Billing Made Simple: The Power of Laravel Cashier with Braintree
Laravel is a popular PHP framework, loved by developers for its elegance and flexibility. One of the many robust packages offered by the Laravel ecosystem is Laravel Cashier. Designed to handle subscription billing, Cashier simplifies the integration of several payment services. Today, we’ll focus on integrating Cashier with Braintree, a full-stack payment platform known for its user-friendly features.
1. Why Use Laravel Cashier with Braintree?
Laravel Cashier provides an expressive, fluent interface to subscription billing services. It can handle coupons, swapping subscription, subscription “quantities”, cancellation grace periods, and even generate invoice PDFs. Combining this with Braintree, which offers a range of payment options (from credit cards to PayPal), makes for a powerful billing solution.
2. Setting Things Up
2.1. Installation
Start by requiring the Cashier package via Composer:
```bash composer require laravel/cashier-braintree ```
Next, publish the Cashier migrations:
```bash php artisan vendor:publish --tag="cashier-migrations" ```
After the migration has been published, you can create the `cashier_braintree_subscriptions` and `cashier_braintree_plans` tables by running the migrations:
```bash php artisan migrate ```
2.2. Configuration
In your `.env` file, set your Braintree credentials:
``` BRAINTREE_ENV=sandbox BRAINTREE_MERCHANT_ID=your_merchant_id BRAINTREE_PUBLIC_KEY=your_public_key BRAINTREE_PRIVATE_KEY=your_private_key ```
3. Key Concepts
3.1 Creating Subscriptions
Once set up, creating subscriptions becomes straightforward.
Here’s a basic example:
```php $user->newSubscription('main', 'monthly-premium')->create($creditCardToken); ```
In the code above, `’main’` is the name of the subscription, and `’monthly-premium’` would be the id of the plan you’ve created in your Braintree dashboard.
3.2 Checking Subscription Status
To verify if a user is subscribed to a given plan:
```php if ($user->subscribed('main')) { // User is subscribed to "main" plan } ```
3.3 Handling Webhooks
Webhooks allow Braintree to communicate with your application about various events. Cashier can handle these webhook events for you. All you need to do is point a route to Cashier’s controller.
```php Route::post( 'webhook-url', '\Laravel\Cashier\Http\Controllers\WebhookController@handleWebhook' ); ```
Remember to configure the webhook URL in your Braintree dashboard.
4. Advanced Features
4.1 Coupons
Cashier makes it easy to handle discount coupons:
```php $user->newSubscription('main', 'monthly-premium') ->withCoupon('coupon_code') ->create($creditCardToken); ```
4.2 Swapping Subscriptions
Switching users between plans is just as straightforward:
```php $user->subscription('main')->swap('monthly-basic'); ```
4.3 Subscription Quantities
You can easily manage the quantity for subscription plans:
```php $user->subscription('main')->incrementQuantity(); $user->subscription('main')->decrementQuantity(); ```
4.4 Cancellation & Grace Period
Cancelling a subscription but allowing access until the period ends is a graceful touch:
```php $user->subscription('main')->cancel(); ```
To resume a subscription:
```php $user->subscription('main')->resume(); ```
Wrapping Up
Laravel Cashier, combined with Braintree, truly offers a seamless way to manage subscription billing. It abstracts away most of the complexity, allowing you to focus on what really matters – building your application.
Billing and subscription management can be a daunting task, but with tools like Laravel Cashier, it becomes a lot more manageable. It’s a testament to how Laravel continues to offer solutions that make the life of developers easier, allowing us to build robust applications without getting bogged down by the intricacies of subscription billing.
Remember to always check the official documentation for both Laravel Cashier and Braintree, as they provide a wealth of information to help you harness the full potential of this powerful combination. Happy coding!
Table of Contents
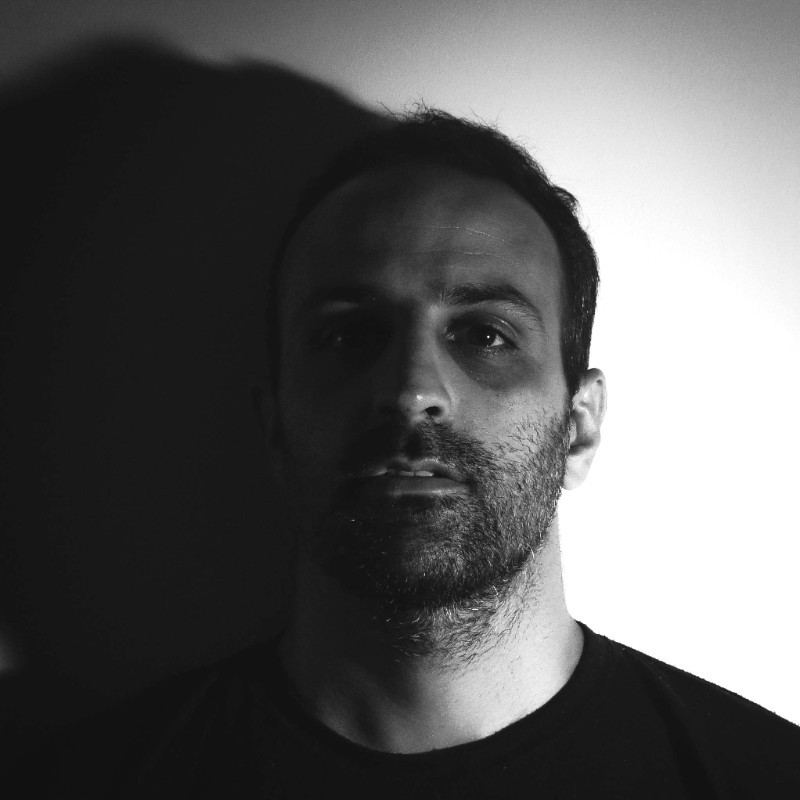
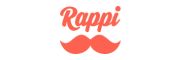