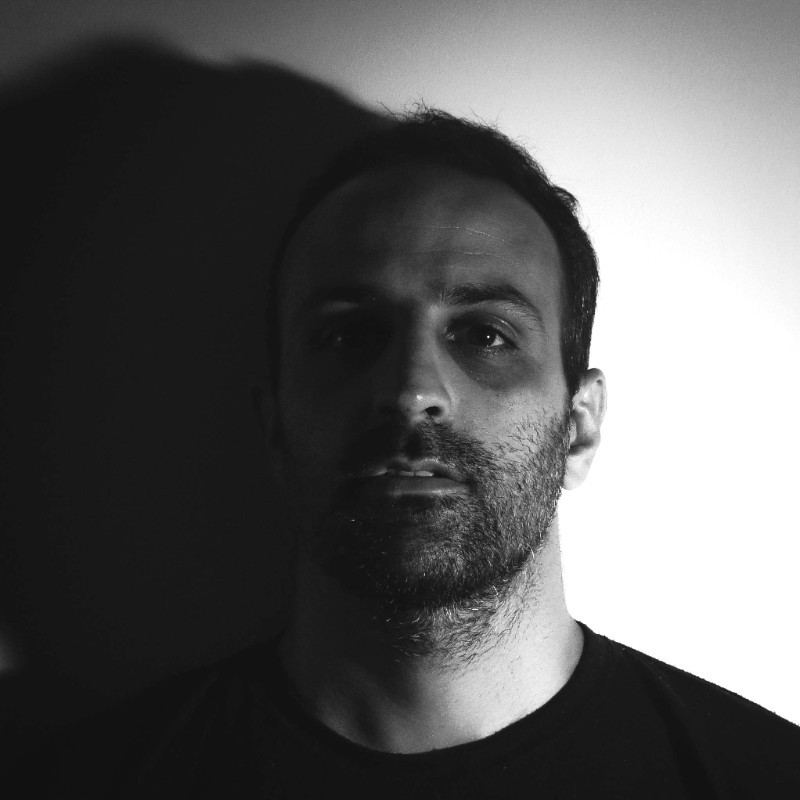
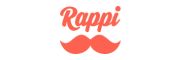