Bolstering Web Development with Laravel’s Artisan Command-Line Interface
As an open-source PHP framework, Laravel has gained widespread popularity among web developers worldwide, thanks to its elegant syntax and extensive features. Laravel offers a built-in command-line interface (CLI) named Artisan, designed to streamline the development process and boost developers’ productivity. This powerful feature set makes it an attractive framework for businesses looking to hire Laravel developers. This blog post explores the practical uses of Artisan, shedding light on why hiring Laravel developers can be a smart choice for your business. We provide concrete examples to illustrate the capabilities of Artisan and how it can streamline your Laravel development projects.
Introduction to Artisan
Artisan is the name of the command-line interface included with Laravel. It provides a large number of helpful commands that can assist you while you build your application. Artisan comes pre-bundled with Laravel and is driven by the powerful Symfony Console component.
For example, to access a list of all the available Artisan commands, you can use the command:
```bash php artisan list ```
The output will display all the commands you can use, including their description and usage.
Key Features of Artisan
Artisan comes equipped with various commands that can help in generating boilerplate code for controllers, migrations, tests, and more. Let’s look at some of the key features:
1. Code Generation:
Artisan provides commands to generate code for controllers, models, migrations, tests, and more. Here’s an example of how to generate a new controller:
```bash php artisan make:controller BlogController ```
This command will create a new controller class at `app/Http/Controllers/BlogController.php`.
2. Database Migrations:
Artisan can manage your database structure by creating migrations. This helps maintain a version control for your database schema, which can be extremely useful when working in a team environment. To create a migration file, you can use the following command:
```bash php artisan make:migration create_blogs_table --create=blogs ```
This command generates a new migration file for a ‘blogs’ table.
3. Task Scheduling:
Artisan also includes a task scheduler, which allows you to schedule tasks by the minute, hour, day, month, or year. You just need to add the tasks to the `schedule()` function within the `App\Console\Kernel` class.
For example:
```php protected function schedule(Schedule $schedule) { $schedule->command('emails:send')->daily(); } ```
This will schedule the `emails:send` Artisan command to run daily.
4. Tinker:
Tinker is a REPL (Read-Eval-Print Loop) that allows you to interact with your Laravel application from the command line. You can perform almost any operation with Tinker that you would typically perform in your Laravel app. To start a Tinker session, simply use:
```bash php artisan tinker ```
Boosting Productivity with Artisan
Let’s delve into some practical examples of how Artisan can help improve your development process:
Creating and Managing Database Structures
Laravel provides an easy way to manage database structures through migrations. Migrations are like version control for your database, allowing a team to easily modify and share the application’s database schema.
For instance, to create a migration for a ‘users’ table:
```bash php artisan make:migration create_users_table --create=users ```
You can then define the table structure in the generated migration file:
```php public function up() { Schema::create('users', function (Blueprint $table) { $table->id(); $table->string('name'); $table->string('email')->unique(); $table->timestamps(); }); } ```
To run the migrations, use:
```bash php artisan migrate ```
This will create the ‘users’ table with the defined columns.
Automating Tasks
Artisan’s task scheduling feature enables you to automate various tasks. Suppose you want to send out daily email reminders to your users. First, you’d need to create a command:
```bash php artisan make:command SendEmails ```
This command generates a new command class at `app/Console/Commands/SendEmails.php`. In this file, you’d need to implement the `handle()` method, where you define the logic to send emails.
```php public function handle() { // Logic to send emails } ```
To schedule this command to run daily, you add it to the `schedule()` function within the `App\Console\Kernel` class:
```php protected function schedule(Schedule $schedule) { $schedule->command('emails:send')->daily(); } ```
Interactive Database Manipulation
Laravel Tinker provides an interactive shell to manipulate your application’s data. It’s highly useful for debugging, prototyping and exploring Laravel’s features.
For example, to create a new user, you’d start a Tinker session:
```bash php artisan tinker ```
And then, in the Tinker shell, you can create a new user:
```bash $user = new \App\Models\User; $user->name = 'John Doe'; $user->email = 'johndoe@example.com'; $user->save(); ```
Conclusion
Artisan, Laravel’s built-in CLI, is an incredibly powerful tool that significantly boosts productivity, making it an essential part of the toolkit for Laravel developers. Its features, such as code generation, database migrations, task scheduling, and an interactive shell (Tinker), speed up the development process and allow developers to focus on the more critical aspects of a project. This is why businesses looking to build robust applications often choose to hire Laravel developers. Whether you’re a seasoned Laravel developer or a beginner, or even a business looking to hire Laravel developers, being well-acquainted with Artisan is vital to harness the full power of the Laravel framework.
Table of Contents
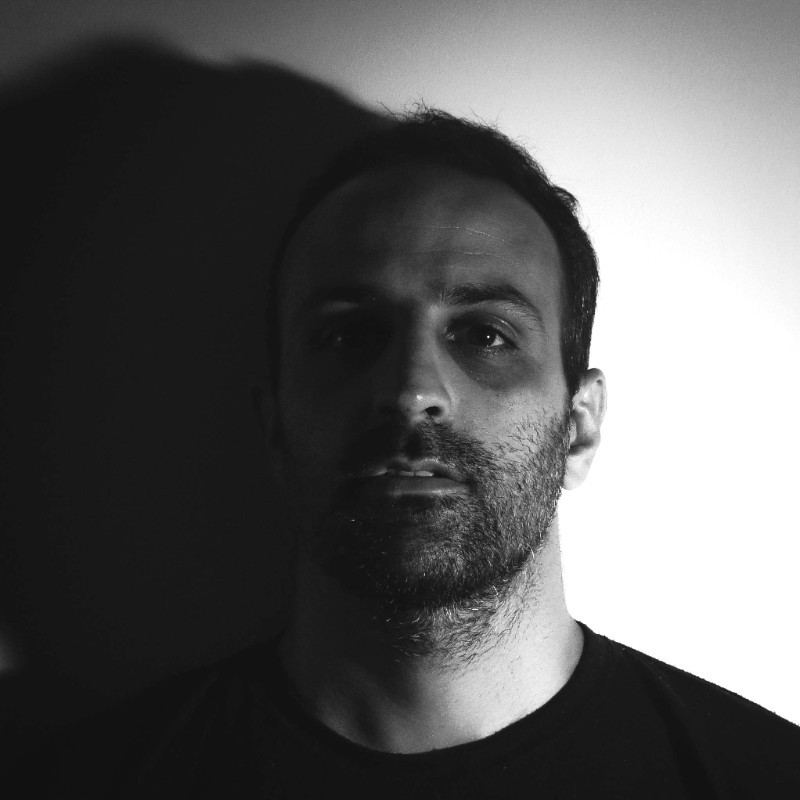
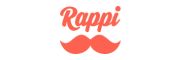