Simplify Your Workflow: Explore Laravel Mix for Easy Asset Compilation
Laravel Mix is an elegant wrapper for webpack, a powerful module bundler, allowing developers, particularly those you might hire as Laravel developers, to compile and optimize various web assets with ease. Mix provides a clean, API-based approach to defining webpack build steps for your Laravel application. This feature makes it even more appealing to hire Laravel developers who can effectively utilize this tool to streamline your project’s workflow.
Table of Contents
1. What is Laravel Mix?
Before we delve into examples, let’s understand what Laravel Mix is. Laravel Mix, created by Jeffrey Way for Laravel, provides a fluent API for defining webpack build steps for your Laravel applications using several common CSS and JavaScript preprocessors. You can think of it as a streamlined interface for webpack, offering a simple configuration process while also allowing you to leverage webpack’s raw power when needed.
Through Laravel Mix, you can compile CSS, JavaScript, and even images and fonts. It’s versatile, handling popular CSS preprocessors like Less and Sass and supporting modern JavaScript transpiling using Babel.
2. Setting Up Laravel Mix
If you are using Laravel, Laravel Mix is included out of the box. But if you want to use Laravel Mix in a non-Laravel project, you can do so. Here’s how:
- First, you need to install Node.js and NPM (Node Package Manager). You can download them from the official Node.js website.
- In your project root, run the following command to create a `package.json` file:
```bash npm init -y ```
- Now install Laravel Mix:
```bash npm install laravel-mix --save-dev ```
- Once installed, you can copy the example Mix file to your project root:
```bash cp node_modules/laravel-mix/setup/webpack.mix.js ./ ```
You now have a `webpack.mix.js` file in your project root. This is where you’ll define your asset compilation.
3. Basic Usage
The `webpack.mix.js` file is where you will define all your webpack build steps. Here’s an example of a simple Laravel Mix setup:
```javascript const mix = require('laravel-mix'); mix.js('resources/js/app.js', 'public/js') .sass('resources/sass/app.scss', 'public/css'); ```
In the example above, we’ve asked Mix to compile the `app.js` file and the `app.scss` Sass file and output them to the `public/js` and `public/css` directories, respectively.
To compile the assets, run:
```bash npm run dev ```
If you want to watch the files for changes and compile them automatically, you can use:
```bash npm run watch ```
4. Processing Stylesheets
Laravel Mix provides support for CSS preprocessors like Less, Sass, and Stylus, along with post-processing of CSS via PostCSS.
```javascript // Using Less mix.less('resources/less/app.less', 'public/css'); // Using Sass mix.sass('resources/sass/app.scss', 'public/css'); // Using Stylus mix.stylus('resources/stylus/app.styl', 'public/css'); // Using PostCSS mix.postCss('resources/css/app.css', 'public/css', [ require('postcss-custom-properties') ]); ```
5. Compiling JavaScript
Laravel Mix uses Babel for JavaScript compilation, supporting ES2015, ES2017, and JSX syntax out of the box. Here’s how you can compile your JavaScript files:
```javascript mix.js('resources/js/app.js', 'public/js'); ```
You can also compile multiple JavaScript files into a single output file:
```javascript mix.js(['resources/js/app.js', 'resources/js/dashboard.js'], 'public/js/all.js'); ```
6. Versioning / Cache Busting
Browser caching can often lead to old versions of your CSS or JavaScript files being used, even after updates have been made. Laravel Mix offers an easy way to version your files to help avoid this:
```javascript mix.js('resources/js/app.js', 'public/js') .sass('resources/sass/app.scss', 'public/css') .version(); ```
With the `version()` method, Mix will append a unique hash to the filenames of compiled files, like `app.js?id=8e5c48a33b50f8e160f7`.
7. Copying Files & Directories
You might need to move some files or directories during compilation. Laravel Mix’s `copy()` function makes this easy:
```javascript mix.copy('resources/img', 'public/img'); ```
This command copies everything from `resources/img` to `public/img`.
8. BrowserSync
If you’re making changes frequently and want to see those changes in real-time without manually refreshing your browser, Laravel Mix’s integration with BrowserSync can be a game-changer:
```javascript mix.browserSync('your-domain.test'); ```
Replace `’your-domain.test’` with your local development URL. Now, whenever you modify a script or PHP file, watch as the browser automatically refreshes the page!
Wrapping Up
Laravel Mix takes the pain out of asset compiling and optimization, making it a powerful tool for developers and a primary reason to hire Laravel developers. The examples provided here are basic, yet Laravel Mix’s capabilities extend much further. It enables handling tasks like code splitting, hot module replacement, and much more.
For detailed information and advanced usage, I recommend visiting the [official Laravel Mix documentation] (https://laravel-mix.com/docs).
By combining the flexibility of webpack with an intuitive, simplified API, Laravel Mix indeed lets you compile assets with ease. It’s one of the key advantages that Laravel developers bring to your project. Whether you’re building a small application or a complex system, it’s an invaluable tool that will streamline your workflow, improve productivity, and enhance the overall development experience. Hiring Laravel developers will ensure you leverage these benefits effectively. Happy coding!
Table of Contents
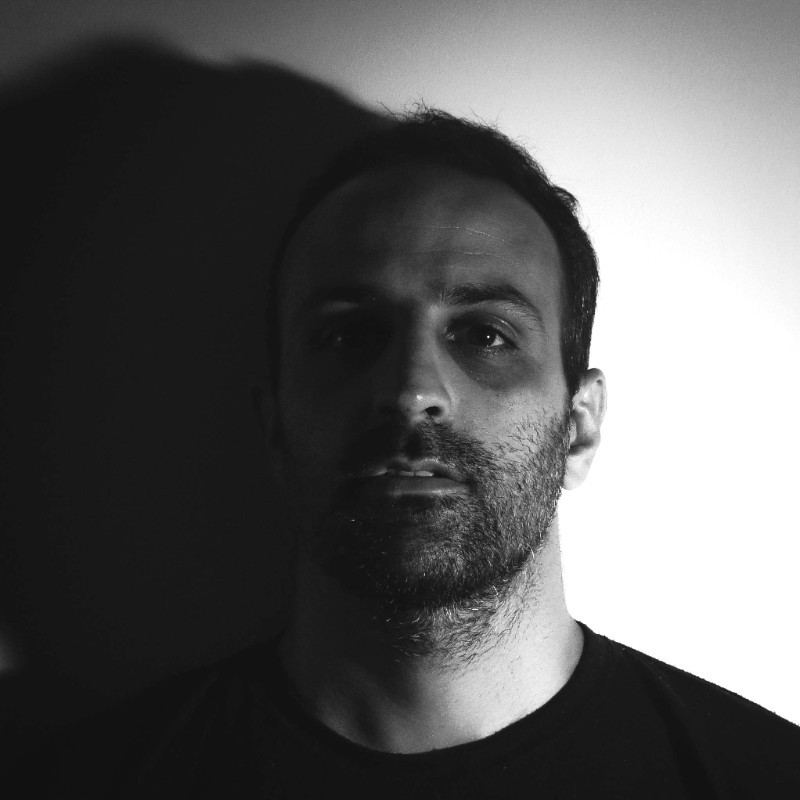
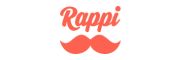