A Beginner’s Guide to Building a Blog Content Management System with Laravel
Laravel, a prominent open-source PHP framework, is renowned for its simplicity, elegance, and readability. With its rich ecosystem and expressive syntax, it stands as an excellent choice for building complex applications, like a Content Management System (CMS). To leverage this potential fully, many choose to hire Laravel developers who can expedite and enhance the development process.
In this article, we will walk you through creating a basic blog CMS using Laravel. Whether you’re a seasoned developer or you’ve opted to hire Laravel developers for your project, this guide will serve as a useful roadmap. By the end, you will have a system that allows you to create, read, update, and delete (CRUD) blog posts with ease and efficiency.
Prerequisites
To follow this tutorial, you’ll need:
– Basic understanding of PHP
– Laravel and Composer installed on your machine
– Basic knowledge of Laravel’s MVC (Model-View-Controller) architecture
Step 1: Setting Up the Laravel Project
First, we’ll create a new Laravel project using the following command in the terminal:
“`
composer create-project –prefer-dist laravel/laravel blog
“`
Next, navigate into the newly created directory:
“`
cd blog
“`
Step 2: Setting Up Database
Now, we need to set up a database. Laravel supports many databases, but for simplicity, we’ll use MySQL in this tutorial. In your `.env` file, set up your database configuration:
``` DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=blog DB_USERNAME=root DB_PASSWORD= ```
After setting up the database, run the following migration command:
``` php artisan migrate ```
Step 3: Creating the Blog Model
A model represents a table in your database. Create a `Blog` model by running the command:
``` php artisan make:model Blog -m ```
The `-m` option creates a migration file for the `Blog` model. Open this migration file, and within the `up` method, specify the fields you want for your blog posts:
```php public function up() { Schema::create('blogs', function (Blueprint $table) { $table->id(); $table->string('title'); $table->text('body'); $table->timestamps(); }); } ```
After setting up the fields, run the migrations:
``` php artisan migrate ```
Step 4: Building the Controller
The controller handles the logic of the application. Create a `BlogController`:
``` php artisan make:controller BlogController --resource ```
The `–resource` option generates a controller with methods for each CRUD operation.
Open the `BlogController`, and you’ll see methods like `index()`, `create()`, `store()`, `edit()`, `update()`, and `destroy()`.
For example, to store a blog post, your `store()` method may look like this:
```php public function store(Request $request) { $blog = new Blog; $blog->title = $request->title; $blog->body = $request->body; $blog->save(); return redirect('/blogs')->with('success', 'Blog Created Successfully'); } ```
Step 5: Building the Views
Views are responsible for displaying the application’s UI. For our blog, we’ll need views to list all posts, display a single post, create a post, and edit a post.
To create a view, make a Blade file in the `resources/views` directory. Laravel’s Blade templating engine provides an elegant syntax for writing HTML and PHP.
For instance, the view to display a form to create a new post (`create.blade.php`) may look like:
```html @extends('layouts.app') @section('content') <div class="container"> <form action="/blogs" method="POST"> @csrf <div class="form-group"> <label for="title">Title</label> <input type="text" class="form-control" id="title" name="title" required> </div> <div class="form-group"> <label for="body">Body</label> <textarea class="form-control" id="body" name="body" rows="3" required></textarea> </div> <button type="submit" class="btn btn-primary">Submit</button> </form> </div> @endsection ```
Step 6: Setting Up Routes
Lastly, you need to define the routes to your application’s actions. In the `routes/web.php` file, you can define routes like this:
```php Route::resource('blogs', 'BlogController'); ```
This single line of code will automatically generate all the routes needed for the CRUD operations in your `BlogController`.
Now, you can start your Laravel development server:
``` php artisan serve ```
And visit `http://127.0.0.1:8000/blogs` to see your blog in action.
Conclusion
Building a blog CMS with Laravel is straightforward, thanks to its expressive syntax, MVC architecture, and robust ecosystem. By following these steps, you have created a blog where you can create, read, update, and delete posts.
Remember, this is a basic implementation. To enhance the functionality of your blog CMS, consider hiring Laravel developers who can add advanced features like authentication, categories, tags, comments, image uploading, and much more. With Laravel’s extensive documentation and rich package ecosystem, these developers will have the necessary tools to build complex applications tailored to your needs. Embrace the possibilities of Laravel and enjoy the journey of coding!
To hire pre-vetted Laravel developers from CloudDevs, get in touch with our consultants today.
Table of Contents
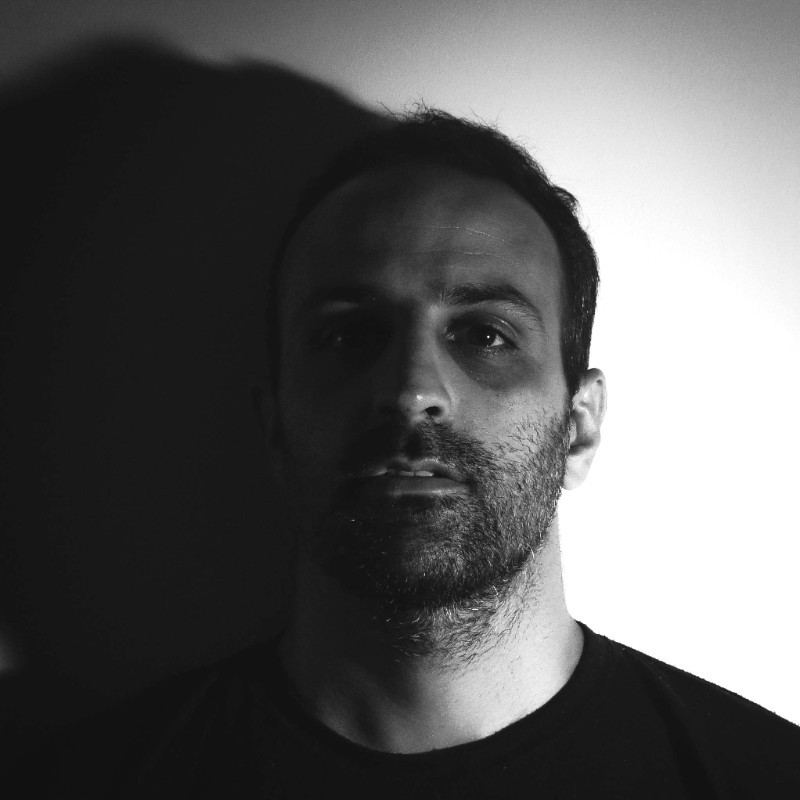
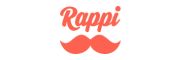