Building a Chat Application with Laravel and WebSockets
In today’s fast-paced digital world, real-time communication is essential for providing a seamless user experience. Whether it’s connecting friends on social media or facilitating business collaboration, chat applications have become an integral part of our lives. In this tutorial, we’ll walk you through the process of building a real-time chat application using Laravel and WebSockets. With Laravel’s powerful features and the efficiency of WebSockets, you can create a robust and responsive chat platform that will delight your users.
Table of Contents
1. Prerequisites
Before we dive into the technical details, let’s make sure you have everything you need to get started:
- Basic understanding of Laravel framework
- Composer installed on your system
- Laravel project set up on your local machine
- Basic knowledge of JavaScript and Vue.js (optional, but helpful)
2. Setting Up Laravel Project
If you already have a Laravel project set up, you can skip this step. If not, follow these steps to create a new Laravel project:
1. Open your terminal and navigate to the directory where you want to create the project.
2. Run the following command to create a new Laravel project:
bash composer create-project --prefer-dist laravel/laravel ChatApp
3. Navigate to the newly created project directory:
bash cd ChatApp
3. Installing Laravel WebSockets Package
Laravel WebSockets is a package that enables real-time communication between clients and servers using WebSockets. It simplifies the process of creating interactive, real-time applications like chat systems. To install the package, follow these steps:
1. Install the package using Composer:
bash composer require beyondcode/laravel-websockets
2. Publish the package’s configuration files:
bash php artisan vendor:publish --provider="BeyondCode\LaravelWebSockets\WebSocketsServiceProvider" --tag="config"
3. Migrate the necessary database tables:
bash php artisan migrate
4. Configuring Laravel WebSockets
To configure Laravel WebSockets for your chat application, follow these steps:
1. Open the config/websockets.php file and make any necessary changes according to your application’s requirements. You can configure settings such as the apps, max_request_size, and path
2. In the config/broadcasting.php file, set the default broadcasting driver to pusher:
php 'default' => env('BROADCAST_DRIVER', 'pusher'),
3. Set your Pusher credentials in the .env file:
env BROADCAST_DRIVER=pusher PUSHER_APP_ID=your-app-id PUSHER_APP_KEY=your-app-key PUSHER_APP_SECRET=your-app-secret PUSHER_APP_CLUSTER=mt1
5. Creating the Chat Interface
Now that we have Laravel WebSockets set up, let’s create the chat interface using Laravel’s powerful Blade templating engine and Vue.js (optional). We’ll create a simple chat room where users can send and receive messages in real-time.
- Create a new Blade view file named chat.blade.php in the resources/views directory.
- Build the basic structure of the chat interface using HTML and CSS. You can style it according to your application’s design.
- Integrate Vue.js to handle real-time updates and user interactions. If you’re new to Vue.js, you can include it via a CDN or set up a build process using Laravel Mix.
6. Implementing Real-Time Chat Functionality
With the chat interface in place, let’s implement the real-time chat functionality using Laravel WebSockets.
1. In your chat.blade.php file, include the necessary JavaScript libraries and create a Vue instance to manage the chat application’s behavior.
html <script src="https://cdn.jsdelivr.net/npm/vue@2.6.14/dist/vue.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/laravel-echo/1.11.4/echo.js"></script> <script src="{{ asset('js/app.js') }}"></script> <!-- Your custom JavaScript file -->
2. Initialize Echo in your JavaScript file (app.js in this case):
javascript import Echo from 'laravel-echo'; window.Echo = new Echo({ broadcaster: 'pusher', key: process.env.MIX_PUSHER_APP_KEY, cluster: process.env.MIX_PUSHER_APP_CLUSTER, encrypted: true, });
3. Set up listeners to receive and display messages in real-time:
javascript new Vue({ el: '#app', data: { messages: [], newMessage: '', }, mounted() { window.Echo.private('chat') .listen('MessageSent', (e) => { this.messages.push({ message: e.message.message, user: e.user, }); }); }, methods: { sendMessage() { if (this.newMessage.trim() === '') return; axios.post('/send', { message: this.newMessage, }); this.newMessage = ''; }, }, });
4. Create a route and controller to handle message sending:
php Route::post('/send', 'ChatController@sendMessage');
In your ChatController:
php public function sendMessage(Request $request) { $user = auth()->user(); event(new MessageSent($user, $request->message)); }
Conclusion
Congratulations! You’ve successfully built a real-time chat application using Laravel and WebSockets. This tutorial covered the essential steps to set up Laravel WebSockets, create the chat interface, and implement real-time functionality using Laravel Echo. From here, you can further enhance the application by adding features like private messaging, message history, and user authentication.
Real-time communication has never been easier, thanks to the powerful combination of Laravel and WebSockets. Whether you’re building a social networking platform, a customer support tool, or a collaborative workspace, the skills you’ve acquired in this tutorial will set you on the path to delivering exceptional real-time experiences for your users.
Table of Contents
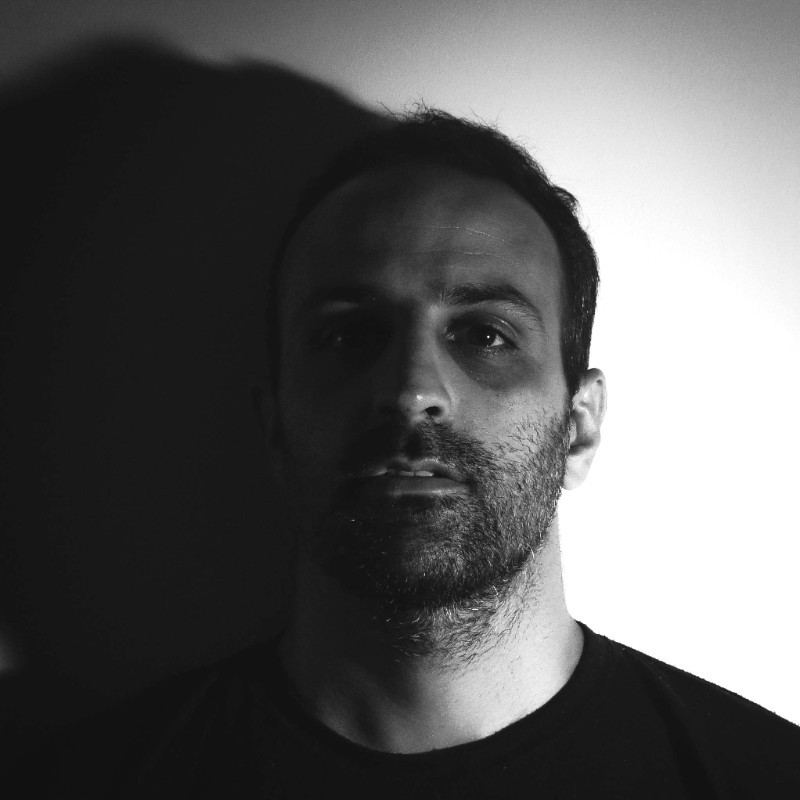
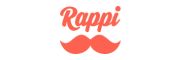