Discover the Secrets of Efficient Data Processing with Laravel Collections
Laravel, a prominent PHP framework, has long been admired for its elegant syntax, ease of use, and comprehensive ecosystem. Among its myriad of features, Laravel Collections stands out as a versatile tool for data manipulation. Collections provide a fluent, intuitive interface to work with arrays of data, making complex operations much simpler and more readable.
In this blog post, we’ll delve deep into Laravel Collections, exploring its power and flexibility through various examples.
1. What are Laravel Collections?
A collection is essentially a wrap around arrays, but it provides a multitude of methods to manipulate data. Think of it as a Swiss Army knife for arrays. With collections, you can filter, sort, modify, and even perform advanced mathematical operations on data with minimal effort.
2. Creating a Collection
A collection can be instantiated in various ways:
```php $collection = collect([1, 2, 3, 4, 5]); ```
You can also create a collection from a range:
```php $collection = collect(range(1, 5)); ```
3. Key Examples of Collection Methods
3.1. Filtering Data
Suppose you have a collection of users and you want to filter out all the users who are older than 30. Here’s how you can do it:
```php $users = collect([ ['name' => 'John', 'age' => 28], ['name' => 'Doe', 'age' => 32], ['name' => 'Smith', 'age' => 24], ]); $usersUnder30 = $users->filter(function ($user) { return $user['age'] <= 30; }); print_r($usersUnder30->values()->all()); ```
3.2. Mapping Over Collections
Let’s say you want to modify each user’s data, perhaps adding a field. The `map` method makes it a breeze:
```php $updatedUsers = $users->map(function ($user) { $user['isAdmin'] = $user['age'] > 30; return $user; }); print_r($updatedUsers->all()); ```
3.3. Reducing Collections
If you want to determine the cumulative age of all users, the `reduce` method is the way to go:
```php $totalAge = $users->reduce(function ($carry, $user) { return $carry + $user['age']; }, 0); echo $totalAge; ```
3.4. Sorting Collections
Sorting becomes extremely easy with collections. For instance, to sort users by age:
```php $sortedUsers = $users->sortBy('age'); print_r($sortedUsers->values()->all()); ```
3.5. Chaining Methods
One of the most powerful features of collections is the ability to chain methods together. Imagine you want to filter users under 30 and then sort them by age:
```php $result = $users->filter(function ($user) { return $user['age'] <= 30; })->sortBy('age'); print_r($result->values()->all()); ```
3.6. Sum, Min, Max, and Avg
Get statistical insights into your collections with ease:
```php $minAge = $users->min('age'); $maxAge = $users->max('age'); $averageAge = $users->avg('age'); $sumAge = $users->sum('age'); ```
4. Using Higher-Order Messages
Laravel Collections also introduces the concept of higher-order messages, which provide shortcuts for some of the most common scenarios:
```php // Instead of using the filter method with a closure, you can do: $usersUnder30 = $users->where('age', '<=', 30); ```
5. Transforming Collections In-Place
While most collection methods return a new collection instance, methods like `transform` modify the collection in place:
```php $users->transform(function ($user) { $user['age'] += 1; // Increment age by 1 return $user; }); print_r($users->all()); ```
Conclusion
Laravel Collections are indeed a powerhouse when it comes to data manipulation. By providing an extensive set of utilities, they drastically reduce the amount of boilerplate code and make your data processing logic much more intuitive.
Whether you’re dealing with arrays of primitive data types or complex multi-dimensional arrays, Laravel Collections offer a robust solution to handle them efficiently. The next time you find yourself wrestling with arrays, remember that Collections can make your life much easier!
Table of Contents
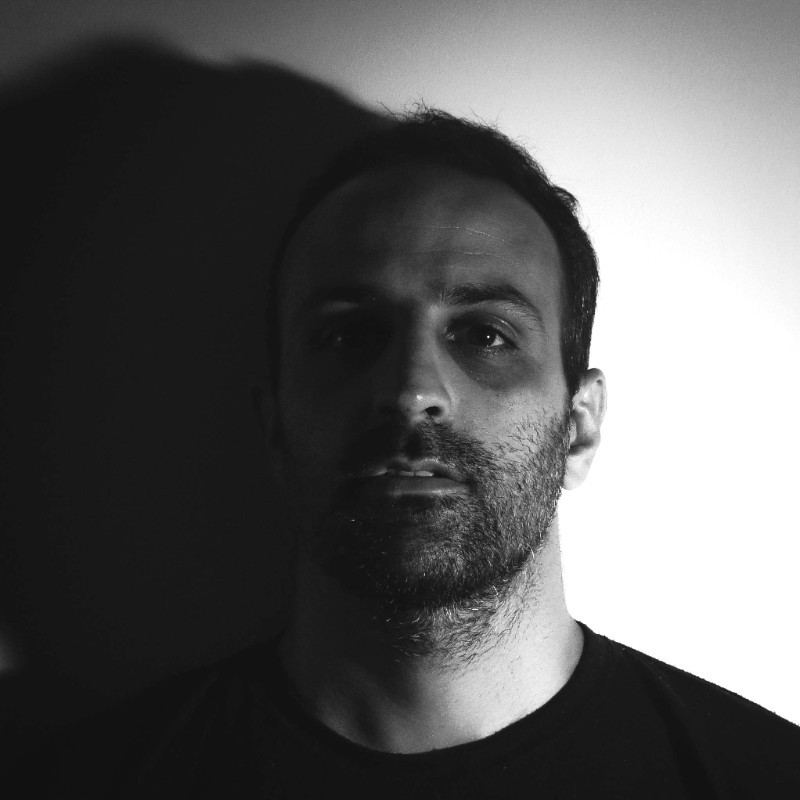
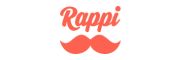