How to create a controller in Laravel?
Creating a controller in Laravel is like crafting a guidebook for your web application, defining how it responds to different user requests and interactions. Controllers serve as the bridge between your application’s routes and the logic that handles those routes, making it easier to organize and manage your application’s functionality. Here’s a user-friendly guide on how to create a controller in Laravel:
To begin, open your terminal or command prompt and navigate to the root directory of your Laravel project. Laravel’s Artisan command-line interface makes it simple to generate controllers with just a few keystrokes.
Once you’re in your project directory, use the following Artisan command to create a new controller:
go php artisan make:controller UserController
In this command, make:controller is the Artisan command used to generate a new controller, and UserController is the name you want to give to your controller. You can replace UserController with any name you prefer for your controller.
After running the command, Laravel will generate a new controller file named UserController.php in the app/Http/Controllers directory of your project. This file contains a basic skeleton for your controller class, including a namespace declaration and an empty class definition.
Inside the UserController class, you can define methods to handle various HTTP requests, such as index to display a list of users, show to view a specific user, store to create a new user, update to update an existing user, and destroy to delete a user.
For example, here’s how you might define an index method in your UserController to display a list of users:
php class UserController extends Controller { public function index() { $users = User::all(); return view('users.index', ['users' => $users]); } }
In this method, we’re using Laravel’s Eloquent ORM to retrieve all user records from the database and pass them to a view called users.index. This view will be responsible for displaying the list of users to the user interface.
You’ve successfully created a controller in Laravel. You can now define additional methods and logic within your controller to handle different routes and user interactions in your application. Laravel’s MVC (Model-View-Controller) architecture makes it easy to organize and manage your application’s functionality, allowing you to focus on building great user experiences.
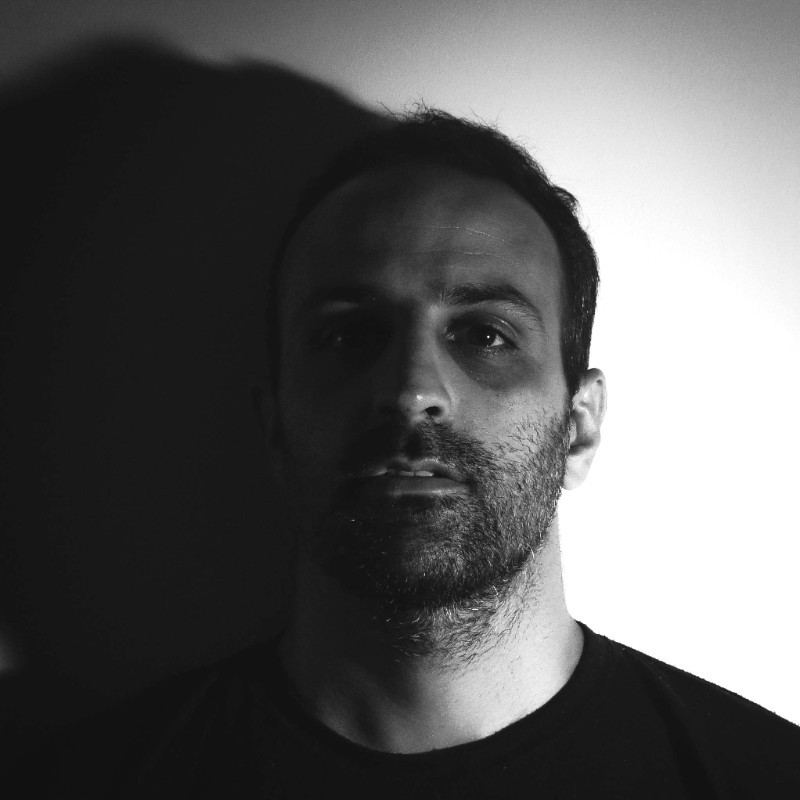
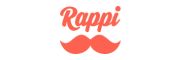