How to create custom middleware in Laravel?
Creating custom middleware in Laravel is like crafting a unique set of rules or filters that your web application follows before serving content to users—it’s a powerful way to add custom functionality and processing logic to your application’s request lifecycle. Let’s explore how you can create custom middleware in Laravel in a human-friendly way:
To begin, open your Laravel project in your preferred code editor. Laravel’s middleware system allows you to define custom middleware classes that encapsulate the logic you want to apply to incoming HTTP requests.
To create a new custom middleware class, you’ll first need to generate it using Laravel’s Artisan command-line interface. In your terminal or command prompt, navigate to your project directory and run the following Artisan command:
go php artisan make:middleware MyCustomMiddleware
Replace MyCustomMiddleware with the name you want to give to your custom middleware class. This command will generate a new PHP class file in the app/Http/Middleware directory of your Laravel project.
Next, open the newly created middleware class file in your code editor. Inside the class, you’ll find a handle method where you can define the logic that should be executed for each incoming request.
The handle method receives two parameters: $request, which represents the incoming HTTP request, and $next, which is a closure that represents the next middleware or the controller logic that should be executed after your middleware.
Here’s a simple example of how you might define a custom middleware that logs information about each incoming request:
php namespace App\Http\Middleware; use Closure; use Illuminate\Support\Facades\Log; class MyCustomMiddleware { public function handle($request, Closure $next) { // Log information about the incoming request Log::info('Incoming request: ' . $request->fullUrl()); // Continue to the next middleware or controller logic return $next($request); } }
In this example, we’re using Laravel’s logging feature to log information about each incoming request, such as the full URL. After logging the information, we’re allowing the request to continue to the next middleware or controller logic by calling the $next closure with the $request parameter.
Once you’ve defined your custom middleware class, you can apply it to routes or groups of routes in your application’s route definitions using Laravel’s expressive syntax. This allows you to selectively apply your custom middleware to specific parts of your application where it’s needed most.
By creating custom middleware in Laravel, you can add powerful functionality and processing logic to your application’s request lifecycle, enhancing its capabilities and providing a richer user experience. Whether you’re implementing logging, authentication, authorization, or any other custom logic, middleware gives you the flexibility and control you need to build robust and feature-rich web applications.
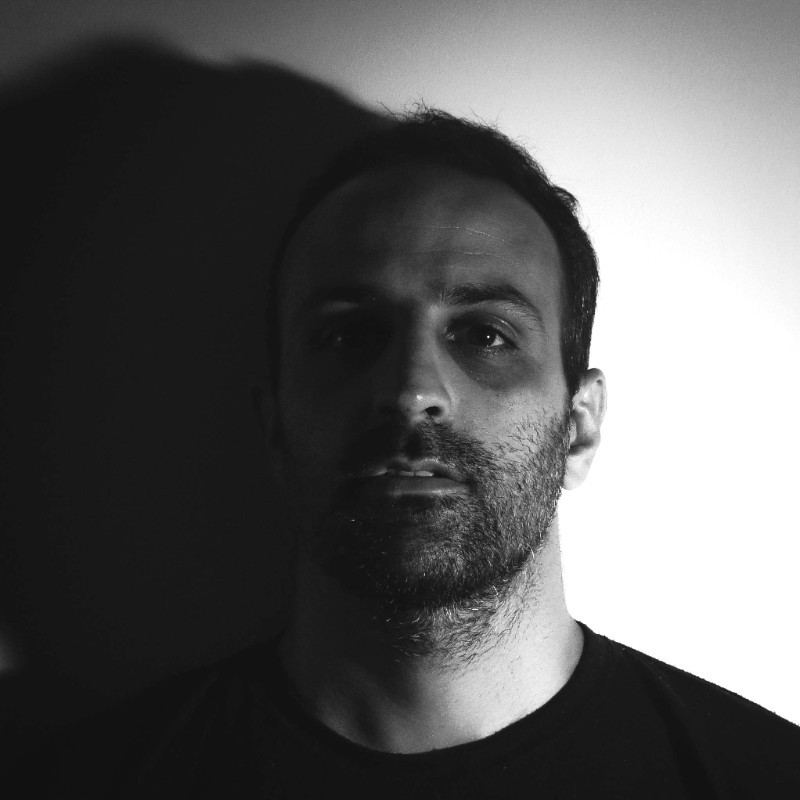
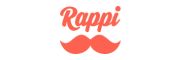