How to create custom Artisan commands in Laravel?
Creating custom Artisan commands in Laravel is like adding your own personalized tools to your development toolkit—it allows you to automate tasks, streamline workflows, and extend the functionality of your Laravel applications. Here’s how you can create custom Artisan commands in Laravel in a user-friendly way:
Create a New Command Class: Start by creating a new PHP class that extends Laravel’s Illuminate\Console\Command base class. This class will serve as the blueprint for your custom Artisan command. You can generate a new command class using the make:command Artisan command:
bash php artisan make:command YourCommandName
Define Command Properties and Methods: Inside your custom command class, define the command name, description, signature, and any command options or arguments that your command requires. You can override the signature, description, handle, and other methods to define the behavior of your custom command.
Implement Command Logic: Implement the logic for your custom command inside the handle method of your command class. This method will be executed when the command is invoked via the Artisan CLI. You can perform any tasks or operations inside this method, such as interacting with the database, making API calls, processing files, or running other Artisan commands.
Register Command with Laravel: Once you’ve defined your custom command class, you need to register it with Laravel’s Artisan command registrar. You can do this by adding your command class to the $commands array in the app/Console/Kernel.php file:
php protected $commands = [ Commands\YourCommandName::class, ];
Use Your Custom Command: With your custom command registered, you can now use it via the Artisan CLI just like any other built-in Artisan command. Simply run the command by its name:
php artisan your:command-name
Pass Command Arguments and Options: If your custom command requires arguments or options, you can pass them when invoking the command via the Artisan CLI. Arguments and options are defined in the signature property of your command class and can be accessed within the handle method using Laravel’s command input helpers.
Test and Iterate: Finally, test your custom command to ensure that it behaves as expected and performs the intended tasks. Iterate on your command logic as needed, and consider adding error handling and validation to make your command more robust and user-friendly.
By following these steps, you can create custom Artisan commands in Laravel to automate tasks, simplify workflows, and extend the functionality of your Laravel applications. Custom Artisan commands provide a convenient way to encapsulate common tasks and operations, making your development process more efficient and enjoyable.
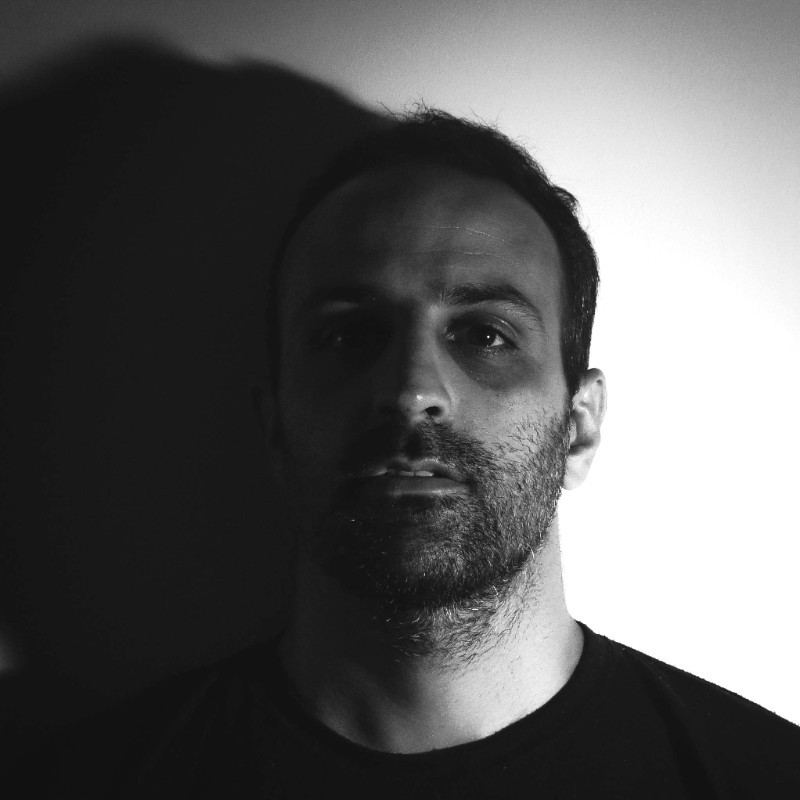
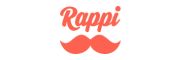